|
|
|
In previous Article Working with AVI Files - Part 1 (Opening and Closing AVI file) We learned how to open and close AVI File. We also learned How to read AVI file information using AVIFileInfo api. In this article we will go one step further. Today we will work with AVI Streams.
Avi file can contain one or more than one streams. Stream is chunk of data. In our project we have defined 4 types of streams |
Click here to copy the following block | Global Const streamtypeVIDEO As Long = 1935960438 Global Const streamtypeAUDIO As Long = 1935963489 Global Const streamtypeMIDI As Long = 1935960429 Global Const streamtypeTEXT As Long = 1937012852 |
Parameters :
pfile [in] Handle to an open AVI file.
ppavi [in, out] Pointer to the new stream interface.
fccType [in] Four-character code indicating the type of stream to open. Zero indicates any stream can be opened. The following definitions apply to the data commonly found in AVI streams.
- streamtypeAUDIO : Indicates an audio stream.
- streamtypeMIDI : Indicates a MIDI stream.
- streamtypeTEXT : Indicates a text stream.
- streamtypeVIDEO : Indicates a video stream.
lParam [in] Count of the stream type. Identifies which occurrence of the specified stream type to access.
Return values :
Returns zero if successful or an error otherwise. Possible error values include the following.
- AVIERR_NODATA : The file does not contain a stream corresponding to the values of fccType and lParam.
- AVIERR_MEMORY : Not enough memory.
Our Sample call may look like as below |
Once we get handle to the required stream you can perform various operations on that stream. Here in our sample code we retrive Number of frames in the requested stream and startting position of that stream (Most of the time it will be 0 but stream may start anywhere in the avi file)
To Get Number of frames in the requested stream call AVIStreamLength with stream handle as an input parameter
To Get start position of stream in the avi file call AVIStreamStart with stream handle as an input parameter
To Get detail stream information you may call AVIStreamInfo function. |
Parameters :
pavi [in] Handle to an open stream.
psi [in, out] Pointer to a structure to contain the stream information.
lSize [in] Size, in bytes, of the structure used for psi.
Return Values
Returns zero if successful or an error otherwise.
And here is the full implementation of our example.
Step-By-Step Example
- Create a standard exe project - Place one command button - Place 2 text box. Set MultiLine=True and Scrollbar=both for text2 and text3 - Run the project - Specify valid AVI path and click on Command button
Place following code in Form1
Form1.frm |
Click here to copy the following block | Private Sub Command1_Click() On Error GoTo errHandler
Dim res As Long Dim hFile As Long Dim hStream As Long
Dim numFrames As Long Dim firstFrame As Long
Dim fileInfo As AVI_FILE_INFO Dim streamInfo As AVI_STREAM_INFO
Call AVIFileInit If AVIFileOpen(hFile, Text1, OF_SHARE_DENY_WRITE, ByVal 0&) = 0 Then
res = AVIFileGetStream(hFile, hStream, streamtypeVIDEO, 0) If res <> AVIERR_OK Then MsgBox "Error while retrieving VIDEO stream information... " & GetAPIErrorDesc(Err.LastDllError) GoTo errHandler End If
firstFrame = AVIStreamStart(hStream)
If firstFrame = -1 Then MsgBox "Error while retrieving the start position of the stream... " & GetAPIErrorDesc(Err.LastDllError) GoTo errHandler End If
numFrames = AVIStreamLength(hStream) If numFrames = -1 Then MsgBox "Error while retrieving the length of the stream in frames... " & GetAPIErrorDesc(Err.LastDllError) GoTo errHandler End If
Text2 = Text2 & "Stream Handle : " & hStream & vbCrLf Text2 = Text2 & "Video stream length : " & numFrames & " (frames)" & vbCrLf Text2 = Text2 & "Stream start on frame : " & firstFrame & vbCrLf
If AVIStreamInfo(hStream, streamInfo, Len(streamInfo)) = 0 Then Call PrintAVIStreamInfo(streamInfo, Text3) Else MsgBox "Error while retrieving AVI stream information... " & GetAPIErrorDesc(Err.LastDllError) End If Else MsgBox "Error while opening the AVI file... " & GetAPIErrorDesc(Err.LastDllError) End If
errHandler: If hFile <> 0 Then If hStream <> 0 Then Call AVIStreamRelease(hStream)
Call AVIFileRelease(hFile)
Call AVIFileExit End If End Sub
Private Sub Form_Load()
If Len(App.Path) > 3 Then Text1 = App.Path & "\" & "test.avi" Else Text1 = App.Path & "test.avi" End If End Sub |
Place following code in the module
Module1.bas |
Click here to copy the following block |
