|
|
|
As you probably know, the "&" operator is rather slow, especially with long strings. When you have to repeatedly append chucks of characters to the same variable, you can speed up your code using a simple trick based on the Mid$ command. The idea is that you pre-allocate a buffer long enough to accomodate for the result of your operation. Here's an example of this technique.
Suppose you want to create a string given by appending the first 10000 integers, e.g. "1 2 3 4 5 6 7 ... 9999 10000". This is the easiest way to do so: |
The problem with the standard approach is that the res variable is reallocated 10000 times. This is a better way to reach the same result: |
Click here to copy the following block | Dim res As String Dim i As Long Dim index As Long
res = Space(90000)
index = 1
For i = 1 to 10000 substr = Str(i) length = Len(substr) Mid$(res, index, length) = substr index = index + length Next
res = Left$(res, index - 1) |
The standard code takes 2.2 seconds on my 333 MHz system computer, while the smart way takes only 0.08 seconds, so it's 25+ times faster.
|
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
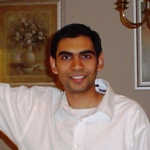
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|