|
|
|
The Process class provides all you need to create a command-line utility that lists all the processes running on the system and all the related information. This utility is therefore similar to the Windows Task Manager, except you can run it from the command prompt. All processes are sorted alphabetically.
The following routine uses the routines FormatValue and FormatMemorySize, that are available elsewhere in our Code Bank. You should compile it as a console application with the name "Processes." |
Click here to copy the following block | Imports System.Diagnostics
Module Module1 Sub Main() Dim procs() As Process = Process.GetProcesses Dim procNames(procs.Length - 1) As String Dim i As Integer For i = 0 To procNames.Length - 1 procNames(i) = GetProcessName(procs(i)) Next Array.Sort(procNames, procs)
Console.WriteLine("PROCESS NAME___________PID__STARTED_CPU " _ & "TIME___MEMORY_THREADS_HANDLES____") Console.WriteLine()
For i = 0 To procs.Length - 1 Dim sb As New System.Text.StringBuilder() Dim p As Process = procs(i)
Try sb.Append(FormatValue(procNames(i), 20, _ FormatColumnAlignment.Left)) sb.Append(" "c) sb.Append(FormatValue(p.Id, 5, FormatColumnAlignment.Right)) sb.Append(" "c) sb.Append(FormatValue(p.StartTime.TimeOfDay, 8, _ FormatColumnAlignment.Left)) sb.Append(" "c)
sb.Append(FormatValue(p.TotalProcessorTime, 8, _ FormatColumnAlignment.Left)) sb.Append(" "c) sb.Append(FormatValue(FormatMemorySize(p.WorkingSet, _ FormatMemorySizeUnits.Kilobytes), 8, _ FormatColumnAlignment.Right)) sb.Append(" "c) sb.Append(FormatValue(p.Threads.Count, 7, _ FormatColumnAlignment.Right)) sb.Append(" "c) sb.Append(FormatValue(p.HandleCount, 7, _ FormatColumnAlignment.Right)) sb.Append(" "c)
Console.WriteLine(sb.ToString) Catch ex As Exception End Try Next End Sub
Function GetProcessName(ByVal p As Process) As String Try Dim procName As String = p.ProcessName If Not p.MainModule Is Nothing Then procName = p.MainModule.ModuleName End If Return procName Catch ex As Exception End Try End Function End Module |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
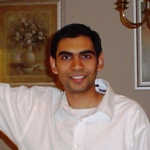
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|