|
|
|
Click here to copy the following block | Private Type BITMAP bmType As Long bmWidth As Long bmHeight As Long bmWidthBytes As Long bmPlanes As Integer bmBitsPixel As Integer bmBits As Long End Type
Private Type SafeArray2 cDims As Integer fFeatures As Integer cbElements As Long cLocks As Long pvData As Long cElements1 As Long lLbound1 As Long cElements2 As Long lLbound2 As Long End Type
Private Declare Sub CopyMemory Lib "kernel32" Alias "RtlMoveMemory" (pDest As _ Any, pSrc As Any, ByVal ByteLen As Long) Private Declare Function GetObjectAPI Lib "gdi32" Alias "GetObjectA" (ByVal _ hObject As Long, ByVal nCount As Long, lpObject As Any) As Long
Private Sub RotatePicture2(sourcePB As PictureBox, destPB As PictureBox, _ xc As Long, yc As Long, degrees As Single)
Const PI As Long = 31416 Const HALFPI As Long = 15707 Const DOUBLEPI As Long = 62831 Const SQRTABLE_MAX As Long = 40000 Static sinTable() As Single Static atnTable() As Long Static sqrTable() As Single Static initialized As Boolean Dim pict1() As Byte Dim pict2() As Byte Dim p1 As SafeArray2, p2 As SafeArray2 Dim bmp1 As BITMAP, bmp2 As BITMAP Dim radians As Long Dim angle As Long Dim angle0 As Long Dim distance As Single Dim distanceSquared As Long Dim deltaX As Long, deltaY As Long Dim deltaXSquared As Single, deltaX10000 As Long Dim x As Long, y As Long Dim dx As Long, dy As Long Dim x0 As Long, y0 As Long Dim xx As Long, yy As Long Dim xStart As Long, xEnd As Long Dim yStart As Long, yEnd As Long Dim bmWidth1 As Long Dim bmHeight1 As Long If Not initialized Then initialized = True Dim i As Long ReDim sinTable(0 To 62831 + 15709) As Single For i = 0 To UBound(sinTable) sinTable(i) = Sin(i / 10000) Next ReDim atnTable(0 To 10000) As Long For i = LBound(atnTable) To UBound(atnTable) atnTable(i) = Atn(i / 10000) * 10000# Next ReDim sqrTable(SQRTABLE_MAX) As Single For i = 0 To SQRTABLE_MAX sqrTable(i) = Sqr(i) Next End If GetObjectAPI sourcePB.Picture, Len(bmp1), bmp1 GetObjectAPI destPB.Picture, Len(bmp2), bmp2
If bmp1.bmPlanes <> 1 Or bmp1.bmBitsPixel <> 8 Or bmp2.bmPlanes <> 1 Or _ bmp2.bmBitsPixel <> 8 Then MsgBox "This routine supports 256-color bitmaps only", vbCritical Exit Sub End If With p1 .cbElements = 1 .cDims = 2 .lLbound1 = 0 .cElements1 = bmp1.bmHeight .lLbound2 = 0 .cElements2 = bmp1.bmWidthBytes .pvData = bmp1.bmBits End With CopyMemory ByVal VarPtrArray(pict1), VarPtr(p1), 4 With p2 .cbElements = 1 .cDims = 2 .lLbound1 = 0 .cElements1 = bmp2.bmHeight .lLbound2 = 0 .cElements2 = bmp2.bmWidthBytes .pvData = bmp2.bmBits End With CopyMemory ByVal VarPtrArray(pict2), VarPtr(p2), 4 radians = degrees / (180 / 3.14159) * 10000& - 628310000 If xc < bmp2.bmWidth \ 2 Then xStart = xc xEnd = bmp2.bmWidth - 1 Else xStart = 0 xEnd = xc End If
