|
|
|
The .NET framework lets you create two types of "empty" arrays: unitialized arrays and arrays that are initialized with zero elements. Uninitialized arrays are actually array variables that are set to Nothing, whereas zero-element arrays are non-Nothing variables that point to arrays with zero elements. Here is the (undocumented) method for creating zero-element arrays: |
If you have a routine that returns an array, you can decide whether you want to return Nothing or a zero-element array when an empty array should be returned. In general, returning a zero-element array makes for a more linear code in the caller. Consider this routine, that returns all the items in a string array that contain a given substring: |
Click here to copy the following block | Function Matches(ByVal arr() As String, ByVal Search As String) As String() Dim al As New ArrayList() Dim s As String For Each s In arr If s.IndexOf(Search) >= 0 Then al.Add Next If al.Count Then Return Nothing Dim res(al.Count - 1) As String Dim i As Integer For i = 0 To al.Count - 1 res(i) = al(i) Next Return res End Function |
The caller of the above routine must discern the Nothing case from regular case: |
Click here to copy the following block | Dim res() As String = Matches( arr, "Find this") If res Is Nothing Then Console.WriteLine("Found 0 matches") Else Console.WriteLine("Found {0} matches", res.Length) End If |
Now, consider what happens if you delete these two lines in the Matches routine: |
Not only is the Matches routine simpler, also the caller requires less code, because it doesn't have to check for Nothing first: |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
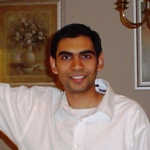
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|