|
|
|
I haven't tried this but it may be helpful to someone who is trying to display RTF formatted text on their report. Crystal Report Support RTF/HTML format but RS 2005 doesnt support. May be MS will add in the next release.
Just follow these steps:
Compile the code below it into a class
First copy the class in the C:\Program Files\Microsoft Visual Studio 8\Common7\IDE\PrivateAssemblies folder so it can be accessed by the designer.
Then copy the class in your C:\Program Files\Microsoft SQL Server\MSSQL.4\Reporting Services\ReportServer\bin (the MSSQL.(n) portion of the path varies from installation to installation.
Add the following lines to the C:\Program Files\Microsoft SQL Server\MSSQL.4\Reporting Services\ReportServer\rssrvpolicy.config |
Click here to copy the following block | <CodeGroup class="UnionCodeGroup" version="1" PermissionSetName="FullTrust" Name="RTF_Converter" Description="A special code group for my custom assembly."> <IMembershipCondition class="UrlMembershipCondition" version="1" Url="C:\Program Files\Microsoft SQL Server\MSSQL.4\Reporting Services\ReportServer\bin\RTFConverter.dll" /> </CodeGroup> |
Create your report. Add references to System.Drawing and your new custom class. Add this function to the reports Code section |
Click here to copy the following block | Public Function GetRTFImage(ByVal rtfText as String) As Byte() Dim rtfConv As New RTFConverter() rtfConv.Rtf = rtfText return RTFConverter.ConvertImageToByteArray(rtfConv.PrintToImage(500,50)) End Function |
Add an image to your report
Set the source to "Database"
Set the MIMEType to "image/bmp"
Set the value to "=Code.GetRTFImage(Fields!RTFText.Value)" |
Click here to copy the following block | using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Runtime.InteropServices;
using System.Drawing.Imaging;
using System.IO;
using System.Collections;
using System.Security.Permissions;
#region Class_RichTextImager
public partial class RichTextImager : RichTextBox
{
public RichTextImager()
{
}
protected override void OnPaint(PaintEventArgs e)
{
// Calling the base class OnPaint
base.OnPaint(e);
}
//Convert the unit used by the .NET framework (1/100 inch)
//and the unit used by Win32 API calls (twips 1/1440 inch)
private const double anInch = 14.4;
[StructLayout(LayoutKind.Sequential)]
private struct RECT
{
public int Left;
public int Top;
public int Right;
public int Bottom;
}
[StructLayout(LayoutKind.Sequential)]
private struct CHARRANGE
{
public int cpMin; //First character of range (0 for start of doc)
public int cpMax; //Last character of range (-1 for end of doc)
}
[StructLayout(LayoutKind.Sequential)]
private struct FORMATRANGE
{
public IntPtr hdc; //Actual DC to draw on
public IntPtr hdcTarget; //Target DC for determining text formatting
public RECT rc; //Region of the DC to draw to (in twips)
public RECT rcPage; //Region of the whole DC (page size) (in twips)
public CHARRANGE chrg; //Range of text to draw (see earlier declaration)
}
private const int WM_USER = 0x0400;
private const int EM_FORMATRANGE = WM_USER + 57;
[DllImport("USER32.dll")]
private static extern IntPtr SendMessage(IntPtr hWnd, int msg, IntPtr wp, IntPtr lp);
// Render the contents of the RichTextBox for printing
// Return the last character printed + 1 (printing start from this point for next page)
public int Print(int charFrom, int charTo, Graphics gr, Rectangle bounds)
{
//Calculate the area to render and print
RECT rectToPrint;
rectToPrint.Top = (int)(bounds.Top * anInch);
rectToPrint.Bottom = (int)(bounds.Height * anInch);// (int)(bounds.Bottom * anInch);
rectToPrint.Left = (int)(bounds.Left * anInch);
rectToPrint.Right = (int)(bounds.Width * anInch);// (int)(bounds.Right * anInch);
//Calculate the size of the page
RECT rectPage;
rectPage.Top = (int)(bounds.Top * anInch);
rectPage.Bottom = (int)(gr.ClipBounds.Height * anInch);//(int)(bounds.Bottom * anInch);
rectPage.Left = (int)(bounds.Left * anInch);
rectPage.Right = (int)(gr.ClipBounds.Right * anInch);//(int)(bounds.Right * anInch);
IntPtr hdc = gr.GetHdc();
FORMATRANGE fmtRange;
fmtRange.chrg.cpMax = charTo; //Indicate character from to character to
fmtRange.chrg.cpMin = charFrom;
fmtRange.hdc = hdc; //Use the same DC for measuring and rendering
fmtRange.hdcTarget = hdc; //Point at printer hDC
fmtRange.rc = rectToPrint; //Indicate the area on page to print
fmtRange.rcPage = rectPage; //Indicate size of page
IntPtr res = IntPtr.Zero;
IntPtr wparam = IntPtr.Zero;
wparam = new IntPtr(1);
//Get the pointer to the FORMATRANGE structure in memory
IntPtr lparam = IntPtr.Zero;
lparam = Marshal.AllocCoTaskMem(Marshal.SizeOf(fmtRange));
Marshal.StructureToPtr(fmtRange, lparam, false);
//System.Security.Permissions.SecurityPermission, mscorlib
SecurityPermission secPerm = new SecurityPermission(PermissionState.Unrestricted);
secPerm.Assert();
//Send the rendered data for printing
res = SendMessage(Handle, EM_FORMATRANGE, wparam, lparam);
//Free the block of memory allocated
Marshal.FreeCoTaskMem(lparam);
//Release the device context handle obtained by a previous call
gr.ReleaseHdc(hdc);
//Return last + 1 character printer
return res.ToInt32();
}
}
#endregion
#region Class_RichTextConverter
/// <summary>
/// Convert rich text to an image or byte array.
