|
|
|
This article illustrates how to programmatically retrieve IP configuration information similar to the IPCONFIG.EXE utility. It demonstrates how to use the IP Helper APIs GetNetworkParams() and GetAdaptersInfo() from Visual Basic.
The libraries called by the code sample in this article are only supported on the following platforms: Microsoft Windows 2000 Microsoft Windows 98 Microsoft Windows Millennium Edition (Me) Running it on any other platform results in an error.
Here is the sample output this code |
Host Name : it15666
DNS Servers : 192.168.2.1
DNS Servers : 172.16.254.13
DNS Servers : 172.16.254.14
Node type : Hybrid
NetBIOS Scope ID :
IP Routing not enabled
WINS Proxy not Enabled
NetBIOS Resolution Does not use DNS
-----------------------------------------------------------------------------
Adapter [#1]
-----------------------------------------------------------------------------
Adapter name : Ethernet adapter
AdapterDescription : Belkin 11Mbps Wireless Notebook Network Card
Physical Address : 0-30-ED-4D-37-62
DHCP Enabled
IP Address : 192.168.2.73
Subnet Mask : 255.255.255.0
Default Gateway : 192.168.2.1
DHCP Server : 192.168.2.1
Primary WINS Server : 0.0.0.0
Secondary WINS Server : 0.0.0.0
Lease Obtained : Tuesday, Nov 23 00:49:04 2004
Lease Expires : Tuesday, Jan 19 03:14:07 2038
-----------------------------------------------------------------------------
Adapter [#2]
-----------------------------------------------------------------------------
Adapter name : Ethernet adapter
AdapterDescription : Intel(R) PRO Adapter
Physical Address : 0-10-A4-D-DA-FD
DHCP Enabled
IP Address : 0.0.0.0
Subnet Mask : 0.0.0.0
Default Gateway :
DHCP Server : 172.16.254.20
Primary WINS Server : 172.16.254.20
Secondary WINS Server : 172.16.254.20
Lease Obtained : Monday, Nov 22 14:17:12 2004
Lease Expires : Saturday, Nov 27 14:17:12 2004 |
Step-By-Step Example
- Create a standard exe project - Add one Listbox control on the form1 - Add the following code in form1 |
Click here to copy the following block | Private Const MAX_HOSTNAME_LEN = 132 Private Const MAX_DOMAIN_NAME_LEN = 132 Private Const MAX_SCOPE_ID_LEN = 260 Private Const MAX_ADAPTER_NAME_LENGTH = 260 Private Const MAX_ADAPTER_ADDRESS_LENGTH = 8 Private Const MAX_ADAPTER_DESCRIPTION_LENGTH = 132 Private Const ERROR_BUFFER_OVERFLOW = 111 Private Const MIB_IF_TYPE_ETHERNET = 6 Private Const MIB_IF_TYPE_TOKENRING = 9 Private Const MIB_IF_TYPE_FDDI = 15 Private Const MIB_IF_TYPE_PPP = 23 Private Const MIB_IF_TYPE_LOOPBACK = 24 Private Const MIB_IF_TYPE_SLIP = 28
Private Type IP_ADDR_STRING Next As Long IpAddress As String * 16 IpMask As String * 16 Context As Long End Type
Private Type IP_ADAPTER_INFO Next As Long ComboIndex As Long AdapterName As String * MAX_ADAPTER_NAME_LENGTH Description As String * MAX_ADAPTER_DESCRIPTION_LENGTH AddressLength As Long Address(MAX_ADAPTER_ADDRESS_LENGTH - 1) As Byte Index As Long Type As Long DhcpEnabled As Long CurrentIpAddress As Long IpAddressList As IP_ADDR_STRING GatewayList As IP_ADDR_STRING DhcpServer As IP_ADDR_STRING HaveWins As Byte PrimaryWinsServer As IP_ADDR_STRING SecondaryWinsServer As IP_ADDR_STRING LeaseObtained As Long LeaseExpires As Long End Type
Private Type FIXED_INFO HostName As String * MAX_HOSTNAME_LEN DomainName As String * MAX_DOMAIN_NAME_LEN CurrentDnsServer As Long DnsServerList As IP_ADDR_STRING NodeType As Long ScopeId As String * MAX_SCOPE_ID_LEN EnableRouting As Long EnableProxy As Long EnableDns As Long End Type
