|
|
|
The Win32 API provides two sets of APIs for working with security descriptors (SDs) and access control lists (ACLs). Low-level as well as high-level access control API sets provide an interface for working with SDs and ACLs. For Microsoft Windows 2000 and MIcrosoft Windows XP, the high-level access control APIs have been enhanced to support object-specific access control entries (ACEs), directory service (DS) objects, and automatic inheritance. This article provides sample code that uses high-level access control APIs to modify an existing discretionary ACL (DACL) on a folder securable object.
The following Visual Basic sample code uses the GetNamedSecurityInfo() API to retrieve the existing DACL of the C:\Test1 folder. Then, it builds the EXPLICIT_ACCESS structure for everyone trustee with GENERIC_READ access by using the BuildExplicitAccessWithName() API. In this example, the ACE is constructed with inheritance CONTAINER_INHERIT_ACE or OBJECT_INHERIT_ACE, which applies as an effective ACE for the C:\Test1 folder and an inheritable ACE for subfolders as well as files.
The SetEntriesInAcl() API creates a new access-control list (ACL) by merging new access-control information specified in EXPLICIT_ACCESS structures into an existing ACL and returns a newly updated DACL in memory. This updated DACL is then used to set security on the C:\Test1 folder by using the SetNamedSecurityInfo() API.
Step-By-Step Example - Create a standard exe project - Add one command button the the form1 - Copy/Paste the following code in form1's code window |
Click here to copy the following block | Option Explicit
Private Const ERROR_SUCCESS = 0&
Private Const SE_FILE_OBJECT = 1&
Private Const DACL_SECURITY_INFORMATION = 4& Private Const SET_ACCESS = 2&
Private Const SYNCHRONIZE = &H100000 Private Const READ_CONTROL = &H20000 Private Const WRITE_DAC = &H40000 Private Const WRITE_OWNER = &H80000 Private Const STANDARD_RIGHTS_READ = (READ_CONTROL) Private Const STANDARD_RIGHTS_WRITE = (READ_CONTROL) Private Const DELETE = &H10000
Private Const GENERIC_ALL = &H10000000 Private Const GENERIC_EXECUTE = &H20000000 Private Const GENERIC_READ = &H80000000 Private Const GENERIC_WRITE = &H40000000
Private Const CONTAINER_INHERIT_ACE = &H2 Private Const OBJECT_INHERIT_ACE = &H1
Private Type TRUSTEE pMultipleTrustee As Long MultipleTrusteeOperation As Long TrusteeForm As Long TrusteeType As Long ptstrName As String End Type
Private Type EXPLICIT_ACCESS grfAccessPermissions As Long grfAccessMode As Long grfInheritance As Long pTRUSTEE As TRUSTEE End Type
Private Declare Sub BuildExplicitAccessWithName Lib "Advapi32.dll" Alias _ "BuildExplicitAccessWithNameA" _ (ea As Any, _ ByVal TrusteeName As String, _ ByVal AccessPermissions As Long, _ ByVal AccessMode As Integer, _ ByVal Inheritance As Long)
Private Declare Function SetEntriesInAcl Lib "Advapi32.dll" Alias _ "SetEntriesInAclA" _ (ByVal CountofExplicitEntries As Long, _ ea As Any, _ ByVal OldAcl As Long, _ NewAcl As Long) As Long
Private Declare Function GetNamedSecurityInfo Lib "Advapi32.dll" Alias _ "GetNamedSecurityInfoA" _ (ByVal ObjName As String, _ ByVal SE_OBJECT_TYPE As Long, _ ByVal SecInfo As Long, _ ByVal pSid As Long, _ ByVal pSidGroup As Long, _ pDacl As Long, _ ByVal pSacl As Long, _ pSecurityDescriptor As Long) As Long
Private Declare Function SetNamedSecurityInfo Lib "Advapi32.dll" Alias _ "SetNamedSecurityInfoA" _ (ByVal ObjName As String, _ ByVal SE_OBJECT As Long, _ ByVal SecInfo As Long, _ ByVal pSid As Long, _ ByVal pSidGroup As Long, _ ByVal pDacl As Long, _ ByVal pSacl As Long) As Long
Private Declare Function LocalFree Lib "kernel32" (ByVal hMem As Long) As Long
Private Sub Command1_Click()
Dim result As Long Dim pSecDesc As Long Dim ea As EXPLICIT_ACCESS Dim pNewDACL As Long Dim pOldDACL As Long
result = GetNamedSecurityInfo("c:\test1", _ SE_FILE_OBJECT, _ DACL_SECURITY_INFORMATION, _ 0&, _ 0&, _ pOldDACL, _ 0&, _ pSecDesc)
If result = ERROR_SUCCESS Then
Call BuildExplicitAccessWithName(ea, _ "Users", _ GENERIC_READ, _ SET_ACCESS, _ CONTAINER_INHERIT_ACE Or OBJECT_INHERIT_ACE)
result = SetEntriesInAcl(1, ea, pOldDACL, pNewDACL)
If result = ERROR_SUCCESS Then MsgBox "SetEntriesInAcl succeeded"
result = SetNamedSecurityInfo("c:\test1", _ SE_FILE_OBJECT, _ DACL_SECURITY_INFORMATION, _ 0&, _ 0&, _ pNewDACL, _ 0&)
If result = ERROR_SUCCESS Then MsgBox "SetNamedSecurityInfo succeeded" Else MsgBox "SetNamedSecurityInfo failed with error code : " & result End If
LocalFree pNewDACL Else MsgBox "SetEntriesInAcl failed with error code : " & result End If
LocalFree pSecDesc Else MsgBox "GetNamedSecurityInfo failed with error code : " & result End If End Sub |
- Before running demo make sure you create a folder c:\test1 - Press F5 to Run - Now if you observe on c:\Test1 Properties->Security tab for All users we got explicit read permission.
Before Demo
After Demo |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
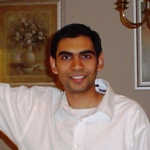
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|