|
|
|
A brush is a graphics tool that applications use to paint the interior of polygons, ellipses, and paths. Drawing applications use brushes to paint shapes; word processing applications use brushes to paint rules; computer-aided design (CAD) applications use brushes to paint the interiors of cross-section views; and spreadsheet applications use brushes to paint the sections of pie charts and the bars in bar graphs.
Brush Types
There are four types of logical brushes: solid, stock, hatch, and pattern. These brushes are shown in the following illustration.
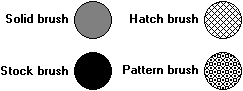
The stock and hatch types each have several predefined brushes. The CreateBrushIndirect function creates a logical brush with a specified style, color, and pattern.
- Solid Brush : A solid brush is a logical brush that contains 64 pixels of the same color. An application can create a solid logical brush by calling the CreateSolidBrush function, specifying the color of the brush required. After creating the solid brush, the application can select it into its device context and use it to paint filled shapes.
- Stock Brush : There are seven predefined logical stock brushes maintained by the graphics device interface (GDI). There are also 21 predefined logical stock brushes maintained by the window management interface (USER). An application can retrieve a handle identifying one of the seven stock brushes by calling the GetStockObject function, specifying the brush type.
The 21 stock brushes maintained by the window management interface correspond to the colors of window elements such as menus, scroll bars, and buttons. An application can obtain a handle identifying one of these brushes by calling the GetSysColorBrush function and specifying a system-color value. An application can retrieve the color corresponding to a particular window element by calling the GetSysColor function. An application can set the color corresponding to a window element by calling the SetSysColors function.
- Hatch Brush : There are six predefined logical hatch brushes maintained by GDI. The following rectangles were painted by using the six predefined hatch brushes.
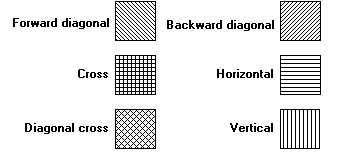
An application can create a hatch brush by calling the CreateHatchBrush function, specifying one of the six hatch styles.
- Pattern Brush : A pattern (or custom) brush is created from an application-defined bitmap or device-independent bitmap (DIB). The following rectangles were painted by using different pattern brushes.
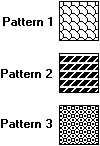
To create a logical pattern brush, an application must first create a bitmap. After creating the bitmap, the application can create the logical pattern brush by calling the CreatePatternBrush or CreateDIBPatternBrushPt function, supplying a handle that identifies the bitmap (or DIB). The brushes that appear in the preceding illustration were created from monochrome bitmaps. For a description of bitmaps, DIBs, and the functions that create them, see Bitmaps.
Brush Origin
When an application calls a drawing function to paint a shape, the system positions a brush at the start of the paint operation and maps a pixel in the brush bitmap to the client area at the window origin, which is the upper-left corner of the window. The coordinates of the pixel that the system maps are called the brush origin. The default brush origin is located in the upper-left corner of the brush bitmap, at the coordinates (0,0).
The Windows NT and Windows 2000 operating systems automatically track the origin of all window-managed DC's and adjust their brushes to maintain an alignment of patterns on the surface. Windows 95 doesn't support automatic tracking of the brush origin, so an application must call the following three functions to align a brush each time it paints using a patterned brush: |
