|
|
|
The Microsoft .NET Framework provides a robust system of primitive types to store and represent data in your application. Data primitives represent integer numbers, floating-point numbers, Boolean values, characters, and strings.
In VB.net you can declare variable using Dim statement as shown below. You can also initialize value during declaration or you can assign value later. |
In our previous article "Language and Syntax changes in VB.Net for VB6 Programmers" I explained Variable Types little bit but in this article will cover in detail.
Under .net all data types are inherited from System.Object class so all data types in VB.net share common functionality exposed by System.Object class. In .Net there are 2 types of variables.
- Value type (Structures and Primitive types) - Reference type (Classes)
Value Type (Structure and Premitive types) vs Reference Type
All inbuilt data types (i.e. Integer, Boolean, Double… etc.) and Structures are Value types.
The main difference between Value type and reference type is
Value objects get allocated on the stack in general Reference type get allocated on the Heap in general
When any variable assigned to value type then it gets copy of data instead of reference of the variable.
Example of Value type |
The above example will print "AAA" because s2 contains copy of s1 so after assignment if you change s1 its not going to affect s2.
Lets look at the example of Reference Type.
Example of Reference Type |
So here in the above example output will be "BBB" because sb2 is reference type data type and it’s basically pointing to sb1 so whenever you make any changes to sb2 or sb1 it will change data of sb1.
Now let’s understand what is stack and heap
The Stack:
Stack is a special type of memory area and it is used to store data of fixed length, such as integer (every int consists of 4 bytes in .net). Every Program in execution has its own separate stack that no other program can look into. It is very fast to allocate Push on the stack and deAllocate Pop values off the stack, so speed is important here.
The Heap: In C and C++, the heap tended to be used partly to store data of variable length, such as strings, and partly to store data that needs to have a lifetime longer than the method in which it is first defined. So objects can persist and can be passed via a reference to other functions in the program. In .net it is called the Managed Heap. The managed heap operates internally in a more efficient way. Based on the type of object the compiler makes the choice where the object will be allocated on the stack or the heap.
Structures are put on the stack Arrays are put on the Heap
Etc...
Lets look at different datatypes available in VB.net |
Visual Basic type |
.NET Runtime type |
structure Storage size |
Value range |
Boolean |
System.Boolean |
4 bytes |
True or False |
Byte |
System.Byte |
1 byte |
0 to 255 (unsigned) |
Char |
System.Char |
2 bytes |
0 to 65535 (unsigned) |
Date |
System.DateTime |
8 bytes |
January 1, 1 CE to December 31, 9999 |
Decimal |
System.Decimal |
12 bytes |
+/-79,228,162,514,264,337,593,543,950,335 with
no decimal point; +/-7.9228162514264337593543950335 with 28
places to the right of the decimal; smallest non-zero number is
+/-0.0000000000000000000000000001 |
Double |
System.Double |
8 bytes |
-1.79769313486231E308 to -4.94065645841247E-324
for negative values; 4.94065645841247E-324 to
1.79769313486232E308 for positive values
|
Integer |
System.Int32 |
4 bytes |
-2,147,483,648 to 2,147,483,647 |
Long |
System.Int64 |
8 bytes |
-9,223,372,036,854,775,808 to
9,223,372,036,854,775,807
|
Object |
System.Object (class) |
4 bytes |
Any type can be stored in a variable of type
Object
|
Short |
System.Int16 |
2 bytes |
-32,768 to 32,767 |
Single |
System.Single |
4 bytes |
-3.402823E38 to -1.401298E-45 for negative
values; 1.401298E-45 to 3.402823E38 for positive values |
String |
System.String (class) |
10 bytes + (2 * string length) |
0 to approximately two billion Unicode
characters |
User-Defined Type (structure) |
(inherits from System.ValueType )
|
Sum of the sizes of its members |
Each member of the structure has a range
determined by its data type and independent of the ranges of the
other members
|
.NET Framework types not available in VB.NET: SByte ,
UInt16 , UInt32 , UInt64 . |
|
Now lets look at each datatype with example
Integer type
In VB6 we had Integer datatype which was 16 bit but in VB7 (VB.net) same Integer datatype is 32 bit (4 Bytes). On 32-bit processor 32 bit datatype is processed faster than 16-bit or 64-bit datatype so in VB.net Integer is the most fastest datatype for 32 bit processor.
