|
|
|
Reflection makes it easy to invoke a method (or assign a field or a property) by its name. For example, suppose you have the following classes: |
Click here to copy the following block | Class Person Event PropertyChanged(ByVal propertyName As String, ByVal newValue As Object)
Dim m_Name As String
Property Name() As String Get Return m_Name End Get Set(ByVal Value As String) If Value <> m_Name Then m_Name = Value RaiseEvent PropertyChanged("Name", m_Name) End If End Set End Property End Class |
Here's how you can assign a Person's Name and a Car's Color through reflection: |
However, setting up an event handler for an event of which you know just the name isn't as immediate. These are the steps you need to follow: 1. Create an event handler that reflects the event syntax 2. Get the EventInfo object corresponding to the event in question 3. Create a delegate to local event handler by means of the Delegate.CreateDelegate method 4. call the EventInfo.AddEventHandler method to establish the connection
Remember to remove the event handler when you don't need to receive events any longer. Here's a complete example that uses the Person class, but it can be easily adapted to other classes: |
Click here to copy the following block | Module MainModule Sub Main Dim pe As New Person() Dim peEv As EventInfo = pe.GetType.GetEvent("PropertyChanged") Dim peDel As [Delegate] = [Delegate].CreateDelegate(peEv.EventHandlerType, _ Me, "PropertyChanged") peEv.AddEventHandler(pe, peDel)
pe.Name = "John"
peEv.RemoveEventHandler(pe, peDel) End Sub
Private Sub PropertyChanged(ByVal propertyName As String, _ ByVal newValue As Object) Console.WriteLine("Property {0} has changed. The new value is {1}", _ propertyName, newValue) End Sub End Module |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
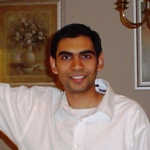
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|