|
|
|
Get a valid handle of AVI file using AVIFileOpen api
The next step is to be able to open the AVI file that the user pass the AVI path a get a valid file handle hFile which can be passed to other AVIFile functions as necessary. We use the AVIFileOpen function to do this. Here is the VB declaration for AVIFileOpen. |
The AVIFileOpen function accepts the same OF_XXXX flags as the OpenFile API function. The hFile variable will contain the file interface pointer to the AVI file specified in the szFile varable. If the call is successful, it will return AVIERR_OK ( which is 0)
Parameters:
ppfile [in] Address to contain the new file interface pointer.
szFile [in] Null-terminated string containing the name of the file to open.
mode [in] Access mode to use when opening the file. The default access mode is OF_READ. The following access modes can be specified with AVIFileOpen:
- OF_CREATE : Creates a new file. If the file already exists, it is truncated to zero length.
- OF_SHARE_DENY_NONE : Opens the file nonexclusively. Other processes can open the file with read or write access. AVIFileOpen fails if another process has opened the file in compatibility mode.
- OF_SHARE_DENY_READ : Opens the file nonexclusively. Other processes can open the file with write access. AVIFileOpen fails if another process has opened the file in compatibility mode or has read access to it.
- OF_SHARE_DENY_WRITE : Opens the file nonexclusively. Other processes can open the file with read access. AVIFileOpen fails if another process has opened the file in compatibility mode or has write access to it.
- OF_SHARE_EXCLUSIVE : Opens the file and denies other processes any access to it. AVIFileOpen fails if any other process has opened the file.
- OF_READ : Opens the file for reading.
- OF_READWRITE : Opens the file for reading and writing.
- OF_WRITE : Opens the file for writing.
pclsidHandler [in] Address of a class identifier of the standard or custom handler you want to use. If the value is NULL, the system chooses a handler from the registry based on the file extension or the RIFF type specified in the file.
Read AVI file information using AVIFileInfo api
After getting a valid handle to AVI file you can read AVI file information by calling AVIFileInfo. Here is the VB declaration for AVIFileInfo. |
This function takes 3 parameters
Parameters :
pfile [in] Handle of an open AVI file.
pfi [in, out] Address of the structure used to return file information. Typically, this parameter points to an AVIFILEINFO (we have renamed to AVI_FILE_INFO to avoid confusion with API and Structure with same name) structure.
lSize [in] Size, in bytes, of the structure.
So our actual call may be like below |
Print AVI file information which we retrived in AVI_FILE_INFO structure
Here is a simple routine to dump the information stored in AVI_FILE_INFO structure. |
Click here to copy the following block | Public Sub PrintAVIFileInfo(afi As AVI_FILE_INFO, Optional txtDebug As TextBox) Dim txt As String txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & "**** AVI_FILE_INFO (START) *******" txt = txt & vbCrLf & "**********************************" With afi txt = txt & vbCrLf & "dwMaxBytesPerSecond = " & .dwMaxBytesPerSecond txt = txt & vbCrLf & "dwFlags = " & .dwFlags txt = txt & vbCrLf & "dwCaps = " & .dwCaps txt = txt & vbCrLf & "dwStreams = " & .dwStreams txt = txt & vbCrLf & "dwSuggestedBufferSize = " & .dwSuggestedBufferSize txt = txt & vbCrLf & "dwWidth = " & .dwWidth txt = txt & vbCrLf & "dwHeight = " & .dwHeight txt = txt & vbCrLf & "dwScale = " & .dwScale txt = txt & vbCrLf & "dwRate = " & .dwRate txt = txt & vbCrLf & "dwLength = " & .dwLength txt = txt & vbCrLf & "dwEditCount = " & .dwEditCount txt = txt & vbCrLf & "szFileType = " & .szFileType End With txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & "**** AVI_FILE_INFO (END) *********" txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & "" If IsMissing(txtDebug) Then Debug.Print txt Else txtDebug = txt End If End Sub |
Release AVI file and AVIFile Lib using AVIFileRelease and AVIFileExit api
And at last once we done we can call cleanup routines |
The AVIFileRelease function decrements the reference count of an AVI file interface handle and closes the file if the count reaches zero.
AVIFileRelease takes only one parameter pfile which is our AVI file handle hFile.
The AVIFileExit function exits the AVIFile library and decrements the reference count for the library. It takes no argument.
