|
|
|
This sample code will show you how to control a shelled application using API.
I have used several tricks in this sample code. Once you know these tricks, you can also use them in your programs. This code will open Notepad at a specified position with specified window size. You will also learn how to get a Window handle from ProcessId.
Step-By-Step Example
- Create a standard exe project - Add two command button controls on the form1 - Place the following code in form1 code window |
Click here to copy the following block | Option Explicit
Private Type STARTUPINFO cb As Long lpReserved As String lpDesktop As String lpTitle As String dwX As Long dwY As Long dwXSize As Long dwYSize As Long dwXCountChars As Long dwYCountChars As Long dwFillAttribute As Long dwFlags As Long wShowWindow As Integer cbReserved2 As Integer lpReserved2 As Long hStdInput As Long hStdOutput As Long hStdError As Long End Type
Private Type PROCESS_INFORMATION hProcess As Long hThread As Long dwProcessID As Long dwThreadID As Long End Type
Private Declare Function WaitForSingleObject Lib "kernel32.dll" (ByVal hHandle As Long, ByVal dwMilliseconds As Long) As Long Private Declare Function CloseHandle Lib "kernel32.dll" (ByVal hObject As Long) As Long Private Declare Function CreateProcessA Lib "kernel32.dll" (ByVal lpApplicationName As Long, ByVal lpCommandLine As _ String, ByVal lpProcessAttributes As Long, ByVal _ lpThreadAttributes As Long, ByVal bInheritHandles As Long, _ ByVal dwCreationFlags As Long, ByVal lpEnvironment As Long, _ ByVal lpCurrentDirectory As Long, lpStartupInfo As _ STARTUPINFO, lpProcessInformation As PROCESS_INFORMATION) As Long
Private Const NORMAL_PRIORITY_CLASS = &H20& Private Const INFINITE = -1&
Public taskID As Long
Private Const GW_HWNDFIRST = 0 Private Const GW_HWNDNEXT = 2 Private Const GW_CHILD = 5
Private Declare Function GetWindow Lib "user32" (ByVal hWnd As Long, ByVal wCmd As Long) As Long Private Declare Function GetDesktopWindow Lib "user32" () As Long Private Declare Function GetWindowThreadProcessId Lib "user32" (ByVal hWnd As Long, lpdwProcessId As Long) As Long
Private Declare Function SetWindowPos Lib "user32" _ (ByVal hWnd As Long, ByVal hWndInsertAfter As Long, _ ByVal X As Long, ByVal Y As Long, _ ByVal cx As Long, ByVal cy As Long, ByVal wFlags As Long) As Long Private Declare Sub Sleep Lib "kernel32" (ByVal dwMilliseconds As Long)
Private Const HWND_TOP = 0 Private Const HWND_BOTTOM = 1 Private Const HWND_TOPMOST = -1 Private Const HWND_NOTOPMOST = -2
Private Const SWP_NOSIZE = &H1 Private Const SWP_NOMOVE = &H2 Private Const SWP_NOZORDER = &H4 Private Const SWP_NOREDRAW = &H8 Private Const SWP_NOACTIVATE = &H10 Private Const SWP_FRAMECHANGED = &H20 Private Const SWP_SHOWWINDOW = &H40 Private Const SWP_HIDEWINDOW = &H80 Private Const SWP_NOCOPYBITS = &H100 Private Const SWP_NOOWNERZORDER = &H200
Public Function hWndFromProcID(ProcID As Long) As Long Dim lhTmp As Long Dim lRetVal As Long Dim lCurrentProcID As Long On Error GoTo errhWndFromProcID lhTmp = GetDesktopWindow() lhTmp = GetWindow(lhTmp, GW_CHILD) Do While lhTmp <> 0 lRetVal = GetWindowThreadProcessId(lhTmp, lCurrentProcID) If lCurrentProcID = ProcID Then hWndFromProcID = lhTmp Exit Do End If lhTmp = GetWindow(lhTmp, GW_HWNDNEXT) Loop Exit Function errhWndFromProcID: hWndFromProcID = 0 End Function
Public Function ExecuteTask(Path As String, Optional Parameters As String = "", _ Optional XPos As Long = 0, _ Optional YPos As Long = 0, _ Optional lWidth As Long = -1, _ Optional lHeight As Long = -1) As Long
Dim proc As PROCESS_INFORMATION Dim startup As STARTUPINFO Dim ret As Long Dim cmdline As String
cmdline = Path If Trim(Parameters) <> "" Then cmdline = cmdline & " """ & Parameters & """" End If startup.cb = Len(startup) ret = CreateProcessA(0&, cmdline, 0&, 0&, 1&, NORMAL_PRIORITY_CLASS, 0&, 0&, startup, proc)
If lWidth <> -1 Or lHeight <> -1 Then Dim lHandle As Long Sleep 100
lHandle = hWndFromProcID(proc.dwProcessID) SetWindowPos lHandle, 0, XPos, YPos, lWidth, lHeight, 0 End If
ExecuteTask = proc.hProcess End Function
Public Function IsTaskRunning(taskID As Long) As Boolean Dim ret As Long
If taskID = 0 Then Exit Function ret = WaitForSingleObject(taskID, 0) IsTaskRunning = (ret <> 0) If Not IsTaskRunning Then ret = CloseHandle(taskID) taskID = 0 End If End Function
Private Sub Command1_Click() taskID = ExecuteTask("Notepad", , 10, 10, 600, 300) End Sub
Private Sub Command2_Click() If IsTaskRunning(taskID) Then MsgBox "Task running", vbInformation Else MsgBox "Task not running", vbExclamation End If End Sub
Private Sub Form_Load() Command1.Caption = "Launch New Task" Command2.Caption = "Check Task Status" End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
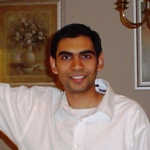
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|