|
|
|
Way back when Microsoft released Internet Explorer 4.0, they bundled with it a number of upgrades to the operating system including a new DLL called SHLWAPI.DLL (Shell Lightweight Utility APIs). That DLL provided programmers with some useful path manipulation functions (amongst other things), but obviously any applications that made use of those functions would also require that the user had installed Internet Explorer. Of course, now that Microsoft has 'integrated' their web browser into the operating system, the availability of SHLWAPI.DLL is no longer as much of an issue, so this article may seem a bit dated (it's been a while since I've had a chance to update this site). However, if you're still writing code for Windows 95 and NT 4.0, you might find it helpful to know that many of the functions in SHLWAPI.DLL are already available in a regular installation of Windows 95 in SHELL32.DLL - they're just not documented.
Now lets see different Path related APIs with examples
Testing Path
To start off with, we're going to look at some functions for testing paths. |
Click here to copy the following block |
Const MT_WORD_DOC = "application/msword" Const MT_TEXT_FILE = "text/plain" Const MT_DLL_EXE = "application/x-msdownload" Const MT_HTML = "text/html" Const MT_IMAGE_BMP = "image/bmp" Const MT_ADOBE_PDF = "application/pdf" Const MT_IMAGE_JPG = "image/jpeg" Const MT_XML = "text/xml"
Private Const GCT_INVALID = &H0 Private Const GCT_LFNCHAR = &H1 Private Const GCT_SEPARATOR = &H8 Private Const GCT_SHORTCHAR = &H2 Private Const GCT_WILD = &H4
Private Declare Function PathParseIconLocation Lib "shlwapi.dll" Alias "PathParseIconLocationA" ( _ ByVal pszIconFile As String) As Long Private Declare Function PathIsContentType Lib "shlwapi.dll" Alias "PathIsContentTypeA" ( _ ByVal pszPath As String, _ ByVal pszContentType As String) As Long Private Declare Function PathIsDirectory Lib "shlwapi.dll" Alias "PathIsDirectoryA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsDirectoryEmpty Lib "shlwapi.dll" Alias "PathIsDirectoryEmptyA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsFileSpec Lib "shlwapi.dll" Alias "PathIsFileSpecA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsLFNFileSpec Lib "shlwapi.dll" Alias "PathIsLFNFileSpecA" ( _ ByVal lpName As String) As Long Private Declare Function PathIsNetworkPath Lib "shlwapi.dll" Alias "PathIsNetworkPathA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsPrefix Lib "shlwapi.dll" Alias "PathIsPrefixA" ( _ ByVal pszPrefix As String, _ ByVal pszPath As String) As Long Private Declare Function PathIsRelative Lib "shlwapi.dll" Alias "PathIsRelativeA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsSameRoot Lib "shlwapi.dll" Alias "PathIsSameRootA" ( _ ByVal pszPath1 As String, _ ByVal pszPath2 As String) As Long Private Declare Function PathIsRoot Lib "shlwapi.dll" Alias "PathIsRootA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsSystemFolder Lib "shlwapi.dll" Alias "PathIsSystemFolderA" ( _ ByVal pszPath As String, _ ByVal dwAttrb As Long) As Long Private Declare Function PathIsUNC Lib "shlwapi.dll" Alias "PathIsUNCA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsUNCServer Lib "shlwapi.dll" Alias "PathIsUNCServerA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsUNCServerShare Lib "shlwapi.dll" Alias "PathIsUNCServerShareA" ( _ ByVal pszPath As String) As Long Private Declare Function PathIsURL Lib "shlwapi.dll" Alias "PathIsURLA" ( _ ByVal pszPath As String) As Long