|
|
|
This article demonstrates how to use a set of related Component Object Model (COM) APIs that retrieve and manipulate CLSIDs and ProgIDs. The following APIs are discussed:
Step-By-Step Example
- Create a new ActiveX DLL project (Project1) in Visual Basic (Class1 is created by default). - Compile the default project. Now, you have a test component: Project1.dll, which contains a COM class (Project1.Class1) - Create a new Standard EXE project (Project1) in Visual Basic. Form1 is created by default. - Add three TextBoxes, one commandbutton and one ListBox to Form1. - Add the following code to Form1's code module: |
Click here to copy the following block | Option Explicit
Private Type GUID Data1 As Long Data2 As Integer Data3 As Integer Data4(7) As Byte End Type
Private Declare Function CLSIDFromProgID _ Lib "ole32.dll" (ByVal lpszProgID As Long, _ pCLSID As GUID) As Long
Private Declare Function ProgIDFromCLSID _ Lib "ole32.dll" (pCLSID As GUID, lpszProgID As Long) As Long
Private Declare Function StringFromCLSID _ Lib "ole32.dll" (pCLSID As GUID, lpszProgID As Long) As Long
Private Declare Function CLSIDFromString _ Lib "ole32.dll" (ByVal lpszProgID As Long, _ pCLSID As GUID) As Long
Private Declare Sub CopyMemory Lib "kernel32" Alias _ "RtlMoveMemory" (pDst As Any, pSrc As Any, ByVal ByteLen As Long)
Private Sub GetInfo() Dim strProgID As String * 255 Dim pProgID As Long Dim udtCLSID As GUID Dim strCLSID As String * 255 Dim pCLSID As Long Dim lngRet As Long Dim strTemp As String Dim i As Integer
strTemp = Text1.Text
lngRet = CLSIDFromProgID(StrPtr(strTemp), udtCLSID)
With List1 .AddItem Hex(udtCLSID.Data1) .AddItem Hex(udtCLSID.Data2) .AddItem Hex(udtCLSID.Data3) For i = 0 To 7 .AddItem Hex(udtCLSID.Data4(i)) Next End With
lngRet = StringFromCLSID(udtCLSID, pCLSID)
StringFromPointer pCLSID, strCLSID Text2.Text = strCLSID
With udtCLSID .Data1 = 0 .Data2 = 0 .Data3 = 0 For i = 0 To 7 .Data4(i) = 0 Next End With
strTemp = Text2.Text lngRet = CLSIDFromString(StrPtr(strTemp), udtCLSID)
lngRet = ProgIDFromCLSID(udtCLSID, pProgID)
StringFromPointer pProgID, strProgID Text3.Text = strProgID
End Sub
Private Sub StringFromPointer(pOLESTR As Long, strOut As String) Dim ByteArray(255) As Byte Dim intTemp As Integer Dim intCount As Integer Dim i As Integer
intTemp = 1
While intTemp <> 0 CopyMemory intTemp, ByVal pOLESTR + i, 2 ByteArray(intCount) = intTemp intCount = intCount + 1 i = i + 2 Wend
CopyMemory ByVal strOut, ByteArray(0), intCount End Sub
Private Sub Command1_Click() GetInfo End Sub
Private Sub Form_Load() Text1.Text = "Project1.Class1" GetInfo End Sub |
- Run the project, and press Command1. The following appears:
Text1: Displays the ProgID of the component that you want to get the CLSID from. Text2: Displays the CLSID retrieved in string format. Test3: Displays the ProgID retrieved based on the CLSID. List1: Displays the members of the CLSID structure in hex format. |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
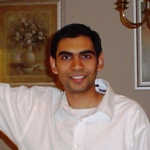
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|