|
|
|
The following routines convert a numeric value into a string that represents the number, and vice versa. They are useful for reading data written by QuickBasic programs, because the QuickBasic language functions that did the conversions were not ported to Visual Basic.
Points to note: 1. These routines are a .NET update to the VB5/VB6 Code Bank article MK? And CV? - Convert numbers to strings and back. 2. The data type differences between VB.NET and previous versions of VB and QB, have forced a change to the parameters of the function calls and API calls - Long (QB/VB) = Integer (.NET), Integer (QB/VB) = Short (.NET), etc. 3. The class calls "unmanaged" code via the API calls. Therefore there may be permission problems when trying to install and run Internet applications that use this code in a .NET environment. 4. Because .NET doesn't support the Currency data type and the Variant data type, the VB5/VB6 routines in the original Code Bank article that handled those data types were not ported. |
Click here to copy the following block | Option Explicit On Imports System.Runtime.InteropServices
Public Class QB2VB
Private Declare Sub CopyMemoryMKD Lib "Kernel32" Alias "RtlMoveMemory" _ (ByVal hDest As String, ByRef hSource As Double, _ ByVal iBytes As Integer) Private Declare Sub CopyMemoryCVD Lib "Kernel32" Alias "RtlMoveMemory" _ (ByRef hDest As Double, ByVal hSource As String, _ ByVal iBytes As Integer) Private Declare Sub CopyMemoryMKS Lib "Kernel32" Alias "RtlMoveMemory" _ (ByVal hDest As String, ByRef hSource As Single, _ ByVal iBytes As Integer) Private Declare Sub CopyMemoryCVS Lib "Kernel32" Alias "RtlMoveMemory" _ (ByRef hDest As Single, ByVal hSource As String, _ ByVal iBytes As Integer) Private Declare Sub CopyMemoryMKL Lib "Kernel32" Alias "RtlMoveMemory" _ (ByVal hDest As String, ByRef hSource As Integer, _ ByVal iBytes As Integer) Private Declare Sub CopyMemoryCVL Lib "Kernel32" Alias "RtlMoveMemory" _ (ByRef hDest As Integer, ByVal hSource As String, _ ByVal iBytes As Integer) Private Declare Sub CopyMemoryMKI Lib "Kernel32" Alias "RtlMoveMemory" _ (ByVal hDest As String, ByRef hSource As Short, ByVal iBytes As Integer) Private Declare Sub CopyMemoryCVI Lib "Kernel32" Alias "RtlMoveMemory" _ (ByRef hDest As Short, ByVal hSource As String, ByVal iBytes As Integer) Private Declare Sub CopyMemoryMKDt Lib "Kernel32" Alias "RtlMoveMemory" _ (ByVal hDest As String, ByRef hSource As Double, _ ByVal iBytes As Integer) Private Declare Sub CopyMemoryCVDt Lib "Kernel32" Alias "RtlMoveMemory" _ (ByRef hDest As Double, ByVal hSource As String, _ ByVal iBytes As Integer)
Shared Function MKD(ByRef Value As Double) As String Dim sTemp As String = Space(8) CopyMemoryMKD(sTemp, Value, 8I) Return sTemp End Function
Shared Function CVD(ByRef Argument As String) As Double Dim dTemp As Double = 0.0R If Len(Argument) <> 8 Then Return Double.NaN End If CopyMemoryCVD(dTemp, Argument, 8I) Return dTemp End Function
Shared Function MKS(ByRef Value As Single) As String Dim sTemp As String = Space(4) CopyMemoryMKS(sTemp, Value, 4I) Return sTemp End Function
Shared Function CVS(ByRef Argument As String) As Single Dim sTemp As Single = 0.0F If Len(Argument) <> 4 Then Return Single.NaN End If CopyMemoryCVS(sTemp, Argument, 4I) Return sTemp End Function
Shared Function MKL(ByRef Value As Integer) As String Dim sTemp As String = Space(4) CopyMemoryMKL(sTemp, Value, 4I) Return sTemp End Function
Shared Function CVL(ByRef Argument As String) As Long Dim lTemp As Integer = 0I If Len(Argument) <> 4 Then Return Long.MinValue End If CopyMemoryCVL(lTemp, Argument, 4I) Return CLng(lTemp) End Function
Shared Function MKI(ByRef Value As Short) As String Dim sTemp As String = Space(2) CopyMemoryMKI(sTemp, Value, 2I) Return sTemp End Function
Shared Function CVI(ByRef Argument As String) As Integer Dim iTemp As Short = 0S If Len(Argument) <> 2 Then Return Integer.MinValue End If CopyMemoryCVI(iTemp, Argument, 2I) Return CInt(iTemp) End Function
Shared Function MKDt(ByRef Value As Double) As String Dim sTemp As String = Space(8) CopyMemoryMKDt(sTemp, Value, 8I) Return sTemp End Function
Shared Function CVDt(ByRef Argument As String) As DateTime Dim dTemp As Double = 0.0R If Len(Argument) <> 8 Then Return DateTime.MinValue End If CopyMemoryCVDt(dTemp, Argument, 8I) Return Date.FromOADate(dTemp) End Function
End Class |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
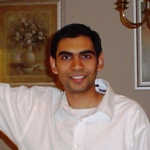
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|