Private Declare Function GetNetworkParams Lib "IPHlpApi.dll" _ (FixedInfo As Any, pOutBufLen As Long) As Long Private Declare Function GetAdaptersInfo Lib "IPHlpApi.dll" _ (IpAdapterInfo As Any, pOutBufLen As Long) As Long Private Declare Sub CopyMemory Lib "kernel32" Alias "RtlMoveMemory" _ (Destination As Any, Source As Any, ByVal Length As Long)
Dim sMsg As String
Sub ShowIPConfig() Dim error As Long Dim FixedInfoSize As Long Dim AdapterInfoSize As Long Dim i As Integer Dim PhysicalAddress As String Dim NewTime As Date Dim AdapterInfo As IP_ADAPTER_INFO Dim AddrStr As IP_ADDR_STRING Dim FixedInfo As FIXED_INFO Dim Buffer As IP_ADDR_STRING Dim pAddrStr As Long Dim pAdapt As Long Dim Buffer2 As IP_ADAPTER_INFO Dim FixedInfoBuffer() As Byte Dim AdapterInfoBuffer() As Byte
FixedInfoSize = 0 error = GetNetworkParams(ByVal 0&, FixedInfoSize) If error <> 0 Then If error <> ERROR_BUFFER_OVERFLOW Then AddInfo "GetNetworkParams sizing failed with error " & error Exit Sub End If End If ReDim FixedInfoBuffer(FixedInfoSize - 1)
error = GetNetworkParams(FixedInfoBuffer(0), FixedInfoSize)
If error = 0 Then CopyMemory FixedInfo, FixedInfoBuffer(0), FixedInfoSize AddInfo FixStr("Host Name") & ": " & FixedInfo.HostName AddInfo FixStr("DNS Servers") & ": " & FixedInfo.DnsServerList.IpAddress pAddrStr = FixedInfo.DnsServerList.Next Do While pAddrStr <> 0 CopyMemory Buffer, ByVal pAddrStr, LenB(Buffer) AddInfo FixStr("DNS Servers") & ": " & Buffer.IpAddress pAddrStr = Buffer.Next Loop
Select Case FixedInfo.NodeType Case 1 AddInfo FixStr("Node type") & ": Broadcast" Case 2 AddInfo FixStr("Node type") & ": Peer to peer" Case 4 AddInfo FixStr("Node type") & ": Mixed" Case 8 AddInfo FixStr("Node type") & ": Hybrid" Case Else AddInfo FixStr("Node type") & ": UNKNOWN" End Select
AddInfo FixStr("NetBIOS Scope ID") & ": " & FixedInfo.ScopeId If FixedInfo.EnableRouting Then AddInfo "IP Routing Enabled " Else AddInfo "IP Routing not enabled" End If If FixedInfo.EnableProxy Then AddInfo "WINS Proxy Enabled " Else AddInfo "WINS Proxy not Enabled " End If If FixedInfo.EnableDns Then AddInfo "NetBIOS Resolution Uses DNS " Else AddInfo "NetBIOS Resolution Does not use DNS " End If Else AddInfo "GetNetworkParams failed with error " & error Exit Sub End If
AdapterInfoSize = 0 error = GetAdaptersInfo(ByVal 0&, AdapterInfoSize) If error <> 0 Then If error <> ERROR_BUFFER_OVERFLOW Then AddInfo "GetAdaptersInfo sizing failed with error " & error Exit Sub End If End If
ReDim AdapterInfoBuffer(AdapterInfoSize - 1)
error = GetAdaptersInfo(AdapterInfoBuffer(0), AdapterInfoSize) If error <> 0 Then AddInfo "GetAdaptersInfo failed with error " & error Exit Sub End If
CopyMemory AdapterInfo, AdapterInfoBuffer(0), AdapterInfoSize pAdapt = AdapterInfo.Next
Dim cnt As Integer Do cnt = cnt + 1 List1.AddItem String(100, "-") List1.AddItem "Adapter [#" & cnt & "]" List1.AddItem String(100, "-") CopyMemory Buffer2, AdapterInfo, AdapterInfoSize Select Case Buffer2.Type Case MIB_IF_TYPE_ETHERNET AddInfo Space(5) & FixStr("Adapter name") & ": Ethernet adapter " Case MIB_IF_TYPE_TOKENRING AddInfo Space(5) & FixStr("Adapter name") & ": Token Ring adapter " Case MIB_IF_TYPE_FDDI AddInfo Space(5) & FixStr("Adapter name") & ": FDDI adapter " Case MIB_IF_TYPE_PPP AddInfo Space(5) & FixStr("Adapter name") & ": PPP adapter" Case MIB_IF_TYPE_LOOPBACK AddInfo Space(5) & FixStr("Adapter name") & ": Loopback adapter " Case MIB_IF_TYPE_SLIP AddInfo Space(5) & FixStr("Adapter name") & ": Slip adapter " Case Else AddInfo Space(5) & FixStr("Adapter name") & ": Other adapter " End Select AddInfo Space(5) & FixStr("AdapterDescription") & ": " & Buffer2.Description
