|
|
|
In this article we will explore 3 APIs (Arc, ArcTo and AngleArc).
An arc is a portion or segment of an ellipse, meaning an arc is a non-complete ellipse. Because an arc must confirm to the shape of an ellipse, it is defined as it fits in a rectangle and can be illustrated as follows:
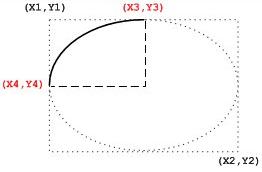
Arc
To draw an arc, you can use the Arc() function whose syntax is: |
Click here to copy the following block | Declare Function Arc Lib "gdi32.dll" ( _ ByVal hdc As Long, _ ByVal nLeftRect As Long, _ ByVal nTopRect As Long, _ ByVal nRightRect As Long, _ ByVal nBottomRect As Long, _ ByVal nXStartArc As Long, _ ByVal nYStartArc As Long, _ ByVal nXEndArc As Long, _ ByVal nXEndArc As Long) As Long |
Besides the left (nLeftRect, nTopRect) and the right (nRightRect, nBottomRect) borders of the rectangle in which the arc would fit, an arc must specify where it starts and where it ends. These additional points are set as the (nXStartArc, nYStartArc) and (nXEndArc, nYEndArc) points of the figure.
ArcTo
Besides the Arc() function, the GDI library provides the ArcTo() member function used to draw an arc. Its syntaxes is: |
Click here to copy the following block | Declare Function ArcTo Lib "gdi32.dll" ( _ ByVal hdc As Long, _ ByVal nLeftRect As Long, _ ByVal nTopRect As Long, _ ByVal nRightRect As Long, _ ByVal nBottomRect As Long, _ ByVal nXStartArc As Long, _ ByVal nYStartArc As Long, _ ByVal nXEndArc As Long, _ ByVal nXEndArc As Long) As Long |
This function uses the same arguments as Arc(). The difference is that while Arc() starts drawing at (nXRadial1, nYRadial1), ArcTo() does not inherently control the drawing starting point. It refers to the current point, exactly like the LineTo() (and the PolylineTo()) function. Therefore, if you want to specify where the drawing should start, can call MoveToEx() before ArcTo().
To control this orientation, the GDI library has SetArcDirection() and GetArcDirection function. Its syntax is: |
This function specifies the direction the Arc() function should follow from the starting to the end points. The argument passed as ArcDirection controls this orientation. It can have the following values: | AD_CLOCKWISE:ThefigureisdrawnclockwiseAD_COUNTERCLOCKWISE:ThefigureisdrawncounterclockwiseThisisdefault AngleArc
AngleArc function draws a line and an arc connected. The arc is based on a circle and not an ellipse. This implies that the arc fits inside a square and not a rectangle. The circle that would be the base of the arc is defined by its center located at C(X, Y) with a radius of dwRadius. The arc starts at an angle of eStartAngle. The angle is based on the x axis and must be positive. That is, it must range from 0° to 360°. If you want to specify an angle that is below the x axis, such as -15°, use 360º-15°=345°. The last argument, eSweepAngle, is the angular area covered by the arc.
Here is the syntax for AngleArc function : |
Click here to copy the following block | Declare Function AngleArc Lib "gdi32" _ (ByVal hdc As Long, _ ByVal xCenter As Long, _ ByVal yCenter As Long, _ ByVal dwRadius As Long, _ ByVal eStartAngle As Single, _ ByVal eSweepAngle As Single) As Long |
Step-By-Step Example
- Create a standard exe project - Add 3 buttons and one picturebox on form1 - Add the following code in form1 code window |
Click here to copy the following block | Const AD_CLOCKWISE = 2 Const AD_COUNTERCLOCKWISE = 1
Private Declare Function Arc Lib "gdi32" _ (ByVal hdc As Long, _ ByVal X1 As Long, _ ByVal Y1 As Long, _ ByVal X2 As Long, _ ByVal Y2 As Long, _ ByVal X3 As Long, _ ByVal Y3 As Long, _ ByVal X4 As Long, _ ByVal Y4 As Long) As Long
Private Declare Function ArcTo Lib "gdi32" _ (ByVal hdc As Long, _ ByVal X1 As Long, _ ByVal Y1 As Long, _ ByVal X2 As Long, _ ByVal Y2 As Long, _ ByVal X3 As Long, _ ByVal Y3 As Long, _ ByVal X4 As Long, _ ByVal Y4 As Long) As Long
Private Declare Function AngleArc Lib "gdi32" _ (ByVal hdc As Long, _ ByVal x As Long, _ ByVal y As Long, _ ByVal dwRadius As Long, _ ByVal eStartAngle As Single, _ ByVal eSweepAngle As Single) As Long
Private Declare Function MoveToEx Lib "gdi32" _ (ByVal hdc As Long, _ ByVal x As Long, _ ByVal y As Long, _ lpPoint As Any) As Long
Private Declare Function SetArcDirection Lib "gdi32" _ (ByVal hdc As Long, _ ByVal ArcDirection As Long) As Long
Private Declare Function GetArcDirection Lib "gdi32" _ (ByVal hdc As Long) As Long
Dim i As Integer Dim flag As Integer
Private Sub Command1_Click() Picture1.Cls
Picture1.ForeColor = vbRed retval = Arc(Picture1.hdc, 0, 0, 200, 100, 0, 50, 100, 100)
Picture1.ForeColor = vbBlue retval = Arc(Picture1.hdc, 0, 0, 200, 100, 100, 100, 200, 50)
Picture1.ForeColor = vbMagenta retval = Arc(Picture1.hdc, 0, 0, 200, 100, 200, 50, 100, 0)
Picture1.ForeColor = vbGreen retval = Arc(Picture1.hdc, 0, 0, 200, 100, 100, 0, 0, 50) End Sub
Private Sub Command2_Click() Picture1.Cls
Picture1.Line (0, 0)-(200, 100), , B
If GetArcDirection(Picture1.hdc) = AD_CLOCKWISE Then SetArcDirection Picture1.hdc, AD_COUNTERCLOCKWISE End If
Picture1.ForeColor = vbRed MoveToEx Picture1.hdc, 100, 50, ByVal 0& AngleArc Picture1.hdc, 100, 50, 50, 0, 45
Picture1.ForeColor = vbBlue MoveToEx Picture1.hdc, 100, 50, ByVal 0& AngleArc Picture1.hdc, 100, 50, 50, 180, -45
End Sub
Private Sub Command3_Click() Picture1.Cls MoveToEx Picture1.hdc, 100, 0, ByVal 0&
Picture1.ForeColor = vbRed retval = ArcTo(Picture1.hdc, 0, 0, 200, 100, 0, 50, 100, 100)
Picture1.ForeColor = vbBlue retval = ArcTo(Picture1.hdc, 0, 0, 200, 100, 200, 50, 100, 0) End Sub Private Sub Form_Load() Picture1.BackColor = vbWhite Command1.Caption = "Arc" Command2.Caption = "AngleArc" Command3.Caption = "ArcTo"
Picture1.AutoRedraw = True Picture1.ScaleMode = vbPixels Picture1.Line (0, 0)-(200, 100), , B End Sub |
- Press F5 to run the project - Click on the different buttons to see the curves generated by various Arc APIs |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
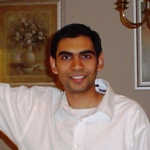
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|