In Vb.net you can declare Integer using any of the following statement. |
Lets look at the difference in previous version and current version integer datatypes. |
The following table shows correspondences between previous integer types and Visual Basic.NET 7.0 types.
Integer Size |
VB6 Type & Character |
VB.net Type & Character |
.NET Framework and Runtime Type |
16 bits, signed |
Integer (% ) |
Short (none) |
System.Int16 |
32 bits, signed |
Long (& ) |
Integer (% ) |
System.Int32 |
64 bits, signed |
<Not Available> |
Long (& ) |
System.Int64 |
|
You can assign values to the Integer types using either decimal or hexadecimal notation. To use hexadecimal notation in an integer literal, it should be prefixed with &H for Visual Basic .NET |
Floating-Point Types
You can represent numbers with fraction using following datatypes provided by VB.net |
Visual Basic type |
.NET Runtime type |
structure Storage size |
Value range |
Single |
System.Single |
4 bytes |
-3.402823E38 to -1.401298E-45
for negative values; 1.401298E-45 to 3.402823E38 for positive values |
Decimal |
System.Decimal |
12 bytes |
+/-79,228,162,514,264,337,593,543,950,335 with no
decimal point; +/-7.9228162514264337593543950335 with 28 places to the
right of the decimal; smallest non-zero number is
+/-0.0000000000000000000000000001 |
Double |
System.Double |
8 bytes |
-1.79769313486231E308 to -4.94065645841247E-324 for
negative values; 4.94065645841247E-324 to 1.79769313486232E308 for
positive values
|
|
Boolean type
System.Boolean type can represent True or False. In VB.net False is 0 and True is -1.
Char and Byte Datatypes
Char is a new datatype in VB.net. Char datatype can store single unicode character so its actually 16 bit type. All unicode characters can be represented using 2 bytes. |
In the above example note the c after character literal
Another type is Byte which can store 0 to 255. |
Date or DateTime
DateTime and Date both are same types just 2 different names. In VB.net DateTime is very different than VB6 Date type. VB6 stores date as Double (8 Bytes) but now in .Net DateTime is defined as Structure which exposes several useful methods and properties. Lets look at a simple example which demonstrate few propeties of datetime type. |
Click here to copy the following block | Dim dt As DateTime = "12/2/2005"
Console.WriteLine(dt.UtcNow)
Console.WriteLine(dt.Second) Console.WriteLine(dt.Minute) Console.WriteLine(dt.Hour) Console.WriteLine(dt.Day) Console.WriteLine(dt.Month) Console.WriteLine(dt.Year) |
For detail description about DateTime type please check article "Working with DateTime and TimeSpan Object in VB.net"
String
VB.net has the same String datatype as VB6 but internals are changed. In VB.net any alteration to String causes New string to be created. So the following statement will create new string instead of changing the existing string. |
This is surely performance hit but still its transparent to the developers.
Another change from VB6 to VB.net is now no more fixed length string. So the following statement is invalid in VB.net |
For more information check the "String Manipulation" article
Here is list of some useful string methods. |
Some Useful Static (Shared) String Methods
Name |
Description |
String.Compare |
Compares two specified string objects |
String.Concat |
Returns a string that is the result of the concatenation
of two or more strings |
String.Format |
Returns a string that has been formatted according to a
specified format |
String.Join |
Returns a string that is the result of the concatenation
of a specified array of strings, with a specified separation
string between each member of the array |
Some Useful Instance String Methods
Name |
Description |
String.Insert |
Inserts a specified string into the current instance |
String.PadLeft,
String.PadRight |
Adds characters to the left and right of the string, respectively |
String.Remove |
Deletes a specified number of characters from the string, beginning
at a specified character |
String.Replace |
Replaces all occurrences of a specified character in the string with
another specified character |
String.Split |
Returns an array of substrings that are delimited by a specified character |
String.Substring |
Returns a substring from the specified instance |
String.ToCharArray |
Returns an array of the characters that make up the string |
String.ToLower,
String.ToUpper |
Returns the string converted to all lowercase or all uppercase, respectively |
String.TrimEnd,
String.TrimStart,
String.Trim |
Removes trailing, leading, or both characters from the string, respectively |
|
Object
The Object data type is nothing but just a 32-bit (4-byte) address that points to an object within your application or in some other application. When you declare a variable as Object, you can subsequently use it to refer to any object recognized by your application (i.e. Integer, String, Textbox, Datagrid, Form ...etc).
