|
|
|
If you fully understand how VB and VBA work, you can often save some statements. This makes your listings more concise and more readable, and indirectly optimizes your program. Here is a short list of the many tricks you can use with this goal. You don't need to initialize a numeric variable to 0 or a string variable to a null string when the variable is local to a procedure: |
The default return value for Function is zero or a null string, so you don't have to explicitly assign such values to the return value, unless you set a different value earlier in the procedure: |
Under VB4 and later versions it's OK for an error handler procedure to flow into an End Sub or End Function statement. In earlier versions an Exit Sub/Function was necessary. There is a lot of pre-VB4 code still around, that uses the longer approach, similar to the following code: |
If an argument is defined with ByVal you can freely modify it inside the procedure without any risk of side-effects in the main program. This often saves you a local variable: |
A similar point can be made with optional arguments. Even if the argument is found to be Missing, you can still use it without the need to declare a local variable. Moreover, assigning a value to it doesn't require you to use ByVal, because if the argument is missing the calling code won't be affected if you change it inside your procedure: |
Click here to copy the following block | Sub MyProc(Optional arg As Variant) Dim var As Variant If IsMissing(arg) Then var = Now() Else var = arg End If End Sub
Sub MyProc(Optional var As Variant) If IsMissing(var) Then var = Now() End Sub |
Under VB5 and later versions, you can define non-Variant optional parameters, and you can also define a default value for them. This means that you can re-write old VB4 code and optimize it using the following approach: |
A simple technique that often lets you save code in a Function is to use the Function's name as if it were a local variable (and in fact, it is a local variable!). In most cases the shortest version is also faster than the original one. For example: |
Click here to copy the following block | Function AppendStrings(ParamArray args() As Variant) As String Dim i As Integer Dim result As String For i = LBound(args) To UBound(args) result = result & args(i) Next AppendStrings = result End Function
Function AppendStrings(ParamArray args() As Variant) As String Dim i As Integer For i = LBound(args) To UBound(args) AppendStrings = AppendStrings & args(i) Next End Function |
When you're calling a Function but you're not interested in its return value - as it often occurs with API functions - you don't need a dummy variable just to hold the result. Instead, just call the function as if it were a procedure, which saves a Dim statement and makes you code faster. For example, the ClipCursor API function returns a non-zero value if successful, zero otherwise. If you aren't interested in trapping errors, or if you are absolutely sure that it can't raise an error (for example when you pass 0 to release the mouse clipping) you can use the function as if it were a Sub: |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
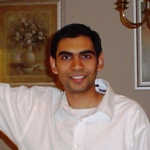
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|