|
|
|
There is no method call that directly retuns information about the number and type of installed CPU(s). However, this information is stored in a few environment variables, so it's just a matter of extracting it with the Environment.GetEnvironmentVariable method: |
This is a sample output produced by the previous code: Number of CPUs: 1 CPU Architecture: x86 CPU Identifier: x86 Family 6 Model 6 Stepping 2, AuthenticAMD CPU Level: 6 CPU Revision: 0602
|
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
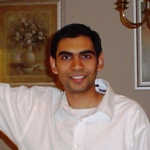
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|