The Object type is the supertype of all types in the .NET Framework. Every type, whether value type or reference type, derives from System.Object that means object type can point to any datatype in VB.net as shown in the following example: |
Click here to copy the following block | Dim objControls(3) As Object Dim i As Integer
objControls(0) = Me.TextBox1 objControls(1) = Me.ComboBox1 objControls(2) = Me.Label1 objControls(3) = Me.CheckBox1
For i = 0 To UBound(objControls) If TypeOf objControls(i) Is CheckBox Then Console.WriteLine(CType(objControls(i), CheckBox).Text)
ElseIf TypeOf objControls(i) Is Label Then Console.WriteLine(CType(objControls(i), Label).Text)
ElseIf TypeOf objControls(i) Is TextBox Then Console.WriteLine(CType(objControls(i), TextBox).Text)
ElseIf TypeOf objControls(i) Is ComboBox Then Console.WriteLine(CType(objControls(i), ComboBox).Text)
End If Next |
So from above example you can see that Object datatype can store reference to anytype including VB.net primitive datatypes, custom classes and Structues. You can cast Object datatype to appropriate type using CType function as shown in the example. CType allows you to convert one datatype to another datatype.
Structure (User Defined Type)
The concept of UDT (User defined type) allows you to create your own complex datatype. In VB.net you can create UDT using "Structure" keyword. |
UDT is more powerful than "Type" in VB6. In UDT you can define methods and properties as shown below. |
Click here to copy the following block |
Public Structure SudentInfo Private m_ID As Integer
Public Age As Integer Public FirstName As String Public Lastname As String Public Property ID() As Integer Get Return m_ID End Get Set(ByVal Value As Integer) If Value <= 0 Then m_ID = 0 Else m_ID = Value End If End Set End Property Public Function FullName() As String Return FirstName + " " + Lastname End Function End Structure |
You must be thinking that whats the difference between "Class" and "Structure". Visual Basic.NET unifies the syntax for structures and classes, with the result that both entities support most of the same features. However, there are also important differences between structures and classes.
Structures and classes are similar in the following respects:
- Both are container types, meaning that they contain other types as members.
- Both have members, which can include constructors, methods, properties, fields, constants, enumerations, events, and event handlers.
- Members of both can have individualized accessibilities. For example, one member can be declared Public and another Private.
- Both can implement interfaces.
- Both can have shared constructors, with or without parameters.
- Both can expose a default property, provided that property takes at least one argument.
- Both can declare and raise events, and both can declare delegates.
Structures and classes differ in the following particulars:
- Structures are value types; classes are reference types.
- Structures use stack allocation; classes use heap allocation.
- All structure members are Public by default; class variables and constants are Private by default, while other class members are Public by default. This behavior for class members provides compatibility with the Visual Basic 6.0 system of defaults.
- A structure must have at least one nonshared variable or event member; a class can be completely empty.
- Structure members cannot be declared as Protected; class members can.
- A structure procedure can handle events only if it is a Shared Sub procedure, and only by means of the AddHandler statement; any class procedure can handle events, using either the Handles keyword or the AddHandler statement.
- Structure variable declarations cannot specify initializers, the New keyword, or initial sizes for arrays; class variable declarations can.
- Structures implicitly inherit from the ValueType class and cannot inherit from any other type; classes can inherit from any class or classes other than ValueType.
- Structures are not inheritable; classes are.
- Structures are never terminated, so the common language runtime (CLR) never calls the Finalize method on any structure; classes are terminated by the garbage collector, which calls Finalize on a class when it detects there are no active references remaining.
- A structure does not require a constructor; a class does.
- Structures can have nonshared constructors only if they take parameters; classes can have them with or without parameters.
|
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
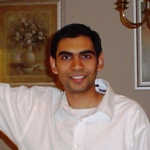
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|