|
|
|
In this article we will see all operators supported by VB.net
Here is the list of operators categories |
Category |
Operators |
Description |
Arithmetic Operators |
^, *, /, \, Mod, +, - |
These operators perform mathematical
calculations. |
Assignment Operators |
=, ^=, *=, /=, \=, +=, -=, <<=, >>=, &= |
These operators perform assignment
operations. |
Comparison Operators |
Is, Like, < (Less than), <= (Less than or equal to), > (Greater than), >= (Greater than or equal to), = (Equal to), <> (Not equal to) |
These operators perform comparisons. |
Concatenation Operators |
&, + |
These operators combine strings. |
Logical/Bitwise Operators |
And, Not, Or, Xor, AndAlso, OrElse |
These operators perform logical
operations. |
Bit Shift Operators |
<<, >> |
These operators perform arithmetic
shifts on bit patterns. |
Miscellaneous Operators |
AddressOf, GetType |
These operators perform miscellaneous
operations. |
|
Arithmetic Operators
These operators used to perform many familiar arithmetic operations that involve calculation of numeric values represented by literals, variables, other expressions, function and property calls, and constants.
^ Operator
Raises a number to the power of another number. |
* Operator
Multiplies two numbers. |
/ Operator
Divides two numbers and returns a floating-point result. |
\ Operator
Divides two numbers and returns an integer result. |
Mod Operator
Divides two numbers and returns only the remainder. |
+ Operator
Adds two numbers. Also used to concatenate two strings. |
- Operator
Yields the difference between two numbers or indicates the negative value of a numeric expression. |
Assignment Operators
= Operator
Used to assign a value to a variable or property |
Click here to copy the following block | Dim myInt as Integer Dim myString as String Dim myButton as System.Windows.Forms.Button Dim myObject as Object myInt = 42 myString = "This is an example of a string literal" myButton = New System.Windows.Forms.Button() myObject = myInt myObject = myString myObject = myButton |
^= Operator
Raises the value of a variable to the power of an expression and assigns the result back to the variable. |
*= Operator
Multiplies the value of a variable by the value of an expression and assigns the result to the variable. |
\= Operator
Divides the value of a variable by the value of an expression and assigns the integer result to the variable. |
+= Operator
Adds the value of an expression to the value of a variable and assigns the result to the variable. Also concatenates a String expression to a String variable and assigns the result to the variable. |
-= Operator
Subtracts the value of an expression from the value of a variable and assigns the result to the variable. |
<<= Operator
Performs an arithmetic left shift on the value of a variable and assigns the result back to the variable.
Arithmetic shifts are not circular, which means the bits shifted off one end of the result are not reintroduced at the other end. In an arithmetic left shift, the bits shifted beyond the range of the result data type are discarded, and the bit positions vacated on the right are set to zero. |
Click here to copy the following block | Dim Pattern As Short = 192 Dim Result1, Result2, Result3, Result4, Result5 As Short
Result1= Pattern Result2= Pattern Result3= Pattern Result4= Pattern Result5= Pattern
Result1 <<= 0 Result2 <<= 4 Result3 <<= 9 Result4 <<= 17 Result5 <<= -1 |
>>= Operator
Performs an arithmetic right shift on the value of a variable and assigns the result back to the variable.
Arithmetic shifts are not circular, which means the bits shifted off one end of the result are not reintroduced at the other end. In an arithmetic right shift, the bits shifted beyond the rightmost bit position are discarded, and the leftmost bit is propagated into the bit positions vacated at the left. This means that if variable has a negative value, the vacated positions are set to one. If variable is positive, or if its data type is Byte, the vacated positions are set to zero. |
Click here to copy the following block | Dim Pattern As Short = 192 Dim Result1, Result2, Result3, Result4, Result5 As Short
Result1= Pattern Result2= Pattern Result3= Pattern Result4= Pattern Result5= Pattern
Dim Pattern As Short = 2560 Dim Result1, Result2, Result3, Result4, Result5 As Short Result1 >>= 0 Result2 >> 4 Result3 >> 10 Result4 = Result4 >> 18 Result5 = Result5 >> -1 |
&= Operator
Concatenates a String expression to a String variable and assigns the result to the variable. |
Comparison Operators
These operators that compare two expressions and return a Boolean value representing the result of the comparison.
Is Operator
Compares two object reference variables.
The Is operator determines if two object references refer to the same object. However, it does not perform value comparisons. If object1 and object2 both refer to the same object, result is True; if they do not, result is False. |
Click here to copy the following block | Dim myObject As New Object Dim otherObject As New Object Dim yourObject, thisObject, thatObject As Object Dim myCheck As Boolean yourObject = myObject thisObject = myObject thatObject = otherObject myCheck = yourObject Is thisObject myCheck = thatObject Is thisObject myCheck = myObject Is thatObject |
Like Operator
Compares two strings. If string matches pattern, result is True; if there is no match, result is False. If both string and pattern are an empty string. the result is True. Otherwise, if either string or pattern is an empty string, the result is False. |
Characters in
pattern |
Matches in string |
? |
Any single character |
* |
Zero or more characters |
# |
Any single digit (0–9) |
[charlist] |
Any single character in charlist |
[!charlist] |
Any single character not in charlist |
|
Click here to copy the following block | Dim myCheck As Boolean
myCheck = "F" Like "F"
myCheck = "F" Like "f"
myCheck = "F" Like "FFF"
myCheck = "aBBBa" Like "a*a"
myCheck = "F" Like "[A-Z]"
myCheck = "F" Like "[!A-Z]"
myCheck = "a2a" Like "a#a"
myCheck = "aM5b" Like "a[L-P]#[!c-e]"
myCheck = "BAT123khg" Like "B?T*"
myCheck = "CAT123khg" Like "B?T*" |
Concatenation Operators
These operators join multiple strings into a single string.
