|
|
|
While you can access individual pixels of a bitmap by means of the GetPixel and SetPixel methods of the Bitmap object, in practice you seldom want to use these methods, as they are simply too slow for most cpu-intensive operations. Fortunately there is a faster way, which consists of moving all the pixels to an array of bytes, process the array, and move its elements back to the bitmap. You have to use the Bitmap.LockBits method to get a BitmapData object, which you later use to retrieve the address of pixels in memory.
This example shows how to create a "pixelation" effect on a 8-bit-per-pixel bitmap. Notice that you must modify this code for other bitmap formats: |
Click here to copy the following block | Dim bmp As New Bitmap("c:\winnt\coffee bean.bmp")
Dim width As Integer = bmp.Width Dim height As Integer = bmp.Height
Dim rect As New Rectangle(0, 0, width, height) Dim bmpData As System.Drawing.Imaging.BitmapData = bmp.LockBits(Nothing, _ Drawing.Imaging.ImageLockMode.ReadWrite, _ Drawing.Imaging.PixelFormat.Format8bppIndexed)
Dim ptr As IntPtr = bmpData.Scan0
Dim bytes As Integer = width * height Dim pixels(bytes - 1) As Byte
Marshal.Copy(ptr, pixels, 0, bytes)
Dim r, c As Integer For r = 0 To height - 1 Step 2 For c = 0 To width - 1 Step 2 Dim index As Integer = c * height + r Dim colorValue As Byte = pixels(index) pixels(index + 1) = colorValue pixels(index + height) = colorValue pixels(index + height + 1) = colorValue Next Next
Marshal.Copy(pixels, 0, ptr, bytes)
bmp.UnlockBits(bmpData)
bmp.Save("c:\newbitmap.bmp") |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
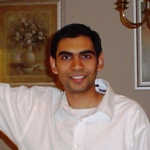
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|