|
|
|
The following example uses some of the methods discussed in the Basic String Operations topics to construct a class that performs string manipulations in a manner that might be found in a real-world application. The MailToData class stores the name and address of an individual in separate properties and provides a way to combine the City, State, and Zip fields into a single string for display to the user. Furthermore, the class allows the user to enter the city, state, and ZIP Code information as a single string; the application automatically parses the single string and enters the proper information into the corresponding property.
For simplicity, this example uses a console application with a command-line interface. |
Click here to copy the following block | Option Explicit Option Strict
Imports System Imports System.IO
Class MainClass Public Shared Sub Main() Dim MyData As New MailToData() Console.Write("Enter Your Name:") MyData.Name = Console.ReadLine() Console.Write("Enter Your Address:") MyData.Address = Console.ReadLine() Console.Write("Enter Your City, State, and ZIP Code separated by spaces:") MyData.CityStateZip = Console.ReadLine() Console.WriteLine("Name: {0}", MyData.Name) Console.WriteLine("Address: {0}", MyData.Address) Console.WriteLine("City: {0}", MyData.City) Console.WriteLine("State: {0}", MyData.State) Console.WriteLine("ZIP Code: {0}", MyData.Zip) Console.WriteLine("The following address will be used:") Console.WriteLine(MyData.Address) Console.WriteLine(MyData.CityStateZip) End Sub End Class
Public Class MailToData Private strName As String = " " Private strAddress As String = " " Private strCityStateZip As String = " " Private strCity As String = " " Private strState As String = " " Private strZip As String = " " Public Sub New()
End Sub Public Property Name() As String Get Return strName End Get Set strName = value End Set End Property Public Property Address() As String Get Return strAddress End Get Set strAddress = value End Set End Property Public Property CityStateZip() As String Get Return ReturnCityStateZip() End Get Set strCityStateZip = value ParseCityStateZip() End Set End Property Public Property City() As String Get Return strCity End Get Set strCity = value End Set End Property Public Property State() As String Get Return strState End Get Set strState = value End Set End Property Public Property Zip() As String Get Return strZip End Get Set strZip = value End Set End Property Private Sub ParseCityStateZip() Dim CityIndex As Integer Dim StateIndex As Integer
Try CityIndex = strCityStateZip.IndexOf(" ") Dim CityArray(CityIndex) As Char strCityStateZip.CopyTo(0, CityArray, 0, CityIndex) StateIndex = strCityStateZip.LastIndexOf(" ") Dim StateArray(StateIndex - CityIndex) As Char strCityStateZip.CopyTo(CityIndex, StateArray, 0, StateIndex - CityIndex) Dim ZipArray(strCityStateZip.Length - StateIndex) As Char strCityStateZip.CopyTo(StateIndex, ZipArray, 0, strCityStateZip.Length - StateIndex) strCity = New String(CityArray) strCity = strCity.Trim(New Char() {" "c, ","c, ";"c, "-"c, ":"c}) strState = New String(StateArray) strState = strState.Trim(New Char() {" "c, ","c, ";"c, "-"c, ":"c})
strZip = New String(ZipArray) strZip = strZip.Trim(New Char() {" "c, ","c, ";"c, "-"c, ":"c})
Catch OverflowException As Exception Console.WriteLine(ControlChars.Lf + ControlChars.Lf + "You must enter spaces between elements." + ControlChars.Lf + ControlChars.Lf) End Try
End Sub Private Function ReturnCityStateZip() As String strState = strState.ToUpper() Dim MyCityStateZip As String = String.Concat(strCity, ", ", strState, " ", strZip) Return MyCityStateZip End Function End Class |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
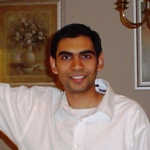
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|