If yc < bmp2.bmHeight \ 2 Then yStart = yc yEnd = bmp2.bmWidth - 1 Else yStart = 0 yEnd = yc End If If xEnd - xStart > yEnd - yStart Then If yStart = 0 Then yStart = yEnd - (xEnd - xStart) Else yEnd = yStart + (xEnd - xStart) End If Else If xStart = 0 Then xStart = xEnd - (yEnd - yStart) Else xEnd = xStart + (yEnd - yStart) End If End If
bmWidth1 = bmp1.bmWidth bmHeight1 = bmp1.bmHeight For x = xStart To xEnd deltaX = x - xc deltaXSquared = deltaX * deltaX deltaX10000 = deltaX * 10000
For y = yStart To yEnd deltaY = y - yc If deltaX > 0 Then If deltaY >= 0 Then If deltaY < deltaX Then angle = atnTable((deltaY * 10000) \ deltaX) Else angle = HALFPI - atnTable(deltaX10000 \ deltaY) End If Else If -deltaY < deltaX Then angle = -atnTable((deltaY * -10000) \ deltaX) Else angle = -HALFPI + atnTable(-deltaX10000 \ deltaY) End If End If ElseIf deltaX < 0 Then If deltaY > 0 Then If deltaY < -deltaX Then angle = PI - atnTable((deltaY * -10000) \ deltaX) Else angle = HALFPI + atnTable(-deltaX10000 \ deltaY) End If Else If deltaY > deltaX Then angle = PI + atnTable((deltaY * 10000) \ deltaX) Else angle = -HALFPI - atnTable(deltaX10000 \ deltaY) End If End If Else If deltaY >= 0 Then angle = HALFPI Else angle = -HALFPI End If End If distanceSquared = deltaXSquared + deltaY * deltaY If distanceSquared <= SQRTABLE_MAX Then distance = sqrTable(distanceSquared) Else distance = Sqr(distanceSquared) End If angle0 = (angle - radians) Mod DOUBLEPI dx = distance * sinTable(angle0 + HALFPI) dy = distance * sinTable(angle0) If x >= 0 And x < bmWidth1 And y >= 0 And y < bmHeight1 Then x0 = xc + dx y0 = yc + dy If x0 >= 0 And x0 < bmWidth1 And y0 >= 0 And y0 < bmHeight1 Then pict2(x, y) = pict1(x0, y0) Else pict2(x, y) = 0 End If xx = xc - deltaX yy = yc - deltaY If xx >= 0 And xx < bmWidth1 And yy >= 0 And yy < bmHeight1 Then x0 = xc - dx y0 = yc - dy If x0 >= 0 And x0 < bmWidth1 And y0 >= 0 And y0 < bmHeight1 _ Then pict2(xx, yy) = pict1(x0, y0) Else pict2(xx, yy) = 0 End If End If End If xx = xc + deltaY yy = yc - deltaX If xx >= 0 And xx < bmWidth1 And yy >= 0 And yy < bmHeight1 Then x0 = xc + dy y0 = yc - dx If x0 >= 0 And x0 < bmWidth1 And y0 >= 0 And y0 < bmHeight1 Then pict2(xx, yy) = pict1(x0, y0) Else pict2(xx, yy) = 0 End If End If
xx = xc - deltaY yy = yc + deltaX If xx >= 0 And xx < bmWidth1 And yy >= 0 And yy < bmHeight1 Then x0 = xc - dy y0 = yc + dx If x0 >= 0 And x0 < bmWidth1 And y0 >= 0 And y0 < bmHeight1 Then pict2(xx, yy) = pict1(x0, y0) Else pict2(xx, yy) = 0 End If End If Next Next CopyMemory ByVal VarPtrArray(pict1), 0&, 4 CopyMemory ByVal VarPtrArray(pict2), 0&, 4 destPB.Refresh End Sub
Private Function VarPtrArray(arr As Variant) As Long CopyMemory VarPtrArray, ByVal VarPtr(arr) + 8, 4 End Function |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
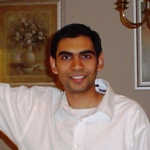
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|