+ and & Operators |
Logical/Bitwise Operators
Logical Operators provide information on operators that compare Boolean expressions and return a Boolean result. Bitwise Operators perform low level bit operations.
And Operator
Performs a logical conjunction on two Boolean expressions, or bitwise conjunction on two numeric expressions. |
Click here to copy the following block | Dim A As Integer = 10 Dim B As Integer = 8 Dim C As Integer = 6 Dim myCheck As Boolean
myCheck = A > B And B > C myCheck = B > A And B > C
myCheck = (A And B) myCheck = (A And C) myCheck = (B And C) |
Not Operator
Performs logical negation on a Boolean expression, or bitwise negation on a numeric expression. |
Click here to copy the following block | Dim A As Integer = 10 Dim B As Integer = 8 Dim C As Integer = 6 Dim myCheck As Boolean
myCheck = Not(A > B) myCheck = Not(B > A)
myCheck = (Not A) myCheck = (Not B) myCheck = (Not C) |
Or Operator
Used to perform a logical disjunction on two Boolean expressions, or bitwise disjunction on two numeric values..
If the operands consist of one Boolean expression and one numeric expression, the result Boolean expression will be converted to a numeric value (-1 for True, and 0 for False) and the bitwise operation will result. |
Click here to copy the following block | Dim A As Integer = 10 Dim B As Integer = 8 Dim C As Integer = 6 Dim myCheck As Boolean
myCheck = A > B Or B > C myCheck = B > A Or B > C myCheck = B > A Or C > B
myCheck = (A Or B) myCheck = (A Or C) myCheck = (B Or C) |
Xor Operator
Performs a logical exclusion operation on two Boolean expressions, or a bitwise exclusion on two numeric expressions.
For Boolean comparisons, if one and only one of the expressions evaluates to True, result is True. Otherwise, result is False. If either expression is stated as Nothing, that expression is evaluated as False.
If the operands consist of one Boolean expression and one numeric expression, the result Boolean expression will be converted to a numeric value (-1 for True, and 0 for False) and the bitwise operation will result. |
Click here to copy the following block | Dim A As Integer = 10 Dim B As Integer = 8 Dim C As Integer = 6 Dim myCheck As Boolean
myCheck = A > B Xor B > C myCheck = B > A Xor B > C myCheck = B > A Xor C > B
myCheck = (A Xor B) myCheck = (A Xor C) myCheck = (B Xor C) |
AndAlso Operator
Performs short-circuiting logical conjunction on two expressions.
A logical operation is said to be short-circuiting if the compiled code can bypass the evaluation of one expression depending on the result of another expression. If the result of the first expression evaluated determines the final result of the operation, there is no need to evaluate the other expression, because it cannot change the final result. Short-circuiting can improve performance if the bypassed expression is complex, or if it involves procedure calls. |
Click here to copy the following block | Dim A As Integer = 10 Dim B As Integer = 8 Dim C As Integer = 6
Dim myCheck As Boolean
myCheck = A > B AndAlso B > C myCheck = B > A AndAlso B > C myCheck = A > B AndAlso C > B |
A more common example for short-circuiting can be as follow. |
Click here to copy the following block | Public Function FindValue(ByVal Arr() As Double, _ ByVal SearchValue As Double) As Double Dim I As Integer = 0
While I <= UBound(Arr) AndAlso Arr(I) <> SearchValue I += 1 End While If I >= UBound(Arr) Then I = –1 Return I End Function |
In the above example if you use And instead of AndAlso then it will throw an exception if Arr() is empty because Arr(I) <> SearchValue condition will be executed regardless of first condition.
OrElse Operator
Used to perform short-circuiting logical disjunction on two expressions. |
Click here to copy the following block | Dim A As Integer = 10 Dim B As Integer = 8 Dim C As Integer = 6 Dim myCheck As Boolean myCheck = A > B OrElse B > C myCheck = B > A OrElse B > C myCheck = B > A OrElse C > B
If MyFunction(5) = True OrElse MyOtherFunction(4) = True Then
End If |
Bit Shift Operators
Ok so now not only C++ programmer can do bit shifting in one line but VB.net programmers have the same capability using bit shift operators. There are 2 new bit shift operators added in VB.net, Left shift operator(<<) and right shift operator(>>).
<< Operator
Performs an arithmetic left shift on a bit pattern.
Arithmetic shifts are not circular, which means the bits shifted off one end of the result are not reintroduced at the other end. In an arithmetic left shift, the bits shifted beyond the range of the result data type are discarded, and the bit positions vacated on the right are set to zero. |
>> Operator
Performs an arithmetic right shift on a bit pattern.
Arithmetic shifts are not circular, which means the bits shifted off one end of the result are not reintroduced at the other end. In an arithmetic right shift, the bits shifted beyond the rightmost bit position are discarded, and the leftmost (sign) bit is propagated into the bit positions vacated at the left. This means that if pattern has a negative value, the vacated positions are set to one; otherwise they are set to zero. |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
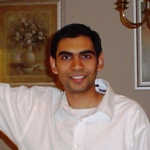
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|