PhysicalAddress = "" For i = 0 To Buffer2.AddressLength - 1 PhysicalAddress = PhysicalAddress & Hex(Buffer2.Address(i)) If i < Buffer2.AddressLength - 1 Then PhysicalAddress = PhysicalAddress & "-" End If Next AddInfo Space(5) & FixStr("Physical Address") & ": " & PhysicalAddress
If Buffer2.DhcpEnabled Then AddInfo Space(5) & "DHCP Enabled " Else AddInfo Space(5) & "DHCP disabled" End If
AddInfo Space(5) & FixStr("IP Address") & ": " & Buffer2.IpAddressList.IpAddress AddInfo Space(5) & FixStr("Subnet Mask") & ": " & Buffer2.IpAddressList.IpMask pAddrStr = Buffer2.IpAddressList.Next Do While pAddrStr <> 0 CopyMemory Buffer, Buffer2.IpAddressList, LenB(Buffer) AddInfo Space(5) & FixStr("IP Address") & ": " & Buffer.IpAddress AddInfo Space(5) & FixStr("Subnet Mask") & ": " & Buffer.IpMask pAddrStr = Buffer.Next If pAddrStr <> 0 Then CopyMemory Buffer2.IpAddressList, ByVal pAddrStr, _ LenB(Buffer2.IpAddressList) End If Loop
AddInfo Space(5) & FixStr("Default Gateway") & ": " & Buffer2.GatewayList.IpAddress pAddrStr = Buffer2.GatewayList.Next Do While pAddrStr <> 0 CopyMemory Buffer, Buffer2.GatewayList, LenB(Buffer) AddInfo Space(5) & FixStr("IP Address") & ": " & Buffer.IpAddress pAddrStr = Buffer.Next If pAddrStr <> 0 Then CopyMemory Buffer2.GatewayList, ByVal pAddrStr, _ LenB(Buffer2.GatewayList) End If Loop
AddInfo Space(5) & FixStr("DHCP Server") & ": " & Buffer2.DhcpServer.IpAddress AddInfo Space(5) & FixStr("Primary WINS Server") & ": " & _ Buffer2.PrimaryWinsServer.IpAddress AddInfo Space(5) & FixStr("Secondary WINS Server") & ": " & _ Buffer2.SecondaryWinsServer.IpAddress
NewTime = DateAdd("s", Buffer2.LeaseObtained, #1/1/1970#) AddInfo Space(5) & FixStr("Lease Obtained") & ": " & _ CStr(Format(NewTime, "dddd, mmm d hh:mm:ss yyyy"))
NewTime = DateAdd("s", Buffer2.LeaseExpires, #1/1/1970#) AddInfo Space(5) & FixStr("Lease Expires ") & ": " & _ CStr(Format(NewTime, "dddd, mmm d hh:mm:ss yyyy")) pAdapt = Buffer2.Next If pAdapt <> 0 Then CopyMemory AdapterInfo, ByVal pAdapt, AdapterInfoSize End If Loop Until pAdapt = 0
sMsg = "" For i = 0 To List1.ListCount - 1 sMsg = sMsg & List1.List(i) & vbCrLf Next Clipboard.SetText sMsg MsgBox "Information copied to clipboard", vbInformation End Sub
Function AddInfo(sMsg As String) List1.AddItem TrimNull(sMsg) End Function
Private Sub Form_Load() If IsRunningIDE Then MsgBox "Run compiled version to prevent application crash", vbExclamation End If Call ShowIPConfig End Sub
Function FixStr(sMsg As String, Optional MaxLen As Integer = 25) As String Dim sPad As String Dim c As Long c = MaxLen - Len(sMsg) sPad = IIf(c > 0, Space(c), "")
FixStr = Left(sMsg, MaxLen) & sPad End Function Private Function TrimNull(item As String) As String Dim pos As Integer pos = InStr(item, Chr$(0)) If pos Then TrimNull = Left$(item, pos - 1) Else: TrimNull = item End If End Function
Function IsRunningIDE() As Boolean On Error GoTo errHandler
Debug.Print 1 / 0
Exit Function errHandler: IsRunningIDE = True End Function |
- Compile the project - Run the exe |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
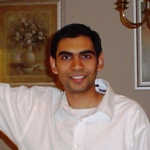
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|