Filled shapes are geometric forms that are outlined by using the current pen and filled by using the current brush. There are five filled shapes:
Applications use filled shapes for a variety of tasks. Spreadsheet applications, for example, use filled shapes to construct charts and graphs, and drawing and painting applications use filled shapes to allow the user to draw figures and illustrations. Let's understand each shape in detail
Ellipse
An ellipse is a closed curve defined by two fixed points (f1 and f2) such that the sum of the distances (d1 + d2) from any point on the curve to the two fixed points is constant. The following illustration shows an ellipse drawn by using the Ellipse function.
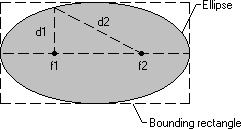
When calling Ellipse, an application supplies the coordinates of the upper-left and lower-right corners of the ellipse's bounding rectangle. A bounding rectangle is the smallest rectangle completely surrounding the ellipse. When the system draws the ellipse, it excludes the right and lower sides if no world transformations are set. Therefore, for any rectangle measuring x units wide by y units high, the associated ellipse measures x1 units wide by y1 units high. If the application sets a world transformation by calling the SetWorldTransform or ModifyWorldTransform function, the system includes the right and lower sides.
Chord A chord is a region bounded by the intersection of an ellipse and a line segment called a secant. The following illustration shows a chord drawn by using the Chord function.
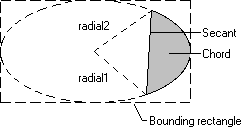
When calling Chord, an application supplies the coordinates of the upper-left and lower-right corners of the ellipse's bounding rectangle, as well as the coordinates of two points defining two radials. A radial is a line drawn from the center of an ellipse's bounding rectangle to a point on the ellipse.
When the system draws the curved part of the chord, it does so by using the current arc direction for the specified device context. The default arc direction is counterclockwise. You can have your application reset the arc direction by calling the SetArcDirection function.
Pie
A pie is a region bounded by the intersection of an ellipse curve and two radials. The following illustration shows a pie drawn by using the Pie function.
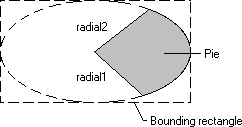
When calling Pie, an application supplies the coordinates of the upper-left and lower-right corners of the ellipse's bounding rectangle, as well as the coordinates of two points defining two radials.
When the system draws the curved part of the pie, it uses the current arc direction for the given device context. The default arc direction is counterclockwise. An application can reset the arc direction by calling the SetArcDirection function.
Polygon
A polygon is a filled shape with straight sides. The sides of a polygon are drawn by using the current pen. When the system fills a polygon, it uses the current brush and the current polygon fill mode. The two fill modesalternate (the default) and windingdetermine whether regions within a complex polygon are filled or left unpainted. An application can select either mode by calling the SetPolyFillMode function. For more information about polygon fill modes, see Regions.
The following illustration shows a polygon drawn by using Polygon.
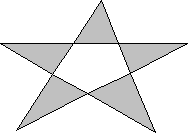
In addition to drawing a single polygon by using Polygon, an application can draw multiple polygons by using the PolyPolygon function.
Rectangle
Rectangles are used for the cursor clipping region, the invalid portion of the client area, an area for displaying formatted text, or the scroll area. Your applications can also use rectangles to fill, frame, or invert a portion of the client area with a given brush, and to retrieve the coordinates of a window or a window's client area.
