|
|
|
Click here to copy the following block | Public Enum eDateLocale edlArabic = &H401 edlDanish = &H406 edlGerman = &H407 edlSwissGerman = &H807 edlAmerican = &H409 edlBritish = &H809 edlAustralian = &HC09 edlSpanish = &H40A edlFinnish = &H40B edlFrench = &H40C edlFrenchCanadian = &HC0C edlHebrew = &H40D edlItalian = &H410 edlDutch = &H413 edlDutchPreferred = &H13 edlDutchBelgian = &H813 edlNorskBokmal = &H414 edlNorskNynorsk = &H814 edlPortBrazil = &H416 edlPortIberian = &H816 edlSwedish = &H41D edlCatalan = &H403 edlRussian = &H419 edlCzech = &H405 edlHungarian = &H40E edlPolish = &H415 edlJapanese = &H411 edlKorean = &H412 edlTaiwan = &H404 edlChina = &H804 edlTurkish = &H41F edlGreek = &H408 edlBasque = &H42D edlSlovenian = &H424 edlMalaysian = &H43E edlAfrikaans = &H436 edlBulgarian = &H402 edlCroatian = &H41A edlEstonian = &H425 edlLatvian = &H426 edlLithuanian = &H427 edlMacedonian = &H42F edlRomanian = &H418 edlSerbianCyrillic = &HC1A edlSerbianLatin = &H81A edlByelorussian = &H423 edlSlovak = &H41B edlUkrainian = &H422 edlIcelandic = &H40F edlVietnamese = &H42A edlThai = &H41E End Enum
Private Const LOCALE_SDAYNAME1 = &H2A Private Const LOCALE_SDAYNAME2 = &H2B Private Const LOCALE_SDAYNAME3 = &H2C Private Const LOCALE_SDAYNAME4 = &H2D Private Const LOCALE_SDAYNAME5 = &H2E Private Const LOCALE_SDAYNAME6 = &H2F Private Const LOCALE_SDAYNAME7 = &H30 Private Const LOCALE_SMONTHNAME1 = &H38 Private Const LOCALE_SMONTHNAME2 = &H39 Private Const LOCALE_SMONTHNAME3 = &H3A Private Const LOCALE_SMONTHNAME4 = &H3B Private Const LOCALE_SMONTHNAME5 = &H3C Private Const LOCALE_SMONTHNAME6 = &H3D Private Const LOCALE_SMONTHNAME7 = &H3E Private Const LOCALE_SMONTHNAME8 = &H3F Private Const LOCALE_SMONTHNAME9 = &H40 Private Const LOCALE_SMONTHNAME10 = &H41 Private Const LOCALE_SMONTHNAME11 = &H42 Private Const LOCALE_SMONTHNAME12 = &H43
Private Declare Function IsValidLocale Lib "kernel32" (ByVal Locale As Long, _ ByVal dwFlags As Long) As Boolean Private Declare Function GetLocaleInfo Lib "kernel32" Alias "GetLocaleInfoA" _ (ByVal Locale As Long, ByVal LCType As Long, ByVal lpLCData As String, _ ByVal cchData As Long) As Long Private Declare Function GetUserDefLCID Lib "kernel32" Alias _ "GetUserDefaultLCID" () As Long
Function FormatInternationalDate(sDate As String, Optional sRequiredFormat As _ String = "YYYY/MM/DD", Optional sDelimiter As Variant = " ") As String On Error GoTo ERROR_FormatInternationalDate Dim sTmp As String Dim oSplit() As String Dim sDay As String Dim sMonth As String Dim sYear As String Dim colLanguage As New Collection Dim oLanguage As Variant Dim nLanguage As eDateLocale sDay = vbNullString sMonth = vbNullString sYear = vbNullString sTmp = Trim$(sDate) Do While InStr(sTmp, sDelimiter & sDelimiter) > 0 sTmp = Replace(sTmp, sDelimiter & sDelimiter, sDelimiter, , , _ vbTextCompare) Loop oSplit = Split(sTmp, sDelimiter) If UBound(oSplit) = 2 Then sDay = oSplit(0) sMonth = oSplit(1) sYear = oSplit(2) Else sMonth = sDate End If colLanguage.Add "L" & edlFrench colLanguage.Add "L" & edlFrenchCanadian colLanguage.Add "L" & edlGerman colLanguage.Add "L" & edlSpanish colLanguage.Add "L" & edlItalian colLanguage.Add "L" & edlDutch colLanguage.Add "L" & edlSwedish colLanguage.Add "L" & edlDanish colLanguage.Add "L" & edlArabic colLanguage.Add "L" & edlSwissGerman colLanguage.Add "L" & edlAmerican colLanguage.Add "L" & edlBritish colLanguage.Add "L" & edlAustralian colLanguage.Add "L" & edlFinnish colLanguage.Add "L" & edlHebrew colLanguage.Add "L" & edlDutchPreferred colLanguage.Add "L" & edlDutchBelgian colLanguage.Add "L" & edlNorskBokmal colLanguage.Add "L" & edlNorskNynorsk colLanguage.Add "L" & edlPortBrazil colLanguage.Add "L" & edlPortIberian colLanguage.Add "L" & edlCatalan colLanguage.Add "L" & edlRussian colLanguage.Add "L" & edlCzech colLanguage.Add "L" & edlHungarian colLanguage.Add "L" & edlPolish colLanguage.Add "L" & edlJapanese colLanguage.Add "L" & edlKorean colLanguage.Add "L" & edlTaiwan colLanguage.Add "L" & edlChina colLanguage.Add "L" & edlTurkish colLanguage.Add "L" & edlGreek colLanguage.Add "L" & edlBasque colLanguage.Add "L" & edlSlovenian colLanguage.Add "L" & edlMalaysian colLanguage.Add "L" & edlAfrikaans colLanguage.Add "L" & edlBulgarian colLanguage.Add "L" & edlCroatian colLanguage.Add "L" & edlEstonian colLanguage.Add "L" & edlLatvian colLanguage.Add "L" & edlLithuanian colLanguage.Add "L" & edlMacedonian colLanguage.Add "L" & edlRomanian colLanguage.Add "L" & edlSerbianCyrillic colLanguage.Add "L" & edlSerbianLatin colLanguage.Add "L" & edlByelorussian colLanguage.Add "L" & edlSlovak colLanguage.Add "L" & edlUkrainian colLanguage.Add "L" & edlIcelandic colLanguage.Add "L" & edlVietnamese colLanguage.Add "L" & edlThai
nLanguage = GetUserDefLCID() sMonth = ReplaceAllMonths(sMonth, nLanguage)
If IsNumeric(sMonth) = False Then For Each oLanguage In colLanguage nLanguage = Replace(oLanguage, "L", vbNullString) sMonth = ReplaceAllMonths(sMonth, nLanguage) If IsNumeric(sMonth) Then Exit For Next End If If IsNumeric(sDay) And IsNumeric(sMonth) And IsNumeric(sYear) Then FormatInternationalDate = Format(DateSerial(sYear, sMonth, sDay), _ sRequiredFormat) Else FormatInternationalDate = vbNullString End If
EXIT_FormatI |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
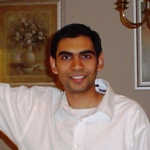
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|