|
|
|
In .Net using "System.ServiceProcess" namespace now its so easy to work with windows services. This article will show you how easy it is to get list of all running service or to check status of a single service by name. To control (i.e start/stop/paush) or retrive information of any service you need to work with "System.ServiceProcess.ServiceController" class.
Step-By-Step Example
- Create a new windows application project - Add new command button on the form (Button1) - Now right click on the references folder (mostly right side in the Solution Explorer) of your project and click "add reference...", Select "System.ServiceProcess.dll", click ok - Add the following code in your form and then press F5 to run the project, click on the button to see the demo
Note : You can not simply use Import Statement to add System.ServiceProcess reference so you must add it using "Add Reference..." |
Click here to copy the following block | Private Sub Button1_Click(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles Button1.Click
Dim srvname As String = "MSSQLSERVER" If IsServiceInstalled(srvname) = True Then Call ShowServiceStatus("localhost", srvname) Call ShowServices() Else MsgBox(srvname & " Service is not installed") End If End Sub
Function IsServiceInstalled(Optional ByVal ServiceName As String = "MSSQLSERVER", _ Optional ByVal MCName As String = "localhost") As Boolean
Try Dim s As String Dim sc As New System.ServiceProcess.ServiceController(ServiceName, MCName) s = sc.DisplayName
Return True Catch ex As Exception If Err.Number = 5 Then IsServiceInstalled = False Else IsServiceInstalled = True End If End Try End Function
Sub ShowServices(Optional ByVal MCName As String = "localhost", _ Optional ByVal OnlyShowRunning As Boolean = True)
Dim arrSc() As System.ServiceProcess.ServiceController Dim i
arrSc = ServiceProcess.ServiceController.GetServices(MCName)
Dim sb As New System.Text.StringBuilder For i = 0 To arrSc.GetUpperBound(0) If OnlyShowRunning = True Then If arrSc(i).Status = ServiceProcess.ServiceControllerStatus.Running Then sb.Append("[" & GetServiceStatusString(arrSc(i)) & "] " & _ arrSc(i).ServiceName & " (" & arrSc(i).DisplayName & ")" & vbCrLf) End If Else sb.Append("[" & GetServiceStatusString(arrSc(i)) & "] " & _ arrSc(i).ServiceName & " (" & arrSc(i).DisplayName & ")" & vbCrLf) End If Next
MsgBox(sb.ToString) End Sub
Sub ShowServiceStatus(Optional ByVal MCName As String = "localhost", _ Optional ByVal ServiceName As String = "MSSQLSERVER")
Dim sc As New System.ServiceProcess.ServiceController
sc.MachineName = MCName sc.ServiceName = ServiceName
MsgBox("MSSQLSERVER Service = > " & GetServiceStatusString(sc)) End Sub
Function GetServiceStatusString(ByVal sc As System.ServiceProcess.ServiceController) As String Select Case sc.Status Case ServiceProcess.ServiceControllerStatus.Running Return ("Running") Case ServiceProcess.ServiceControllerStatus.ContinuePending Return ("ContinuePending") Case ServiceProcess.ServiceControllerStatus.Paused Return ("Paused") Case ServiceProcess.ServiceControllerStatus.PausePending Return ("PausePending") Case ServiceProcess.ServiceControllerStatus.Stopped Return ("Stopped") Case ServiceProcess.ServiceControllerStatus.StopPending Return ("StopPending") End Select End Function |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
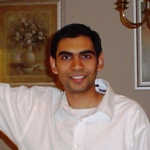
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|