Private Declare Function PathMakeSystemFolder Lib "shlwapi.dll" Alias "PathMakeSystemFolderA" ( _ ByVal pszPath As String) As Long Private Declare Function PathFileExists Lib "shlwapi.dll" Alias "PathFileExistsA" ( _ ByVal pszPath As String) As Long Private Declare Function PathFindOnPath Lib "shlwapi.dll" Alias "PathFindOnPathA" ( _ ByVal pszPath As String, ByVal ppszOtherDirs As String) As Boolean Private Declare Function PathGetCharType Lib "shlwapi.dll" Alias "PathGetCharTypeA" ( _ ByVal ch As Byte) As Long Private Declare Function PathGetDriveNumber Lib "shlwapi.dll" Alias "PathGetDriveNumberA" ( _ ByVal pszPath As String) As Long Private Declare Function PathMatchSpec Lib "shlwapi.dll" Alias "PathMatchSpecA" ( _ ByVal pszFile As String, _ ByVal pszSpec As String) As Long
Private Sub Demo3() Dim strPath1 As String, strPath2 As String
MsgBox "PathIsURL >> " & "index is " & PathParseIconLocation("c:\test.bmp,3"), , "icon index demo"
strPath1 = "c:\test.bmp"
If PathIsContentType(strPath1, MT_IMAGE_BMP) Then MsgBox "PathIsContentType >> " & strPath1 & " is " & MT_IMAGE_BMP, vbInformation, "Content Type Demo" Else MsgBox "PathIsContentType >> " & strPath1 & " is " & MT_IMAGE_BMP, vbInformation, "Content Type Demo" End If
strPath1 = "c:\system32"
If PathIsDirectory(strPath1) Then MsgBox "PathIsDirectory >> " & strPath1 & " is a valid directory", vbInformation, "Check Directory Demo" Else MsgBox "PathIsDirectory >> " & strPath1 & " is not a valid directory", vbInformation, "Check Directory Demo" End If
strPath1 = "c:\testdir1" MkDir strPath1
If PathIsDirectoryEmpty(strPath1) Then MsgBox "PathIsDirectoryEmpty >> " & strPath1 & " is an empty directory", vbInformation, "Check Empty Directory Demo" Else MsgBox "PathIsDirectoryEmpty >> " & strPath1 & " is not an empty directory", vbInformation, "Check Empty Directory Demo" End If RmDir strPath1
strPath1 = "testfile.txt"
If PathIsFileSpec(strPath1) Then MsgBox "PathIsFileSpec >> " & strPath1 & " is File Spec Path", vbInformation, "Check File Spec Path Demo" Else MsgBox "PathIsFileSpec >> " & strPath1 & " is not a File Spec Path", vbInformation, "Check File Spec Path Demo" End If
strPath1 = "c:\Program Files\Microsoft SQL Server\MSSQL\"
If PathIsLFNFileSpec(strPath1) Then MsgBox "PathIsLFNFileSpec >> " & strPath1 & " is Long Path", vbInformation, "Check Long Path Demo" Else MsgBox "PathIsLFNFileSpec >> " & strPath1 & " is not Long Path", vbInformation, "Check Long Path Demo" End If
strPath1 = "c:\api"
If PathIsNetworkPath(strPath1) Then MsgBox "PathIsNetworkPath >> " & strPath1 & " is a Network Path", vbInformation, "Check Network Path Demo" Else MsgBox "PathIsNetworkPath >> " & strPath1 & " is not a Network Path", vbInformation, "Check Network Path Demo" End If
strPath1 = "c:\api"
If PathIsPrefix("c:\", strPath1) Then MsgBox "PathIsPrefix >> " & strPath1 & " is matching with prefix", vbInformation, "Check Prefix Demo" Else MsgBox "PathIsPrefix >> " & strPath1 & " is not matching with prefix", vbInformation, "Check Prefix Demo" End If
strPath1 = "c:\InetPub": strPath2 = "C:\windows"
If PathIsSameRoot(strPath1, strPath2) Then MsgBox "PathIsSameRoot >> " & strPath1 & " and " & strPath2 & " both have same root", vbInformation, "Check Relative Demo" Else MsgBox "PathIsSameRoot >> " & strPath1 & " and " & strPath2 & " both have not same root", vbInformation, "Check Relative Demo" End If
strPath1 = "../Images/user.gif"