Now let's implement working example
Step-By-Step Example
- Create a standard exe project - Add one picturebox and one command button control on the form1 - Add the following code in form1 |
Click here to copy the following block |
Private Type POINTAPI x As Long y As Long End Type
Private Type RECT left As Long top As Long right As Long bottom As Long End Type
Private Declare Function SelectObject Lib "gdi32" ( _ ByVal hdc As Long, ByVal hObject As Long) As Long
Private Declare Function DeleteObject Lib "gdi32" ( _ ByVal hObject As Long) As Long
Private Declare Function GetWindowRect Lib "user32" ( _ ByVal hWnd As Long, lpRect As RECT) As Long
Private Declare Function SetRect Lib "user32.dll" ( _ lpRect As RECT, ByVal X1 As Long, ByVal Y1 As Long, _ ByVal X2 As Long, ByVal Y2 As Long) As Long
Private Declare Function CreateHatchBrush Lib "gdi32" ( _ ByVal nIndex As Long, ByVal crColor As Long) As Long
Private Declare Function Chord Lib "gdi32" ( _ ByVal hdc As Long, ByVal X1 As Long, ByVal Y1 As Long, _ ByVal X2 As Long, ByVal Y2 As Long, _ ByVal X3 As Long, ByVal Y3 As Long, _ ByVal X4 As Long, ByVal Y4 As Long) As Long
Private Declare Function Ellipse Lib "gdi32" ( _ ByVal hdc As Long, ByVal X1 As Long, ByVal Y1 As Long, _ ByVal X2 As Long, ByVal Y2 As Long) As Long
Private Declare Function FillRect Lib "user32" ( _ ByVal hdc As Long, lpRect As RECT, ByVal hBrush As Long) As Long
Private Declare Function FloodFill Lib "gdi32" ( _ ByVal hdc As Long, ByVal x As Long, ByVal y As Long, ByVal crColor As Long) As Long
Private Declare Function ExtFloodFill Lib "gdi32" ( _ ByVal hdc As Long, ByVal x As Long, ByVal y As Long, _ ByVal crColor As Long, ByVal wFillType As Long) As Long
Private Declare Function FrameRect Lib "user32" ( _ ByVal hdc As Long, lpRect As RECT, ByVal hBrush As Long) As Long
Private Declare Function InvertRect Lib "user32" ( _ ByVal hdc As Long, lpRect As RECT) As Long
Private Declare Function Pie Lib "gdi32" ( _ ByVal hdc As Long, ByVal X1 As Long, ByVal Y1 As Long, _ ByVal X2 As Long, ByVal Y2 As Long, _ ByVal X3 As Long, ByVal Y3 As Long, _ ByVal X4 As Long, ByVal Y4 As Long) As Long
Private Declare Function Polygon Lib "gdi32" ( _ ByVal hdc As Long, lpPoint As POINTAPI, ByVal nCount As Long) As Long
Private Declare Function PolyPolygon Lib "gdi32" ( _ ByVal hdc As Long, lpPoint As POINTAPI, lpPolyCounts As Long, _ ByVal nCount As Long) As Long
Private Declare Function Rectangle Lib "gdi32" ( _ ByVal hdc As Long, ByVal X1 As Long, ByVal Y1 As Long, _ ByVal X2 As Long, ByVal Y2 As Long) As Long
Private Declare Function RoundRect Lib "gdi32" ( _ ByVal hdc As Long, ByVal X1 As Long, ByVal Y1 As Long, _ ByVal X2 As Long, ByVal Y2 As Long, _ ByVal X3 As Long, ByVal Y3 As Long) As Long
Const FLOODFILLBORDER = 0 Const FLOODFILLSURFACE = 1 Const crNewColor = &HFFFF80
Const HS_DIAGCROSS = 5
Dim Ret As Long
Dim WndRect As RECT, TmpRect As RECT
Private Sub Command1_Click() If Timer1.Enabled = True Then Timer1.Enabled = False Command1.Caption = "Start Demo" Else Timer1.Enabled = True Command1.Caption = "Stop Demo" End If End Sub