/// </summary>
public class RTFConverter
{
#region Class Members
private RichTextImager _Rtf = new RichTextImager();
private Int32 _lastChar = 0;
private Int32 _width = 640;
private Int32 _height = 480;
private Int32 _pageNumber = 0;
private Boolean _autoCrop = true;
#endregion
#region Contructors
public RTFConverter()
{
}
public RTFConverter(Int32 width, Int32 height)
{
this._width = width;
this._height = height;
}
public RTFConverter(String richText)
{
this.Rtf = richText;
}
public RTFConverter(String richText, Int32 width, Int32 height)
{
this.Rtf = richText;
this._width = width;
this._height = height;
}
public RTFConverter(String richText, Int32 width, Int32 height, Int32 pageNumber)
{
this.Rtf = richText;
this._width = width;
this._height = height;
this._pageNumber = pageNumber;
}
public RTFConverter(String richText, Int32 width, Int32 height, Int32 pageNumber, Boolean autoCrop)
{
this.Rtf = richText;
this._width = width;
this._height = height;
this._pageNumber = pageNumber;
this._autoCrop = autoCrop;
}
#endregion
#region Properties
/// <summary>
/// The plain text equivalent of the rich text to be rendered
/// </summary>
public string Text
{
get { return _Rtf.Text; }
set { _Rtf.Text = value; }
}
/// <summary>
/// The rich text to be rendered
/// </summary>
public string Rtf
{
get
{
UIPermission uiPerm = new UIPermission(PermissionState.Unrestricted);
uiPerm.Assert();
return _Rtf.Rtf;
}
set
{
UIPermission uiPerm = new UIPermission(PermissionState.Unrestricted);
uiPerm.Assert();
_Rtf.Rtf = value;
}
}
/// <summary>
/// The initial width of the returned image
/// </summary>
public Int32 Width
{
get{return _width;}
set{_width = value;}
}
/// <summary>
/// The initial height of the returned image
/// </summary>
public Int32 Height
{
get{return _height;}
set{_height = value;}
}
/// <summary>
/// The page number to be returned, 0 returns all pages
/// </summary>
public Int32 PageNumber
{
get{return _pageNumber;}
set{_pageNumber = value;}
}
/// <summary>
/// Specifies whether the returned image should be cropped to the rendered area only
/// </summary>
public Boolean AutoCrop
{
get{return _autoCrop;}
set{_autoCrop = value;}
}
#endregion
#region General Methods
public void LoadFile(String FilePath)
{
_Rtf.LoadFile(FilePath);
}
#endregion
#region PrintToImage
/// <summary>
/// Renders rich text to an image.
/// </summary>
/// <returns>Image</returns>
private Image GetImage()
{
Image image = new Bitmap(_width, _height);
Graphics g = Graphics.FromImage(image);
try
{
Int32 retVal = _Rtf.Print(_lastChar, this.Text.Length, g, new Rectangle(new Point(0, 0), new Size(_width, _height)));
_lastChar = retVal;
}
catch (SystemException ex)
{
g.DrawString(String.Format("Unable to render text. {0}", ex.Message), new Font("Arial", 10), Brushes.Black, new Point(0, 0));
}
if (_autoCrop)
{
return Crop(image);
}
else
{
return image;
}
}
/// <summary>
/// Generates an array list of images of the size specified.
/// </summary>
/// <returns>ArrayList</returns>
public ArrayList PrintToImageArrayList()
{
ArrayList aryImg = new ArrayList();
System.Drawing.Image pgImg;
if (this.Text.Length < 1)
{
pgImg = (Image)new Bitmap(1, 1);
aryImg.Add(pgImg);
return aryImg;
}
else
{
pgImg = GetImage();
aryImg.Add(pgImg);
}
while (_lastChar < this.Text.Length)
{
pgImg = GetImage();
aryImg.Add(pgImg);
}
return aryImg;
}
/// <summary>
/// Renders rich text to an image of the specified size and page.
