|
|
|
The following code snippet will help you to perform string encryption/decryption using VB.net |
Click here to copy the following block | Imports System Imports System.Xml Imports System.Text Imports System.Security.Cryptography Imports System.IO
Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load Dim sMyPassword As String="mypass123" Dim sSecretData As String="Mission starts tonight at 9PM" Dim sEncryptedText As String Dim sDecryptedText As String sEncryptedText=Encrypt(sSecretData, sMyPassword) sDecryptedText=Decrypt(sEncryptedText, sMyPassword) Msgbox("Encrypted Text : " & sEncryptedText) Msgbox("Decrypted Text : " & sDecryptedText) End Function Private Function Encrypt(ByVal strText As String, ByVal strEncrKey As String) As String Dim byKey() As Byte = {} Dim IV() As Byte = {&H12, &H34, &H56, &H78, &H90, &HAB, &HCD, &HEF}
Try byKey = System.Text.Encoding.UTF8.GetBytes(Strings.Left(strEncrKey, 8))
Dim des As New DESCryptoServiceProvider() Dim inputByteArray() As Byte = Encoding.UTF8.GetBytes(strText) Dim ms As New MemoryStream() Dim cs As New CryptoStream(ms, des.CreateEncryptor(byKey, IV), CryptoStreamMode.Write) cs.Write(inputByteArray, 0, inputByteArray.Length) cs.FlushFinalBlock() Return Convert.ToBase64String(ms.ToArray())
Catch ex As Exception Return ex.Message End Try
End Function
Private Function Decrypt(ByVal strText As String, ByVal sDecrKey As String) As String Dim byKey() As Byte = {} Dim IV() As Byte = {&H12, &H34, &H56, &H78, &H90, &HAB, &HCD, &HEF} Dim inputByteArray(strText.Length) As Byte
Try byKey = System.Text.Encoding.UTF8.GetBytes(Strings.Left(sDecrKey, 8)) Dim des As New DESCryptoServiceProvider() inputByteArray = Convert.FromBase64String(strText) Dim ms As New MemoryStream() Dim cs As New CryptoStream(ms, des.CreateDecryptor(byKey, IV), CryptoStreamMode.Write)
cs.Write(inputByteArray, 0, inputByteArray.Length) cs.FlushFinalBlock() Dim encoding As System.Text.Encoding = System.Text.Encoding.UTF8
Return encoding.GetString(ms.ToArray())
Catch ex As Exception Return ex.Message End Try
End Function |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
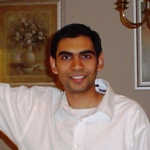
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|