|
|
|
In this article we will discuss about Object Oriented Features available in VB.net
Let’s first understand the basic terminology used in Object Oriented Programming.
Classes, Objects, Members and Abstraction
An object in programming is a construct that represents something from the real life. In the real world, objects are cars, trucks, computers, and so on. Objects of a kind have some common properties and uses. But at the same time each object has some unique properties.
In object oriented programming you can create classes and implement public/private methods (or member functions) and properties, these methods and properties also known as members of a class. So we can consider a class as a template and object as an instance of a class.
The ability of programmatic objects to represent real-world objects is called abstraction (e.g. Customer object is abstract representation of real world customer). We'll see soon how abstraction is achieved.
Encapsulation
Encapsulation is a concept of defining public interface of a class and hiding internal representation and complexities. You can expose public interface of your class to programmers but you can hide all internal complexities, which is known as encapsulation. For example you can create a class CSorting and you can expose a method Sort() but you hide your sorting algorithm from the users by encapsulating it in the class. Abstraction and encapsulation go together.
Inheritance
Inheritance allows you to create several classes that are distinct but share a common functionality. Specialized classes, called derived classes, inherit from a common class called the base class. For example you can have a base class called CEmployee with some common members (e.g. FirstName, LastName, Age) And then you can create derived class called CManager which inherits all members from its base class CEmployee and implements some new members (e.g. DepartmentName).
Polymorphism
Generally, ability to appear in many forms is known as Polymorphism. But this definition is not enough when we are talking in terms of programming. Polymorphism is the ability of different classes to provide different implementations of the same public interfaces (i.e. methods, properties). This is possible in many ways in VB.net.
Inheritance Polymorphism : This type of polymorphism allows you to override base class methods to write your own version of base class method. For example you can derive CTruck, CCar and CBike classes from CVehicle. You can override Drive method of CVehicle in derived class if you want different implementation other than Drive method of base class CVehicle. We will see detail example when we talk about Inheritance and Overriding in this article.
Interface Polymorphism : In this type of polymorphism classes which need to have a common set of functionality implement a common interface. Once class supports the interface then it must implement its own version of all members (i.e. Properties, Methods) defined by the interface. In brief, Interface is a standard which must be supported by all classes which the interface otherwise compiler will generate compile time error. Java programmers should find this familiar!
Another type of polymorphism available in VB.net is overloaded function. Overloaded function allows you to create multiple definitions of the same function with different input parameters.
Overloading and Overriding
Many programmers new to object oriented programming often get confused with these two terms- Overloading and Overriding.
Overloading
Basically overloading allows you to create multiple definitions of the same function each differing only in the input parameters. Process of defining multiple signatures of the same function is known as overloading. When you define more than one method with exact same name but with different signature then it’s known as Overloaded method. For example you can define 2 versions of DrawRectangle method in the same class as below |
Click here to copy the following block | Class CGraphics Sub DrawRectangle(ByVal x1 As Integer, ByVal y1 As Integer, ByVal x2 As Integer, ByVal y2 As Integer) End Sub
Sub DrawRectangle(ByVal p1 As Point, ByVal p2 As Point) End Sub End Class |
If you look at the above code we have two methods with the same name DrawRectangle but both accept different parameters hence becomes Overloaded method.
Another example is if you have a function called Abs() which returns absolute value of an integer. You can define more functions having the same function name but accepting Float and Double values.
In VB overloading can also be implemented using Optional Parameters or ParmArray.
Overriding
Process of redefining the base class function in the derived class is known as Overriding. You can only override base class properties/methods if it’s marked with Overridable keyword in the base class. We will explore this concept later in this article. Summary Now that we have learnt about some of the basic object oriented terms, we'll see how to implement this in VB.net in the next part of this tutorial. |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
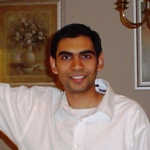
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|