|
|
|
Sometimes you might need to create a window using code on the fly. Many times I have been asked that why do I write this odd code to create a very simple form,I can use VB form instead of Dynamic C style window creation....
Now here is the answer
One common use of this technique in socket programming is to create a hidden window (i.e form) on the fly and use that Window Handle to receive winsock mesages. CreateWindowEx is not limited to form, you can create any window (i.e. tooltip, button, listbox, combobox...)
This code will show you Pure C Style window creation which responds window messages from Windows Procedure.
To use it, simply create a new standard exe project and add a module to it, remove Form1, and paste the code into the module. Set your project's default startup object to Sub Main, save the code and run it. |
Click here to copy the following block | Option Explicit
Public Const CLASS_NAME As String = "VB_WIN" Public Const APP_TITLE As String = "API Window from VB"
Public Const WS_CAPTION = &HC00000 Public Const WS_MAXIMIZEBOX = &H10000 Public Const WS_MINIMIZEBOX = &H20000 Public Const WS_OVERLAPPED = &H0& Public Const WS_SYSMENU = &H80000 Public Const WS_THICKFRAME = &H40000 Public Const WS_OVERLAPPEDWINDOW = (WS_OVERLAPPED Or WS_CAPTION _ Or WS_SYSMENU Or WS_THICKFRAME Or _ WS_MINIMIZEBOX Or WS_MAXIMIZEBOX) Public Const CS_HREDRAW = &H2 Public Const CS_VREDRAW = &H1 Public Const IDI_APPLICATION = 32512& Public Const IDC_ARROW = 32512& Public Const LTGRAY_BRUSH = 1 Public Const SW_SHOWNORMAL = 1
Public Const WM_CREATE = &H1 Public Const WM_CLOSE = &H10 Public Const WM_PAINT = &HF Public Const WM_KEYDOWN = &H100 Public Const WM_KEYUP = &H101 Public Const WM_CHAR = &H102 Public Const WM_DESTROY = &H2 Public Const WM_LBUTTONDOWN = &H201 Public Const WM_RBUTTONDOWN = &H204 Public Const MB_OK = &H0&
Public Type WNDCLASSEX cbSize As Long style As Long lpfnWndProc As Long cbClsExtra As Long cbWndExtra As Long hInstance As Long hIcon As Long hCursor As Long hbrBackground As Long lpszMenuName As String lpszClassName As String hIconSm As Long End Type
Type POINTAPI x As Long y As Long End Type
Type MSG hWnd As Long Message As Long wParam As Long lParam As Long time As Long pt As POINTAPI End Type
Declare Function LoadCursor Lib "user32" Alias "LoadCursorA" _ (ByVal hInstance As Long, _ ByVal lpCursorName As String) As Long
Declare Function LoadIcon Lib "user32" Alias "LoadIconA" _ (ByVal hInstance As Long, _ ByVal lpIconName As String) As Long
Declare Function RegisterClassEx Lib "user32" Alias "RegisterClassExA" _ (pcWndClassEx As WNDCLASSEX) As Long
Declare Function CreateWindowEx Lib "user32" Alias "CreateWindowExA" _ (ByVal dwExStyle As Long, _ ByVal lpClassName As String, _ ByVal lpWindowName As String, _ ByVal dwStyle As Long, _ ByVal x As Long, _ ByVal y As Long, _ ByVal nWidth As Long, _ ByVal nHeight As Long, _ ByVal hWndParent As Long, _ ByVal hMenu As Long, _ ByVal hInstance As Long, _ lpParam As Any) As Long
Declare Function ShowWindow Lib "user32" _ (ByVal hWnd As Long, _ ByVal nCmdShow As Long) As Long
Declare Function UpdateWindow Lib "user32" _ (ByVal hWnd As Long) As Long
Declare Function GetMessage Lib "user32" Alias "GetMessageA" _ (lpMsg As MSG, _ ByVal hWnd As Long, _ ByVal wMsgFilterMin As Long, _ ByVal wMsgFilterMax As Long) As Long
Declare Function TranslateMessage Lib "user32" _ (lpMsg As MSG) As Long
Declare Function DispatchMessage Lib "user32" Alias "DispatchMessageA" _ (lpMsg As MSG) As Long
Declare Sub PostQuitMessage Lib "user32" _ (ByVal nExitCode As Long)
Declare Function GetStockObject Lib "gdi32" _ (ByVal nIndex As Long) As Long
Declare Function MessageBox Lib "user32" Alias "MessageBoxA" _ (ByVal hWnd As Long, _ ByVal lpText As String, _ ByVal lpCaption As String, _ ByVal wType As Long) As Long
Declare Function SetFocus Lib "user32" (ByVal hWnd As Long) As Long
Declare Function DefWindowProc Lib "user32" Alias "DefWindowProcA" _ (ByVal hWnd As Long, _ ByVal wMsg As Long, _ ByVal wParam As Long, _ ByVal lParam As Long) As Long
Declare Function PostMessage Lib "user32" Alias "PostMessageA" _ (ByVal hWnd As Long, _ ByVal wMsg As Long, _ ByVal wParam As Long, _ ByVal lParam As Long) As Long
Public Sub Main() WinMain End Sub
Public Function WinMain() As Long Dim wndClass As WNDCLASSEX Dim hWndMain As Long Dim Message As MSG
With wndClass .cbSize = Len(wndClass) .style = CS_HREDRAW Or CS_VREDRAW .lpfnWndProc = GetFunctionPtr(AddressOf WndProc) .cbClsExtra = 0 .cbWndExtra = 0 .hInstance = App.hInstance .hIcon = LoadIcon(App.hInstance, IDI_APPLICATION) .hCursor = LoadCursor(App.hInstance, IDC_ARROW) .hbrBackground = GetStockObject(LTGRAY_BRUSH) .lpszMenuName = vbNullString .lpszClassName = CLASS_NAME .hIconSm = LoadIcon(App.hInstance, IDI_APPLICATION) End With
RegisterClassEx wndClass
hWndMain = CreateWindowEx(0&, CLASS_NAME, APP_TITLE, _ WS_OVERLAPPEDWINDOW, 0&, 0&, 640&, 480&, 0&, 0&, _ App.hInstance, 0&)
ShowWindow hWndMain, SW_SHOWNORMAL UpdateWindow hWndMain SetFocus hWndMain
Do While 0 <> (GetMessage(Message, 0&, 0&, 0&)) TranslateMessage Message DispatchMessage Message Loop
WinMain = Message.wParam End Function
Public Function WndProc(ByVal hWnd As Long, ByVal Message As Long, _ ByVal wParam As Long, ByVal lParam As Long) As Long Select Case Message
Case WM_CREATE Case WM_CHAR If wParam = vbKeyEscape Then Call PostMessage(hWnd, WM_CLOSE, 0, 0) Else WndProc = DefWindowProc(hWnd, Message, wParam, lParam) End If Case WM_PAINT Case WM_LBUTTONDOWN MessageBox hWnd, "Left Mouse Button Pressed", APP_TITLE, MB_OK Exit Function Case WM_RBUTTONDOWN MessageBox hWnd, "Right Mouse Button Pressed", APP_TITLE, MB_OK Exit Function Case WM_DESTROY PostQuitMessage 0& Exit Function Case Else WndProc = DefWindowProc(hWnd, Message, wParam, lParam) End Select End Function
Public Function GetFunctionPtr(ByVal Func As Long) As Long GetFunctionPtr = Func End Function |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
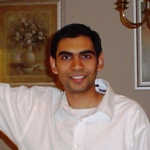
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|