|
|
|
You can use the VB.NET Shell command (in the Microsoft.VisualBasic namespace) to run an external process and wait for its termination, but you can get better control if you work directly with the Process class in the System.Diagnostics namespace. You can start a process with the Start static method (which returns a Process object), and then wait until the process exits by querying the WaitForExit instance method, which takes an optional timeout in milliseconds and returns True if the process has exited: |
Click here to copy the following block | Dim appexe As String = "C:\Program Files\Microsoft Office\Office\Winword.exe"
Dim wordProc As Process = Process.Start(appexe, "")
Do Until wordProc.WaitForExit(1000) Console.WriteLine("Waiting for Word to exit") Loop |
You can also check whether another process is still running by querying the HasExited readonly boolean property. There is also another method to get a notification when a process exits. You must set a handler for the Exited event and enable event raising by setting the EnableRaisingEvent property to True: |
Click here to copy the following block | Dim exited As Boolean
Sub Main() Dim appexe As String = _ "C:\Program Files\Microsoft Office\Office\Winword.exe" Dim wordProc As Process = Process.Start(appexe, "") AddHandler wordProc.Exited, AddressOf Process_Exited wordProc.EnableRaisingEvents = True
Do Threading.Thread.Sleep(100) Loop Until exited RemoveHandler wordProc.Exited, AddressOf Process_Exited End Sub
Private Sub Process_Exited(ByVal sender As Object, ByVal e As EventArgs) Console.WriteLine("Word has exited") exited = True End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
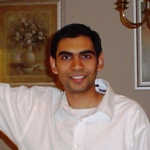
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|