/// </summary>
public Image PrintToImage()
{
ArrayList aryImg = PrintToImageArrayList();
if (_pageNumber == 0 && aryImg.Count == 1)
{
return (System.Drawing.Image)aryImg[0];
}
if (_pageNumber == 0 && aryImg.Count > 1)
{
System.Drawing.Image imgFull = new Bitmap(_width, (_height * aryImg.Count));
System.Drawing.Graphics g = System.Drawing.Graphics.FromImage(imgFull);
Int32 imgY = 0;
foreach (System.Drawing.Image imgPage in aryImg)
{
g.DrawImageUnscaled(imgPage, new Point(0, imgY));
imgY = imgY + imgPage.Height;
}
imgFull = Crop(imgFull);
return imgFull;
}
else if (_pageNumber > aryImg.Count)
{
_pageNumber = aryImg.Count;
return (System.Drawing.Image)aryImg[(_pageNumber - 1)];
}
else
{
return (System.Drawing.Image)aryImg[(_pageNumber - 1)];
}
}
/// <summary>
/// Renders rich text to an image of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <param name="pageNumber">The page number to be returned, 0 returns all pages</param>
/// <returns>Image</returns>
public Image PrintToImage(String richText, Int32 pageNumber)
{
this.Rtf = richText;
this._pageNumber = pageNumber;
return PrintToImage();
}
/// <summary>
/// Renders rich text to an image of the specified size and page.
/// </summary>
/// <param name="pageNumber">The page number to be returned, 0 returns all pages</param>
/// <returns>Image</returns>
public Image PrintToImage(Int32 pageNumber)
{
this._pageNumber = pageNumber;
return PrintToImage();
}
/// <summary>
/// Renders rich text to an image of the specified size and page.
/// </summary>
/// <param name="width">The initial width of the returned image</param>
/// <param name="height">The initial height of the returned image</param>
/// <returns>Image</returns>
public Image PrintToImage(Int32 width, Int32 height)
{
this._width = width;
this._height = height;
return PrintToImage();
}
/// <summary>
/// Renders rich text to an image of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <returns>Image</returns>
public Image PrintToImage(String richText)
{
this.Rtf = richText;
return PrintToImage();
}
/// <summary>
/// Renders rich text to an image of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <param name="width">The initial width of the returned image</param>
/// <param name="height">The initial height of the returned image</param>
/// <returns>Image</returns>
public Image PrintToImage(String richText, Int32 width, Int32 height)
{
this.Rtf = richText;
this._width = width;
this._height = height;
return PrintToImage();
}
/// <summary>
/// Renders rich text to an image of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <param name="width">The initial width of the returned image</param>
/// <param name="height">The initial height of the returned image</param>
/// <param name="pageNumber">The page number to be returned, 0 returns all pages</param>
/// <returns>Image</returns>
public Image PrintToImage(String richText, Int32 width, Int32 height, Int32 pageNumber)
{
this.Rtf = richText;
this._width = width;
this._height = height;
this._pageNumber = pageNumber;
return PrintToImage();
}
/// <summary>
/// Renders rich text to an image of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <param name="width">The initial width of the returned image</param>
/// <param name="height">The initial height of the returned image</param>
/// <param name="pageNumber">The page number to be returned, 0 returns all pages</param>
/// <param name="autoCrop">Specifies whether the returned image should be cropped to the rendered area only.</param>
/// <returns>Image</returns>
public Image PrintToImage(String richText, Int32 width, Int32 height, Int32 pageNumber, Boolean autoCrop)
{
this.Rtf = richText;
this._width = width;
this._height = height;
this._pageNumber = pageNumber;
this._autoCrop = autoCrop;
return PrintToImage();
}
#endregion
#region PrintToArray
public static byte[] ConvertImageToByteArray(System.Drawing.Image imageToConvert)
{
byte[] Ret;
using (System.IO.MemoryStream ms = new MemoryStream())
{
imageToConvert.Save(ms, ImageFormat.Bmp);
ms.Position = 0;
Ret = ms.ToArray();
}
return Ret;
}
/// <summary>
/// Renders rich text to an image byte array of the specified size and page.
/// </summary>
/// <returns>Image</returns>
public byte[] PrintToByteArray()
{
return ConvertImageToByteArray(PrintToImage());
}
/// <summary>
/// Renders rich text to an image byte array of the specified size and page.
