|
|
|
The following code will extract the timezone information from your system. It includes three functions. One returns the current date/time in UTC (or ZULU or GMT) time, the next returns the offset of your time zone from GMT in minutes (divide by 60 to get hours), and the last returns the name of the time zone. This last function uses some WideChar to Ascii conversion functions that are only available on NT or 9x systems with the Microsoft Layer for Unicode on Windows 95/98/Me Systems installed, so be careful with this one. |
Click here to copy the following block | Option Explicit
Private Const CP_ACP = 0
Private Declare Function GetTimeZoneInformation Lib _ "kernel32" (lpTimeZoneInformation As TIME_ZONE_INFORMATION) As Long Private Declare Function lstrlenW Lib "kernel32" (lpString As Any) As Long Private Declare Function WideCharToMultiByte Lib "kernel32" _ (ByVal codepage As Long, ByVal dwFlags As Long, _ lpWideCharStr As Any, ByVal cchWideChar As Long, _ lpMultiByteStr As Any, ByVal cchMultiByte As Long, _ ByVal lpDefaultChar As String, ByVal lpUsedDefaultChar As Long) As Long
Private Type SYSTEMTIME wYear As Integer wMonth As Integer wDayOfWeek As Integer wDay As Integer wHour As Integer wMinute As Integer wSecond As Integer wMilliseconds As Integer End Type
Private Type TIME_ZONE_INFORMATION Bias As Long StandardName(32) As Integer StandardDate As SYSTEMTIME StandardBias As Long DaylightName(32) As Integer DaylightDate As SYSTEMTIME DaylightBias As Long End Type
Private Sub Form_Load() MsgBox "UTC Time :" & GetUTCTime MsgBox "TimeZone Offset" & GetTimeZoneOffset MsgBox "TimeZone Name" & GetTimeZoneName End Sub
Private Function GetUTCTime() As Date Dim tz As TIME_ZONE_INFORMATION Dim lRV As Long Dim dRV As Date
lRV = GetTimeZoneInformation(tz)
dRV = DateAdd("n", CDbl(tz.Bias), Now)
GetUTCTime = dRV End Function
Private Function GetTimeZoneOffset() As Integer Dim tz As TIME_ZONE_INFORMATION Dim lRV As Long Dim iRV As Integer
lRV = GetTimeZoneInformation(tz)
iRV = tz.Bias
GetTimeZoneOffset = iRV End Function
Private Function GetTimeZoneName() As String Dim tz As TIME_ZONE_INFORMATION Dim lRV As Long Dim sRV As String Dim lCounter As Long
Dim wchar(1) As Byte
lRV = GetTimeZoneInformation(tz)
sRV = GetStrFromPtrW(VarPtr(tz.StandardName(0)))
GetTimeZoneName = sRV End Function
Public Function GetStrFromANSIBuffer(sBuf As String) As String If InStr(sBuf, &H0) Then GetStrFromANSIBuffer = Left(sBuf, InStr(sBuf, &H0) - 1) Else GetStrFromANSIBuffer = sBuf End If End Function
Public Function GetStrFromPtrW(lpszW As Long) As String Dim sRV As String
sRV = String$(lstrlenW(ByVal lpszW) * 2, &H0)
WideCharToMultiByte CP_ACP, &H0, ByVal lpszW, -1, ByVal sRV, Len(sRV), &H0, &H0 GetStrFromPtrW = GetStrFromANSIBuffer(sRV) End Function |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
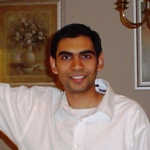
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|