How to allow comma seperated values for search parameter in LightSwitch
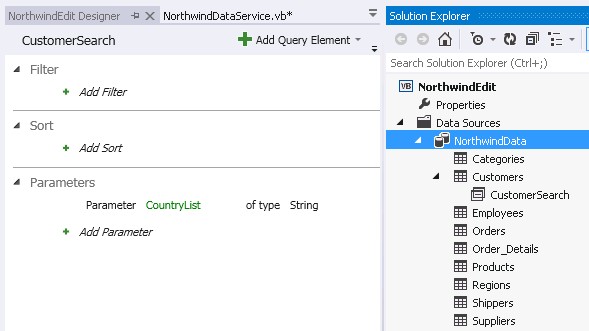
Recently someone asked me how to pass multiple values as search parameter in LightSwitch ? Well I tried to do some research and looks like there is no in-built control to select multiple items from Filter Dropdown. But after doing some research I found an easy way to accomplish similar solution which is not exactly the way I wanted but it works for me.. and hope it works for you too.
So trick is use TextBox for Search parameter where user can enter multiple comma separated values (e.g. P112,P111,P118)
There are 3 Basic Steps we have to do so we can achieve desired behavior
- Create custom query for datasource where you have to define parameter (e.g. CustomerIDList)
- Write code to change default Linq query in MyQuery_PreprocessQuery event handler
- Create search screen and select Custom Query as data source
Create custom query and define parameter
This post assumes you already have defined LightSwitch Datasource. If you already have datasource then you can right click on any datatable and select create new query. Name your new query (e.g. CustomerSearch for this example)
On query screen define parameter as below (Don’t bind with search filter).
Write code in MyQuery_PreprocessQuery event handler
Double click on custom query to open Parameter screen. On this screen you will see “Write Code” button on you top right corner (see below screen). If you pull up that dropdown you will see various events you can handle for this custom query. Select PreprocessQuery Event.
Now what we need to do is modify default Linq query as below so it only selects country found from user defined comma separated list.
Namespace LightSwitchApplication Public Class NorthwindDataService Private Sub CustomerSearch_PreprocessQuery(CountryList As String, ByRef query As System.Linq.IQueryable(Of LightSwitchApplication.Customer)) If String.IsNullOrWhiteSpace(CountryList) Then CountryList = String.Empty End If Dim myInClause() As String = CountryList.Split(",") For i = 0 To myInClause.Length - 1 myInClause(i) = myInClause(i).Trim() Next query = From cust In query Where myInClause.Contains(cust.Country) Select cust End Sub End Class End Namespace
Example-2: Multiple parameters
Here is another example of query with multiple parameters
Private Sub OrdersSearchQuery1_PreprocessQuery(pCustomerList As String, pStartDate As System.Nullable(Of Date), pEndDate As System.Nullable(Of Date), pEmployeeID As System.Nullable(Of Integer), ByRef query As System.Linq.IQueryable(Of LightSwitchApplication.Order) _ ) If String.IsNullOrWhiteSpace(pCustomerList) Then pCustomerList = String.Empty End If Dim myInClause() As String = pCustomerList.Split(",") For i = 0 To myInClause.Length - 1 myInClause(i) = myInClause(i).Trim() Next query = From o In query Where (myInClause.Contains(o.Customer1.CustomerID) _ OrElse pCustomerList = String.Empty) _ AndAlso (o.OrderDate >= pStartDate AndAlso o.OrderDate <= pEndDate) _ AndAlso (o.Employee.EmployeeID = pEmployeeID) Select o End Sub
Create search screen and use CustomQuery as data source
Once we have custom query defined we can use it to bind with search screen.
Create new search screen and select CustomQuery we created as datasource. Below screenshot shows new screen designtime properties.
Run application and test filter
Now execute application and try to search by putting some comma separated values and see your filter works.
Leave a Reply
You must be logged in to post a comment.