|
|
|
Click here to copy the following block |
SELECT CASE WHEN (SELECT COUNT(*) FROM pubs..authors a2 WHERE a2.au_lname <= a1.au_lname and a2.au_fname <= a1.au_fname and a2.state = a1.state) = 1 THEN a1.State ELSE '' END as "State Field Header", a1.au_lname, a1.au_fname, a1.state FROM pubs..authors a1 ORDER BY a1.state, a1.au_lname, a1.au_fname |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
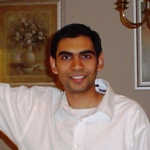
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|