If PathIsRelative(strPath1) Then MsgBox "PathIsRelative >> " & strPath1 & " is relative path", vbInformation, "Check Relative Demo" Else MsgBox "PathIsRelative >> " & strPath1 & " is absolute path", vbInformation, "Check Relative Demo" End If
strPath1 = "C:\InetPub"
If PathIsRoot(strPath1) Then MsgBox "PathIsRoot >> " & strPath1 & " is root", vbInformation, "Check Root Demo" Else MsgBox "PathIsRoot >> " & strPath1 & " is not root", vbInformation, "Check Root Demo" End If
strPath1 = "C:\InetPub"
If PathIsSystemFolder(strPath1, 0) Then MsgBox "PathIsSystemFolder >> " & strPath1 & " is system folder", vbInformation, "Check System folder Demo" Else MsgBox "PathIsSystemFolder >> " & strPath1 & " is not system folder", vbInformation, "Check System folder Demo" End If
strPath1 = "\\dhana\api"
If PathIsUNC(strPath1) Then MsgBox "PathIsUNC >> " & strPath1 & " is UNC", vbInformation, "Check UNC path Demo" Else MsgBox "PathIsUNC >> " & strPath1 & " is not UNC", vbInformation, "Check UNC path Demo" End If
strPath1 = "\\." strPath1 = "\\dhana\api"
If PathIsUNCServer(strPath1) Then MsgBox "PathIsUNCServer >> " & strPath1 & " is UNC Server", vbInformation, "Check UNC Server path Demo" Else MsgBox "PathIsUNCServer >> " & strPath1 & " is not UNC Server", vbInformation, "Check UNC Server path Demo" End If
strPath1 = "\\dhana\api"
If PathIsUNCServerShare(strPath1) Then MsgBox "PathIsUNCServerShare >> " & strPath1 & " is UNC Server share", vbInformation, "Check UNC Server share Demo" Else MsgBox "PathIsUNCServerShare >> " & strPath1 & " is not UNC Server", vbInformation, "Check UNC Server share Demo" End If
strPath1 = "http://binaryworld.net:6576/main"
If PathIsURL(strPath1) Then MsgBox "PathIsURL >> " & strPath1 & " is URL", vbInformation, "Check URL Demo" Else MsgBox "PathIsURL >> " & strPath1 & " is not URL", vbInformation, "Check URL Demo" End If
strPath1 = "c:\boot.ini"
If PathFileExists(strPath1) Then MsgBox "PathFileExists >> " & strPath1 & " exists", vbInformation, "Check File existance" Else MsgBox "PathFileExists >> " & strPath1 & " doesn't exist", vbInformation, "Check File existance" End If
strPath1 = "regsvr32.exe"
If PathFindOnPath(strPath1, vbNullString) Then MsgBox "PathFindOnPath >> " & strPath1 & " the file is located a PATH-directory", vbInformation, "Check File existance in path variable" Else MsgBox "PathFindOnPath >> " & strPath1 & " the file is not located a PATH-directory", vbInformation, "Check File existance in path variable" End If
Dim ch As String, strOutBuffer As String ch = ">" Select Case PathGetCharType(Asc(ch)) Case GCT_INVALID strOutBuffer = "invalid" Case GCT_LFNCHAR strOutBuffer = "valid in a long file name" Case GCT_SHORTCHAR strOutBuffer = "valid in a short (8.3) file name" Case GCT_WILD strOutBuffer = "a wildcard character" Case GCT_SEPARATOR strOutBuffer = "a path separator" End Select MsgBox "PathGetCharType >> The character '" & ch & "' is " + strOutBuffer
strPath1 = "d:\test.txt" MsgBox "PathGetDriveNumber >> The file is located on drive " + Chr$(65 + PathGetDriveNumber(strPath1))
Dim ext As String strPath1 = "test.bmp" ext = "*.b??" ext = "*.ec?"
If PathMatchSpec(strPath1, ext) Then MsgBox "PathMatchSpec >> " & strPath1 & " extension matches with " & ext, vbInformation, "Match Spec" Else MsgBox "PathMatchSpec >> " & strPath1 & " extension does not match with " & ext, vbInformation, "Match Spec" End If End Sub
Private Sub Form_Load() Demo3 End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
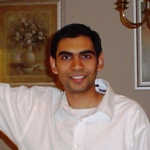
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|