|
|
|
This article will show you step by step approch to Add and Delete Printer Programatically without any user interaction.
When writing a Win32 application that installs a network printer connection, you must take into consideration differences in printing if the application will be run under both Windows 95 and Windows NT. One important difference is that Windows NT may download appropriate drivers from the print server while under Windows 95, you need to add the printer driver files to the system with the AddPrinterDriver function.
This process should take two separate code paths depending on whether you are running Windows NT or Windows 95. In Windows 95, you will want to add the printer driver with AddPrinterDriver and add the printer connection with AddPrinter.
In Windows NT, you need only call the AddPrinterConnection function as below |
Now we will see example for How to install a printer assuming that you have driver installed.
Step-By-Step Example
- Create a standard exe project - Add module1 - Add 4 text box and 2 command button on the Form1
Place the following code in Form1 code window
Form1.frm |
Click here to copy the following block | Private Sub Command1_Click() AddNewPrinter Text1, Text2, Text3, Text4 End Sub
Private Sub Command2_Click() Call DeleteExistingPrinter(PRINTER.DeviceName) End Sub
Private Sub Form_Load() Text1.Text = "My_HP_Test_Printer" Text2.Text = "HP LaserJet 2000" Text3.Text = "LPT1:" Text4.Text = "" Command1.Caption = "Add Printer" Command2.Caption = "Delete Printer" End Sub |
Place the following code in Module1 code window
Module1.bas |
Click here to copy the following block | Public Const CCHDEVICENAME = 32
Public Const CCHFORMNAME = 32
Public Const PRINTER_ATTRIBUTE_SHARED As Long = &H8 Public Const PRINTER_ATTRIBUTE_LOCAL As Long = &H40 Public Const PRINTER_ATTRIBUTE_NETWORK = &H10 Public Const PRINTER_LEVEL2 As Long = &H2
Public Const STANDARD_RIGHTS_REQUIRED As Long = &HF0000 Public Const PRINTER_ACCESS_ADMINISTER As Long = &H4 Public Const PRINTER_ACCESS_USE As Long = &H8 Public Const PRINTER_ALL_ACCESS As Long = (STANDARD_RIGHTS_REQUIRED Or _ PRINTER_ACCESS_ADMINISTER Or _ PRINTER_ACCESS_USE)
Type DEVMODE dmDeviceName As String * CCHDEVICENAME dmSpecVersion As Integer dmDriverVersion As Integer dmSize As Integer dmDriverExtra As Integer dmFields As Long dmOrientation As Integer dmPaperSize As Integer dmPaperLength As Integer dmPaperWidth As Integer dmScale As Integer dmCopies As Integer dmDefaultSource As Integer dmPrintQuality As Integer dmColor As Integer dmDuplex As Integer dmYResolution As Integer dmTTOption As Integer dmCollate As Integer dmFormName As String * CCHFORMNAME dmUnusedPadding As Integer dmBitsPerPel As Integer dmPelsWidth As Long dmPelsHeight As Long dmDisplayFlags As Long dmDisplayFrequency As Long End Type
Type PRINTER_DEFAULTS pDatatype As String pDevMode As Long DesiredAccess As Long End Type
Type PRINTER_INFO_2 pServerName As String pPrinterName As String pShareName As String pPortName As String pDriverName As String pComment As String pLocation As String pDevMode As Long pSepFile As String pPrintProcessor As String pDatatype As String pParameters As String pSecurityDescriptor As Long Attributes As Long Priority As Long DefaultPriority As Long StartTime As Long UntilTime As Long Status As Long cJobs As Long AveragePPM As Long End Type
Type DRIVER_INFO_2 cVersion As Long pName As String pEnvironment As String pDriverPath As String pDataFile As String pConfigFile As String End Type
Declare Function OpenPrinter Lib "winspool.drv" Alias "OpenPrinterA" ( _ ByVal pPrinterName As String, _ phPrinter As Long, _ pDefault As PRINTER_DEFAULTS) As Long
Declare Function AddPrinter Lib "winspool.drv" Alias "AddPrinterA" ( _ ByVal pName As String, _ ByVal Level As Long, _ pPrinter As Any) As Long
Declare Function DeletePrinter Lib "winspool.drv" ( _ ByVal hPrinter As Long) As Long
Declare Function ClosePrinter Lib "winspool.drv" ( _ ByVal hPrinter As Long) As Long
Const FORMAT_MESSAGE_ALLOCATE_BUFFER = &H100 Const FORMAT_MESSAGE_FROM_SYSTEM = &H1000 Const LANG_NEUTRAL = &H0 Const SUBLANG_DEFAULT = &H1 Const ERROR_ACCESS_DENIED = 5&
Private Declare Function GetLastError Lib "kernel32" () As Long Private Declare Sub SetLastError Lib "kernel32" (ByVal dwErrCode As Long) Private Declare Function FormatMessage Lib "kernel32" Alias "FormatMessageA" (ByVal dwFlags As Long, lpSource As Any, ByVal dwMessageId As Long, ByVal dwLanguageId As Long, ByVal lpBuffer As String, ByVal nSize As Long, Arguments As Long) As Long
Function GetAPIErrorDesc(ErrorCode As Long) As String Dim Buffer As String Buffer = Space(200) FormatMessage FORMAT_MESSAGE_FROM_SYSTEM, ByVal 0&, ErrorCode, LANG_NEUTRAL, Buffer, 200, ByVal 0& GetAPIErrorDesc = Buffer End Function
Public Sub AddNewPrinter(ByVal PrinterName As String, ByVal Driver As String, _ Optional ByVal Port As String = "LPT1:", Optional Server As String, _ Optional Comment As String, Optional PhysicalLocation As String, _ Optional ShareName As String)
Dim hPrint As Long, PI As PRINTER_INFO_2
With PI .pServerName = Server .pPrinterName = PrinterName .pDriverName = Driver .pPortName = Port .pPrintProcessor = "WinPrint" .Priority = 1 .DefaultPriority = 1 .pComment = Comment .pDatatype = "RAW" .pLocation = PhysicalLocation .pShareName = ShareName End With
hPrint = AddPrinter(Server, 2, PI)
If hPrint = 0 Then MsgBox "Error :" & GetAPIErrorDesc(Err.LastDllError)
ClosePrinter hPrint
End Sub
Sub DeleteExistingPrinter(PrinterNameToDelete As String) Dim hPrinter As Long Dim sPrinterName As String Dim pd As PRINTER_DEFAULTS
sPrinterName = PrinterNameToDelete
With pd .pDevMode = 0&
.pDatatype = "RAW"
.DesiredAccess = PRINTER_ALL_ACCESS End With
If OpenPrinter(sPrinterName, hPrinter, pd) <> 0 Then
If hPrinter <> 0 Then If DeletePrinter(hPrinter) <> 0 Then MsgBox "Printer deleted" Else MsgBox "Error :" & GetAPIErrorDesc(Err.LastDllError) End If End If ClosePrinter hPrinter Else MsgBox "Error :" & GetAPIErrorDesc(Err.LastDllError) End If End Sub |
Now Press F5 to run the demo.
After you click on Add Printer new printer will be installed and you can see in Printers folder as shown below
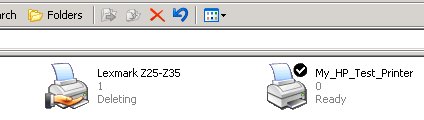
|
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
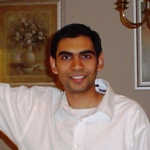
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|