|
|
|
This article explains how to use a Visual Basic application to access the registry on a remote computer in conjunction with the Windows application programming interface (API).
Requirements
You need the following hardware and software to perform the procedures in this article: A Microsoft Windows XP, Microsoft Windows 2000, or Microsoft Windows NT 4.0-based computer Visual Basic 6.0 NOTE: If the remote registry is on a system that is running Windows NT 4.0, Windows 2000, or Windows XP, you must run the code from an account that has permission to read that registry.
You also need the following skills:
Visual Basic 6.0 programming experience Familiarity with the Windows registry |
Click here to copy the following block | Option Explicit Private hRemoteReg As Long
Private Const HKEY_CLASSES_ROOT = &H80000000 Private Const HKEY_CURRENT_USER = &H80000001 Private Const HKEY_LOCAL_MACHINE = &H80000002 Private Const HKEY_USERS = &H80000003
Private Const KEY_QUERY_VALUE = &H1 Private Const KEY_SET_VALUE = &H2 Private Const KEY_ALL_ACCESS = &H3F
Private Const REG_SZ As Long = 1 Private Const ERROR_SUCCESS = 0& Private Declare Function RegConnectRegistry Lib "advapi32.dll" _ Alias "RegConnectRegistryA" _ (ByVal lpMachineName As String, _ ByVal hKey As Long, _ phkResult As Long) As Long
Private Declare Function RegCloseKey Lib "advapi32.dll" _ (ByVal hKey As Long) As Long
Private Declare Function RegOpenKeyEx Lib "advapi32.dll" _ Alias "RegOpenKeyExA" _ (ByVal hKey As Long, _ ByVal lpSubKey As String, _ ByVal ulOptions As Long, _ ByVal samDesired As Long, _ phkResult As Long) As Long Private Declare Function RegQueryValueExString Lib "advapi32.dll" _ Alias "RegQueryValueExA" _ (ByVal hKey As Long, _ ByVal lpValueName As String, _ ByVal lpReserved As Long, _ lpType As Long, _ ByVal lpData As String, _ lpcbData As Long) As Long
Private Sub Command1_Click() Dim lRetVal As Long Dim hKey As Long Dim sValue As String
lRetVal = RegOpenKeyEx(hRemoteReg, _ "HARDWARE\DESCRIPTION\System", 0, KEY_QUERY_VALUE, hKey)
If lRetVal <> ERROR_SUCCESS Then MsgBox "Cannot open key" Else sValue = String(255, " ") lRetVal = RegQueryValueExString(hKey, _ "SystemBIOSVersion", 0&, REG_SZ, sValue, 255)
If lRetVal <> ERROR_SUCCESS Then MsgBox "Cannot query value" Else MsgBox sValue End If lRetVal = RegCloseKey(hKey) If lRetVal <> ERROR_SUCCESS Then MsgBox "Cannot close key" End If End If End Sub
Private Sub Form_Load() Dim lRet As Long lRet = RegConnectRegistry("\\TST", _ HKEY_LOCAL_MACHINE, _ hRemoteReg) If (lRet = ERROR_SUCCESS) Then MsgBox "Successfully connected to remote registry" Else MsgBox "Error:" & Err.LastDllError Unload Me Exit Sub End If End Sub Private Sub Form_Unload(Cancel As Integer) Dim lRet As Long If hRemoteReg <> 0 Then lRet = RegCloseKey(hRemoteReg) End If End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
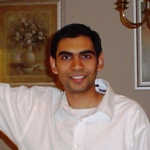
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|