Step-by-Step Example - Create a standard exe project - Add one picturebox control on the form1 - Add five commandbutton controls on the form1 - Add the following code in form1 Note: Make sure that test.gif exists in the application folder otherwise Pattern Brush Demo won't work |
Click here to copy the following block |
Private Declare Function GetBrushOrgEx Lib "gdi32" ( _ ByVal hdc As Long, _ lpPoint As POINTAPI) As Long
Private Declare Function SetBrushOrgEx Lib "gdi32" ( _ ByVal hdc As Long, _ ByVal nXOrg As Long, _ ByVal nYOrg As Long, _ lppt As POINTAPI) As Long
Private Declare Function CreateHatchBrush Lib "gdi32" ( _ ByVal nIndex As Long, _ ByVal crColor As Long) As Long
Private Declare Function SetDCBrushColor Lib "gdi32" ( _ ByVal hdc As Long, _ ByVal colorref As Long) As Long
Private Declare Function CreateBrushIndirect Lib "gdi32" ( _ lpLogBrush As LOGBRUSH) As Long
Private Declare Function CreateSolidBrush Lib "gdi32" ( _ ByVal crColor As Long) As Long
Private Declare Function GetSysColorBrush Lib "user32" ( _ ByVal nIndex As Long) As Long
Private Declare Function CreatePatternBrush Lib "gdi32" ( _ ByVal hBitmap As Long) As Long
Private Declare Function GetStockObject Lib "gdi32" ( _ ByVal nIndex As Long) As Long
Private Declare Function CreateBitmap Lib "gdi32" ( _ ByVal nWidth As Long, _ ByVal nHeight As Long, _ ByVal nPlanes As Long, _ ByVal nBitCount As Long, _ lpBits As Any) As Long
Private Declare Function SelectObject Lib "gdi32" (ByVal hdc As Long, _ ByVal hObject As Long) As Long
Private Declare Function Rectangle Lib "gdi32" (ByVal hdc As Long, _ ByVal X1 As Long, ByVal Y1 As Long, ByVal X2 As Long, _ ByVal Y2 As Long) As Long
Private Declare Function DeleteObject Lib "gdi32" (ByVal hObject As Long) _ As Long
Private Const HS_BDIAGONAL = 3 Private Const HS_CROSS = 4 Private Const HS_DIAGCROSS = 5 Private Const HS_FDIAGONAL = 2 Private Const HS_HORIZONTAL = 0 Private Const HS_VERTICAL = 1
Private Const COLOR_SCROLLBAR As Long = 0 Private Const COLOR_DESKTOP As Long = 1 Private Const COLOR_ACTIVECAPTION As Long = 2 Private Const COLOR_INACTIVECAPTION As Long = 3 Private Const COLOR_MENU As Long = 4 Private Const COLOR_WINDOW As Long = 5 Private Const COLOR_WINDOWFRAME As Long = 6 Private Const COLOR_MENUTEXT As Long = 7 Private Const COLOR_WINDOWTEXT As Long = 8 Private Const COLOR_CAPTIONTEXT As Long = 9 Private Const COLOR_ACTIVEBORDER As Long = 10 Private Const COLOR_INACTIVEBORDER As Long = 11 Private Const COLOR_APPWORKSPACE As Long = 12 Private Const COLOR_HIGHLIGHT As Long = 13 Private Const COLOR_HIGHLIGHTTEXT As Long = 14 Private Const COLOR_3DFACE As Long = 15 Private Const COLOR_3DSHADOW As Long = 16 Private Const COLOR_GRAYTEXT As Long = 17 Private Const COLOR_BTNTEXT As Long = 18 Private Const COLOR_INACTIVECAPTIONTEXT As Long = 19 Private Const COLOR_3DHIGHLIGHT As Long = 20 Private Const COLOR_3DDKSHADOW As Long = 21 Private Const COLOR_3DLIGHT As Long = 22 Private Const COLOR_INFOTEXT As Long = 23 Private Const COLOR_INFOBK As Long = 24 Private Const COLOR_HOTLIGHT As Long = 26 Private Const COLOR_GRADIENTACTIVECAPTION As Long = 27 Private Const COLOR_GRADIENTINACTIVECAPTION As Long = 28
Private Const WHITE_BRUSH = 0 Private Const LTGRAY_BRUSH = 1 Private Const GRAY_BRUSH = 2 Private Const DKGRAY_BRUSH = 3 Private Const BLACK_BRUSH = 4 Private Const NULL_BRUSH = 5 Private Const HOLLOW_BRUSH = NULL_BRUSH Private Const WHITE_PEN = 6 Private Const BLACK_PEN = 7 Private Const NULL_PEN = 8 Private Const OEM_FIXED_FONT = 10 Private Const ANSI_FIXED_FONT = 11 Private Const ANSI_VAR_FONT = 12 Private Const SYSTEM_FONT = 13 Private Const DEVICE_DEFAULT_FONT = 14 Private Const DEFAULT_PALETTE = 15 Private Const SYSTEM_FIXED_FONT = 16 Private Const STOCK_LAST = 16
Private Const BS_DIBPATTERN = 5 Private Const BS_DIBPATTERN8X8 = 8 Private Const BS_DIBPATTERNPT = 6 Private Const BS_HATCHED = 2 Private Const BS_NULL = 1 Private Const BS_HOLLOW = BS_NULL Private Const BS_PATTERN = 3 Private Const BS_PATTERN8X8 = 7 Private Const BS_SOLID = 0
Const DC_BRUSH = 18
Private Type POINTAPI x As Long y As Long End Type