Private Sub Form_Load() Command1.Caption = "Start Demo" Timer1.Enabled = False Timer1.Interval = 500
Retval = GetWindowRect(Picture1.hWnd, WndRect) Retval = SetRect(WndRect, 0, 0, WndRect.right - WndRect.left, WndRect.bottom - WndRect.top)
Picture1.AutoRedraw = True End Sub
Private Sub Form_QueryUnload(Cancel As Integer, UnloadMode As Integer) If h <> 0 Then DeleteObject h End Sub
Private Sub Timer1_Timer() Static NextOp As Long Dim Retval As Long
NextOp = NextOp + 1 Picture1.Cls
Select Case NextOp Case 1 Call ChordDemo Case 2 Call ElliDemo Case 3 Call RectDemo Case 4 Call RoundRectDemo Case 5 Call PolyDemo Case 6 Call MultiPolyDemo Case 7 Call PieDemo Case 8 Call MiscOperationDemo(1) Case 9 Call MiscOperationDemo(2) Case 10 Call MiscOperationDemo(3) Case 11 NextOp = 0 End Select
End Sub
Sub PieDemo() With WndRect Ret = Pie(Picture1.hdc, .left, .top, .right, .bottom, _ .right - .left / 1.5, .top, .right - .left / 1.5, .bottom) End With Me.Caption = "Chord Demo" End Sub
Sub ChordDemo() With WndRect Ret = Chord(Picture1.hdc, .left, .top, .right, .bottom, _ .right - .left / 1.5, .top, .right - .left / 1.5, .bottom) End With Me.Caption = "Chord Demo" End Sub
Sub RectDemo() With WndRect Ret = Rectangle(Picture1.hdc, .left, .top, .right, .bottom) End With Me.Caption = "Rectangle Demo" End Sub
Sub ElliDemo() With WndRect Ret = Ellipse(Picture1.hdc, .left, .top, .right, .bottom) End With Me.Caption = "Ellipse Demo" End Sub
Sub PolyDemo() Dim PolyPoints(2) As POINTAPI
PolyPoints(0).x = 0 PolyPoints(0).y = 0
PolyPoints(1).x = WndRect.right PolyPoints(1).y = 0
PolyPoints(2).x = WndRect.right / 2 PolyPoints(2).y = WndRect.bottom
Ret = Polygon(Picture1.hdc, PolyPoints(0), 3)
Me.Caption = "Polygon Region" End Sub
Sub MultiPolyDemo() Dim PolyPoints(5) As POINTAPI Dim FigurePtCnts(1) As Long
PolyPoints(0).x = 0 PolyPoints(0).y = 100
PolyPoints(1).x = 50 PolyPoints(1).y = 0
PolyPoints(2).x = 150 PolyPoints(2).y = 150
FigurePtCnts(0) = 3 PolyPoints(3).x = 150 PolyPoints(3).y = 0
PolyPoints(4).x = 200 PolyPoints(4).y = 100
PolyPoints(5).x = 50 PolyPoints(5).y = 150
FigurePtCnts(1) = 3
Ret = PolyPolygon(Picture1.hdc, PolyPoints(0), FigurePtCnts(0), 2)
Me.Caption = "Multi Polygon" End Sub
Sub RoundRectDemo() With WndRect Ret = RoundRect(Picture1.hdc, .left, .top, .right, .bottom, 50, 50) End With Me.Caption = "Rounded Rectangle " End Sub
Sub MiscOperationDemo(Optional OpCode As Integer = 1) Dim hBrush As Long, hOldBrush
hBrush = CreateHatchBrush(HS_DIAGCROSS, vbRed) hOldBrush = SelectObject(Picture1.hdc, hBrush)
Picture1.ScaleMode = 3
If OpCode = 1 Then Call ChordDemo Ret = FloodFill(Picture1.hdc, 100, 100, Picture1.Point(100, 100)) Me.Caption = Me.Caption & " [Fill]" End If
If OpCode = 2 Then RectDemo Retval = InvertRect(Picture1.hdc, WndRect) Me.Caption = Me.Caption & " [Invert]" End If
If OpCode = 3 Then RoundRectDemo Retval = FrameRect(Picture1.hdc, WndRect, hBrush) Me.Caption = Me.Caption & " [Frame]" End If
SelectObject Picture1.hdc, hOldBrush DeleteObject hBrush End Sub |
|