Global Const FORMAT_MESSAGE_ALLOCATE_BUFFER = &H100 Global Const FORMAT_MESSAGE_FROM_SYSTEM = &H1000 Global Const LANG_NEUTRAL = &H0 Global Const SUBLANG_DEFAULT = &H1
Public Declare Function GetLastError Lib "kernel32" () As Long Public Declare Sub SetLastError Lib "kernel32" (ByVal dwErrCode As Long) Public Declare Function FormatMessage Lib "kernel32" Alias "FormatMessageA" (ByVal dwFlags As Long, lpSource As Any, ByVal dwMessageId As Long, ByVal dwLanguageId As Long, ByVal lpBuffer As String, ByVal nSize As Long, Arguments As Long) As Long
Public Declare Function AVIFileInfo Lib "avifil32.dll" _ (ByVal pfile As Long, _ pfi As AVI_FILE_INFO, _ ByVal lSize As Long) As Long
Public Declare Sub AVIFileInit Lib "avifil32.dll" ()
Public Declare Function AVIFileOpen Lib "avifil32.dll" _ (ByRef ppfile As Long, _ ByVal szFile As String, _ ByVal uMode As Long, _ ByVal pclsidHandler As Long) As Long
Public Declare Function AVIFileRelease Lib "avifil32.dll" _ (ByVal pfile As Long) As Long
Public Declare Sub AVIFileExit Lib "avifil32.dll" ()
Public Declare Function AVIFileGetStream Lib "avifil32.dll" _ (ByVal pfile As Long, _ ByRef ppaviStream As Long, _ ByVal fccType As Long, _ ByVal lParam As Long) As Long
Public Declare Function AVIStreamInfo Lib "avifil32.dll" _ (ByVal pAVIStream As Long, _ ByRef psi As AVI_STREAM_INFO, _ ByVal lSize As Long) As Long
Public Declare Function AVIStreamStart Lib "avifil32.dll" _ (ByVal pavi As Long) As Long
Public Declare Function AVIStreamLength Lib "avifil32.dll" _ (ByVal pavi As Long) As Long
Public Declare Function AVIStreamRelease Lib "avifil32.dll" _ (ByVal pavi As Long) As Long
Public Type AVI_STREAM_INFO fccType As Long fccHandler As Long dwFlags As Long dwCaps As Long wPriority As Integer wLanguage As Integer dwScale As Long dwRate As Long dwStart As Long dwLength As Long dwInitialFrames As Long dwSuggestedBufferSize As Long dwQuality As Long dwSampleSize As Long rcFrame As AVI_RECT dwEditCount As Long dwFormatChangeCount As Long szName As String * 64 End Type
Global Const OF_CREATE = &H1000 Global Const OF_READ = &H0 Global Const OF_READWRITE = &H2 Global Const OF_SHARE_DENY_WRITE = &H20 Global Const OF_WRITE = &H1
Global Const streamtypeVIDEO As Long = 1935960438 Global Const streamtypeAUDIO As Long = 1935963489 Global Const streamtypeMIDI As Long = 1935960429 Global Const streamtypeTEXT As Long = 1937012852
Public Sub PrintAVIStreamInfo(asi As AVI_STREAM_INFO, txt As TextBox) txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & "**** AVI_STREAM_INFO (START) *****" txt = txt & vbCrLf & "**********************************" With asi txt = txt & vbCrLf & "fccType = " & .fccType txt = txt & vbCrLf & "fccHandler = " & .fccHandler txt = txt & vbCrLf & "dwFlags = " & .dwFlags txt = txt & vbCrLf & "dwCaps = " & .dwCaps txt = txt & vbCrLf & "wPriority = " & .wPriority txt = txt & vbCrLf & "wLanguage = " & .wLanguage txt = txt & vbCrLf & "dwScale = " & .dwScale txt = txt & vbCrLf & "dwRate = " & .dwRate txt = txt & vbCrLf & "dwStart = " & .dwStart txt = txt & vbCrLf & "dwLength = " & .dwLength txt = txt & vbCrLf & "dwInitialFrames = " & .dwInitialFrames txt = txt & vbCrLf & "dwSuggestedBufferSize = " & .dwSuggestedBufferSize txt = txt & vbCrLf & "dwQuality = " & .dwQuality txt = txt & vbCrLf & "dwSampleSize = " & .dwSampleSize txt = txt & vbCrLf & "rcFrame.left = " & .rcFrame.left txt = txt & vbCrLf & "rcFrame.top = " & .rcFrame.top txt = txt & vbCrLf & "rcFrame.right = " & .rcFrame.right txt = txt & vbCrLf & "rcFrame.bottom = " & .rcFrame.bottom txt = txt & vbCrLf & "dwEditCount = " & .dwEditCount txt = txt & vbCrLf & "dwFormatChangeCount = " & .dwFormatChangeCount txt = txt & vbCrLf & "szName = " & .szName End With txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & "**** AVI_STREAM_INFO (END) *******" txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & ""
If TypeOf txt Is TextBox Then Else Debug.Print txt End If
End Sub
Public Function GetAPIErrorDesc(ErrorCode As Long) As String Dim Buffer As String Buffer = Space(200) FormatMessage FORMAT_MESSAGE_FROM_SYSTEM, ByVal 0&, ErrorCode, LANG_NEUTRAL, Buffer, 200, ByVal 0& GetAPIErrorDesc = Buffer End Function |
|
|
|
Submitted By :
Jojo Desuja
(Member Since : 8/10/2004 10:56:17 PM)
|
 |
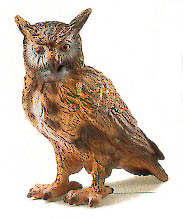
|
Job Description :
The King of Night....... Love to do programming specially night time.... |
View all (22) submissions by this author
(Birth Date : ) |
|
|