/// </summary>
/// <param name="width">The initial width of the returned image</param>
/// <param name="height">The initial height of the returned image</param>
/// <returns>Image</returns>
public byte[] PrintToByteArray(Int32 width, Int32 height)
{
return ConvertImageToByteArray(PrintToImage(width, height));
}
/// <summary>
/// Renders rich text to an image byte array of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <returns>Image</returns>
public byte[] PrintToByteArray(String richText)
{
return ConvertImageToByteArray(PrintToImage(richText));
}
/// <summary>
/// Renders rich text to an image byte array of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <param name="width">The initial width of the returned image</param>
/// <param name="height">The initial height of the returned image</param>
/// <returns>Image</returns>
public byte[] PrintToByteArray(String richText, Int32 width, Int32 height)
{
return ConvertImageToByteArray(PrintToImage(richText, width, height));
}
/// <summary>
/// Renders rich text to an image byte array of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <param name="width">The initial width of the returned image</param>
/// <param name="height">The initial height of the returned image</param>
/// <param name="pageNumber">The page number to be returned, 0 returns all pages</param>
/// <returns>Image</returns>
public byte[] PrintToByteArray(String richText, Int32 width, Int32 height, Int32 pageNumber)
{
return ConvertImageToByteArray(PrintToImage(richText, width, height, pageNumber));
}
/// <summary>
/// Renders rich text to an image byte array of the specified size and page.
/// </summary>
/// <param name="richText">The rich text to be rendered</param>
/// <param name="width">The initial width of the returned image</param>
/// <param name="height">The initial height of the returned image</param>
/// <param name="pageNumber">The page number to be returned, 0 returns all pages</param>
/// <param name="autoCrop">Specifies whether the returned image should be cropped to the rendered area only.</param>
/// <returns>Image</returns>
public byte[] PrintToByteArray(String richText, Int32 width, Int32 height, Int32 pageNumber, Boolean autoCrop)
{
return ConvertImageToByteArray(PrintToImage(richText, width, height, pageNumber, autoCrop));
}
#endregion
#region Cropping
public static System.Drawing.Image Crop(System.Drawing.Image img, Int32 Top, Int32 Left, Int32 Width, Int32 Height)
{
Bitmap bmp = new Bitmap(Width, Height);
Graphics g = Graphics.FromImage(bmp);
g.DrawImageUnscaled(img, (Top * -1), (Left * -1), bmp.Width, bmp.Height);
return bmp;
}
public static System.Drawing.Image Crop(System.Drawing.Image img, Int32 Width, Int32 Height)
{
return Crop(img, 0, 0, Width, Height);
}
public static System.Drawing.Image Crop(System.Drawing.Image img, Color MaskColor)
{
System.Drawing.Bitmap clsBitmap = new System.Drawing.Bitmap(img);
System.Drawing.Graphics clsGraphics = Graphics.FromImage(clsBitmap);
System.Drawing.Color clrThisPixel = Color.White;
System.Drawing.Color clrMaskPixel = MaskColor;
Int32 _cropLeft = 0;
Int32 _cropTop = 0;
Int32 _cropWidth = clsBitmap.Width;
Int32 _cropHeight = clsBitmap.Height;
for (Int32 iT = 0; iT < clsBitmap.Height; iT += 1)
{
for (Int32 iL = 0; iL < clsBitmap.Width; iL += 1)
{
clrThisPixel = clsBitmap.GetPixel(iL, iT);
if (clrThisPixel.ToArgb() != clrMaskPixel.ToArgb())
{
_cropLeft = iL;
break;
}
}
if (clrThisPixel.ToArgb() != clrMaskPixel.ToArgb())
{
_cropTop = iT;
break;
}
}
for (Int32 iX = (clsBitmap.Width - 1); iX > 0; iX += -1)
{
for (Int32 iY = (clsBitmap.Height - 1); iY > 0; iY += -1)
{
clrThisPixel = clsBitmap.GetPixel(iX, iY);
if (clrThisPixel.ToArgb() != clrMaskPixel.ToArgb())
{
_cropHeight = (iY + 1);
break;
}
}
if (clrThisPixel.ToArgb() != clrMaskPixel.ToArgb())
{
_cropWidth = (iX + 1);
break;
}
}
return Crop(img, _cropTop, _cropLeft, (_cropWidth - _cropLeft), (_cropHeight - _cropTop));
}
public static System.Drawing.Image Crop(System.Drawing.Image img)
{
System.Drawing.Bitmap clsBitmap = new System.Drawing.Bitmap(img);
System.Drawing.Color clrMaskPixel = clsBitmap.GetPixel(clsBitmap.Width - 1, clsBitmap.Height - 1);
return Crop(img, clrMaskPixel);
}
#endregion
#endregion
} |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
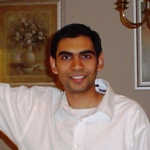
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|