And here is the full copy/paste implementation of our example. To run this example
- Create a standard exe project - Place one command button - Place 2 text box. Set MultiLine=True and Scrollbar=both for text2 - Run the project - Specify valid AVI path and click on Command button
Place following code in Form1
Form1.frm |
Click here to copy the following block | Private Sub Command1_Click() Private Sub Command1_Click() On Error GoTo errHandler
Dim hFile As Long, AviInfo As AVI_FILE_INFO
Call AVIFileInit If AVIFileOpen(hFile, Text1, OF_SHARE_DENY_WRITE, ByVal 0&) = 0 Then If AVIFileInfo(hFile, AviInfo, Len(AviInfo)) = 0 Then Call PrintAVIFileInfo(AviInfo, Text2) Else MsgBox "Error while retrieving AVI information... " & GetAPIErrorDesc(Err.LastDllError) End If Else MsgBox "Error while opening the AVI file... " & GetAPIErrorDesc(Err.LastDllError) End If
errHandler: If hFile <> 0 Then Call AVIFileRelease(hFile)
Call AVIFileExit End If End Sub
Private Sub Form_Load()
If Len(App.Path) > 3 Then Text1 = App.Path & "\" & "test.avi" Else Text1 = App.Path & "test.avi" End If End Sub |
Place following code in the module
Module1.bas |
Click here to copy the following block | Option Explicit
Global Const FORMAT_MESSAGE_ALLOCATE_BUFFER = &H100 Global Const FORMAT_MESSAGE_FROM_SYSTEM = &H1000 Global Const LANG_NEUTRAL = &H0 Global Const SUBLANG_DEFAULT = &H1
Public Declare Function GetLastError Lib "kernel32" () As Long Public Declare Sub SetLastError Lib "kernel32" (ByVal dwErrCode As Long) Public Declare Function FormatMessage Lib "kernel32" Alias "FormatMessageA" (ByVal dwFlags As Long, lpSource As Any, ByVal dwMessageId As Long, ByVal dwLanguageId As Long, ByVal lpBuffer As String, ByVal nSize As Long, Arguments As Long) As Long
Public Declare Function AVIFileInfo Lib "avifil32.dll" _ (ByVal pfile As Long, _ pfi As AVI_FILE_INFO, _ ByVal lSize As Long) As Long
Public Declare Sub AVIFileInit Lib "avifil32.dll" ()
Public Declare Function AVIFileOpen Lib "avifil32.dll" _ (ByRef ppfile As Long, _ ByVal szFile As String, _ ByVal uMode As Long, _ ByVal pclsidHandler As Long) As Long
Public Declare Function AVIFileRelease Lib "avifil32.dll" _ (ByVal pfile As Long) As Long
Public Declare Sub AVIFileExit Lib "avifil32.dll" ()
Public Type AVI_FILE_INFO dwMaxBytesPerSecond As Long dwFlags As Long dwCaps As Long dwStreams As Long dwSuggestedBufferSize As Long dwWidth As Long dwHeight As Long dwScale As Long dwRate As Long dwLength As Long dwEditCount As Long szFileType As String * 64 End Type
Global Const OF_CREATE = &H1000 Global Const OF_READ = &H0 Global Const OF_READWRITE = &H2 Global Const OF_SHARE_DENY_WRITE = &H20 Global Const OF_WRITE = &H1
Public Sub PrintAVIFileInfo(afi As AVI_FILE_INFO, Optional txtDebug As TextBox) Dim txt As String
txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & "**** AVI_FILE_INFO (START) *******" txt = txt & vbCrLf & "**********************************" With afi txt = txt & vbCrLf & "dwMaxBytesPerSecond = " & .dwMaxBytesPerSecond txt = txt & vbCrLf & "dwFlags = " & .dwFlags txt = txt & vbCrLf & "dwCaps = " & .dwCaps txt = txt & vbCrLf & "dwStreams = " & .dwStreams txt = txt & vbCrLf & "dwSuggestedBufferSize = " & .dwSuggestedBufferSize txt = txt & vbCrLf & "dwWidth = " & .dwWidth txt = txt & vbCrLf & "dwHeight = " & .dwHeight txt = txt & vbCrLf & "dwScale = " & .dwScale txt = txt & vbCrLf & "dwRate = " & .dwRate txt = txt & vbCrLf & "dwLength = " & .dwLength txt = txt & vbCrLf & "dwEditCount = " & .dwEditCount txt = txt & vbCrLf & "szFileType = " & .szFileType End With txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & "**** AVI_FILE_INFO (END) *********" txt = txt & vbCrLf & "**********************************" txt = txt & vbCrLf & ""
If IsMissing(txtDebug) Then Debug.Print txt Else txtDebug = txt End If End Sub
Public Function GetAPIErrorDesc(ErrorCode As Long) As String Dim Buffer As String Buffer = Space(200) FormatMessage FORMAT_MESSAGE_FROM_SYSTEM, ByVal 0&, ErrorCode, LANG_NEUTRAL, Buffer, 200, ByVal 0& GetAPIErrorDesc = Buffer End Function |
|
|
|
Submitted By :
Jojo Desuja
(Member Since : 8/10/2004 10:56:17 PM)
|
 |
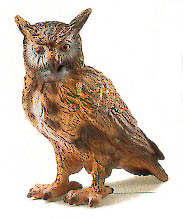
|
Job Description :
The King of Night....... Love to do programming specially night time.... |
View all (22) submissions by this author
(Birth Date : ) |
|
|