|
|
|
If your ASP pages display strings stored in database fields, you should always process the strings with the Server.HTMLEncode method, otherwise the string won't be displayed correctly in the user's browser if it contains characters that have a special meaning to HTML, such as the quote ("), the less-than (<) and greater-than (>) symbols, the ampersand symbol (&), and any character whose ANSI code is larger than 127.
Here's an example of how you should use the Server.HTMLEncode method: |
Click here to copy the following block | Dim rs Set rs = Server.CreateObject("ADODB.Recordset") rs.Open "products", "DSN=mydb"
' display the product name on the first line Response.Write rs("product_name") & "<BR>" ' the Description field might contain special chars Response.Write Server.HTMLEncode(rs("product_description")) |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
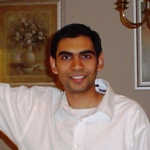
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|