|
|
|
CountSort is yet another sort algorithm, which can be applied only under very particular conditions but that, when such conditions are met, turns to be the fastest of the lot. Count Sort can be used when the key is of Integer type, or when it is of Long type but the range of values is not too large.
The idea underlying the algorithm is that each key data can indirectly work an initial index for itself. The routine parses the original array determining its minimum and maximum value, then builds a temporary array of Long with one element for each possible value of the key. The routine parses the original array again, this time counting the occurrences of each distinct value. After this step, it is very easy to evaluate where each value has to go in the definitive, sorted array, and this can be accomplished in the third step. Here is the complete routine:
The most interesting detail on this sorting algorithm is that its total time is proportional to the number of items to be sorted, therefore is even more convenient than Quick Sort, whose processing time grows proportionally to N * Log2(N). When sorting 10,000 items, Count Sort is 2.5 faster than Quick Sort, and is about five times faster when sorting one hundred thousand values. |
Click here to copy the following block | Sub NdxCountSortI(arr() As Integer, ndx() As Long, Optional ByVal numEls As _ Variant) Dim index As Long Dim inverseOrder As Boolean Dim value As Integer Dim minValue As Integer Dim maxValue As Integer Dim saveCount As Integer Dim newIndex As Long
If IsMissing(numEls) Then numEls = UBound(arr)
minValue = arr(LBound(arr)) maxValue = minValue For index = LBound(arr) To numEls value = arr(index) If value < minValue Then minValue = value ElseIf value > maxValue Then maxValue = value End If Next
ReDim Count(minValue To maxValue) As Long For index = LBound(arr) To numEls value = arr(index) Count(value) = Count(value) + 1 Next
newIndex = LBound(ndx) For index = minValue To maxValue saveCount = Count(index) Count(index) = newIndex newIndex = newIndex + saveCount Next
For index = LBound(arr) To numEls newIndex = Count(arr(index)) ndx(newIndex) = index Count(arr(index)) = newIndex + 1 Next End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
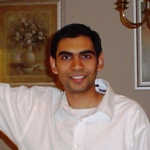
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|