Private Type LOGBRUSH lbStyle As Long lbColor As Long lbHatch As Long End Type
Private RetVal As Long Private hBrushNew As Long Private hBrushOld As Long Private OldOrginPt As POINTAPI Private NewOrginPt As POINTAPI
Const WD = 140 Const HT = 140
Private Sub Command1_Click() Picture1.Cls Call SolidBrushDemo End Sub
Private Sub Command2_Click() Picture1.Cls Call StockBrushDemo End Sub
Private Sub Command3_Click() Picture1.Cls Call HatchBrushDemo End Sub
Private Sub Command4_Click() Picture1.Cls Call PatternBrushDemo End Sub
Private Sub Command5_Click() Picture1.Cls Call IndirectBrushDemo End Sub
Sub SolidBrushDemo() hBrushNew = CreateSolidBrush(RGB(Rnd * 255, Rnd * 255, Rnd * 255)) hBrushOld = SelectObject(Picture1.hdc, hBrushNew)
RetVal = Rectangle(Picture1.hdc, WD / 8, HT / 8, WD * 7 / 8, HT * 3 / 8)
RetVal = SelectObject(Picture1.hdc, GetStockObject(DC_BRUSH)) RetVal = SetDCBrushColor(Picture1.hdc, vbRed) RetVal = Rectangle(Picture1.hdc, WD / 8, HT * 4 / 8, WD * 7 / 8, HT * 6 / 8)
Call SelectObject(Picture1.hdc, hBrushOld) End Sub
Sub StockBrushDemo() hBrushNew = GetStockObject(LTGRAY_BRUSH) hBrushOld = SelectObject(Picture1.hdc, hBrushNew)
RetVal = Rectangle(Picture1.hdc, WD / 8, HT / 8, WD * 7 / 8, HT * 3 / 8)
hBrushNew = GetSysColorBrush(COLOR_DESKTOP) hBrushOld = SelectObject(Picture1.hdc, hBrushNew) RetVal = Rectangle(Picture1.hdc, WD / 8, HT * 4 / 8, WD * 7 / 8, HT * 6 / 8)
Call SelectObject(Picture1.hdc, hBrushOld) End Sub
Sub HatchBrushDemo() hBrushNew = CreateHatchBrush(HS_FDIAGONAL, vbBlue) hBrushOld = SelectObject(Picture1.hdc, hBrushNew) RetVal = Rectangle(Picture1.hdc, WD / 8, HT / 8, WD * 7 / 8, HT * 3 / 8)
hBrushNew = CreateHatchBrush(HS_CROSS, vbMagenta) hBrushOld = SelectObject(Picture1.hdc, hBrushNew) RetVal = Rectangle(Picture1.hdc, WD / 8, HT * 4 / 8, WD - WD / 8, HT * 6 / 8)
Call SelectObject(Picture1.hdc, hBrushOld) End Sub
Sub PatternBrushDemo() Dim hBitmap As Long Dim bBytes(1 To 64) As Byte
Dim c As Integer
For I = 1 To 64 c = c + 1 If c <= 2 Then bBytes(I) = 1 Else bBytes(I) = 255 c = 0 End If Next
hBitmap = CreateBitmap(8, 8, 1, 8, bBytes(1)) hBrushNew = CreatePatternBrush(hBitmap) hBrushOld = SelectObject(Picture1.hdc, hBrushNew) RetVal = Rectangle(Picture1.hdc, WD / 8, HT / 8, WD * 7 / 8, HT * 3 / 8)
Me.Picture = LoadPicture(App.Path & "\test.gif") hBrushNew = CreatePatternBrush(Me.Picture.Handle) hBrushOld = SelectObject(Picture1.hdc, hBrushNew) RetVal = Rectangle(Picture1.hdc, WD / 8, HT * 4 / 8, WD - WD / 8, HT * 6 / 8)
Call SelectObject(Picture1.hdc, hBrushOld) End Sub
Sub IndirectBrushDemo() Dim lb As LOGBRUSH
lb.lbColor = vbYellow lb.lbStyle = BS_SOLID
hBrushNew = CreateBrushIndirect(lb) hBrushOld = SelectObject(Picture1.hdc, hBrushNew) RetVal = Rectangle(Picture1.hdc, WD / 8, HT / 8, WD * 7 / 8, HT * 3 / 8)
Me.Picture = LoadPicture(App.Path & "\test.gif") lb.lbColor = RGB(123, 144, 57) lb.lbHatch = HS_DIAGCROSS lb.lbStyle = BS_HATCHED
hBrushNew = CreateBrushIndirect(lb) hBrushOld = SelectObject(Picture1.hdc, hBrushNew) RetVal = Rectangle(Picture1.hdc, WD / 8, HT * 4 / 8, WD - WD / 8, HT * 6 / 8) Call SelectObject(Picture1.hdc, hBrushOld) End Sub
Private Sub Form_Load() Picture1.ScaleMode = vbPixels Picture1.Width = ScaleX(WD, vbPixels, vbTwips) Picture1.Height = ScaleY(HT, vbPixels, vbTwips)
Command1.Caption = "<< Solid Brush" Command2.Caption = "<< Stock Brush" Command3.Caption = "<< Hatch Brush" Command4.Caption = "<< Pattern Brush" Command5.Caption = "<< Brush Indirect"
RetVal = GetBrushOrgEx(Picture1.hdc, OldOrginPt) Call ChangeDefaultOrigin End Sub
Sub ChangeDefaultOrigin() With NewOrginPt .x = 4 .y = 4 RetVal = SetBrushOrgEx(Picture1.hdc, .x, .y, NewOrginPt) End With End Sub
Private Sub Form_QueryUnload(Cancel As Integer, UnloadMode As Integer) DeleteObject SelectObject(Picture1.hdc, hBrushOld) With OldOrginPt RetVal = SetBrushOrgEx(Picture1.hdc, .x, .y, OldOrginPt) End With End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
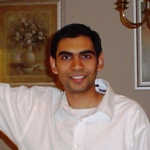
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|